To find current or latest API version of Salesforce organization, please use the following steps:
1. Go to Develop –> API.
2. Click “Generate Partner WSDL”.
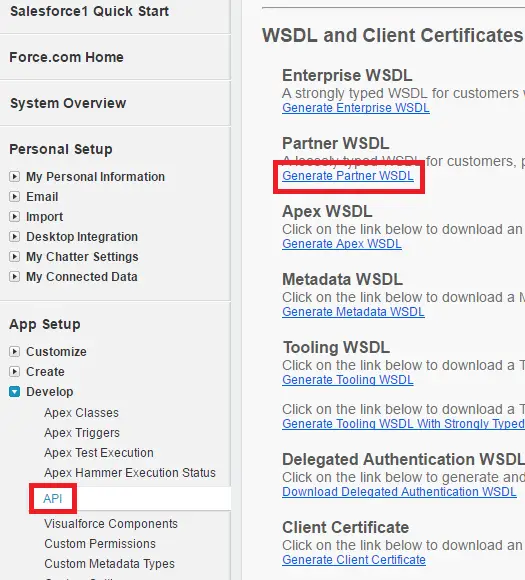
3. First line will show the version.
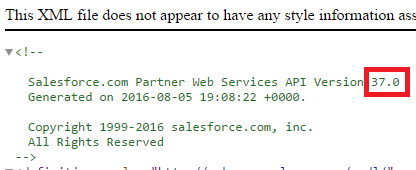
Use the following sample apex code if you want to use apex program to find the latest or current Salesforce API version.
Sample Apex Code:
// Wrapper class for parsing the Response from Salesforce API
public class Wrapper {
public String label;
public String url;
public String version;
}
// Making REST API Salesforce
Http h = new Http();
HttpRequest req = new HttpRequest();
req.setEndpoint(
Url.getOrgDomainUrl().toExternalForm() +
'/services/data'
);
req.setMethod( 'GET' );
req.setHeader(
'Authorization',
'Bearer ' + UserInfo.getSessionId()
);
HttpResponse res = h.send( req );
System.debug( 'Limits are ' + res.getBody() );
List < Wrapper > resultList =
( List < Wrapper > )JSON.deserialize( res.getBody(), List < Wrapper >.class );
for ( Wrapper objWrap : resultList ) {
System.debug( 'Label: ' + objWrap.label );
System.debug( 'URL: ' + objWrap.url );
System.debug( 'version: ' + objWrap.version );
}
System.debug(
'Latest Version is ' +
resultList.get( resultList.size() - 1 ).version
);
Reference Article:
https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/dome_versions.htm