In Salesforce Visualforce Page, we can dynamically make a field read-only using disabled attribute.
Sample Code:
Visualforce page:
<apex:page controller="sample">
<apex:form >
<apex:pageBlock>
<apex:pageBlockSection columns="2">
<apex:pageblockSectionItem>
<apex:outputLabel value="State"/>
</apex:pageblockSectionItem>
<apex:pageblockSectionItem>
<apex:selectList size="1" value="{!state}">
<apex:selectOptions value="{!states}"/>
<apex:actionSupport event="onchange" reRender="a"/>
</apex:selectList>
</apex:pageblockSectionItem>
<apex:pageblockSectionItem>
<apex:outputLabel value="City"/>
</apex:pageblockSectionItem>
<apex:pageblockSectionItem>
<apex:selectList size="1" value="{!city}" id="a" disabled="{!bool}">
<apex:selectOptions value="{!cities}"/>
</apex:selectList>
</apex:pageblockSectionItem>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:form>
</apex:page>
Apex Class:
public class sample {
public String state {
get; set;
}
public String city {
get; set;
}
public Boolean bool {
get; set;
}
public sample() {
bool = true;
}
public List < SelectOption > getStates() {
List < SelectOption > options = new List < SelectOption >();
options.add(
new SelectOption(
'None','--- None ---'
)
);
options.add(
new SelectOption(
'TN','Tamil Nadu'
)
);
options.add(
new SelectOption(
'KL','Kerala'
)
);
return options;
}
public List<SelectOption> getCities() {
List < SelectOption > options = new List < SelectOption >();
if ( state == 'TN' ) {
options.add(
new SelectOption(
'CHE', 'Chennai'
)
);
options.add(
new SelectOption(
'CBE', 'Coimbatore'
)
);
bool = false;
} else if ( state == 'KL' ) {
options.add(
new SelectOption(
'COA', 'Coachin'
)
);
options.add(
new SelectOption(
'MVL', 'Mavelikara'
)
);
bool = false;
}
else {
options.add(
new SelectOption(
'None', '--- None ---'
)
);
bool = true;
}
return options;
}
}
Output:
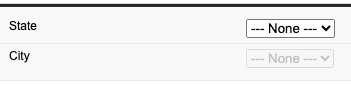
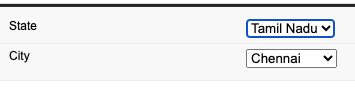