Sample Code:
Component:
<aura:component implements="force:appHostable"
controller="OpportunitySearchController">
<aura:attribute name="oppty"
type="Opportunity"
default="{ 'sobjectType': 'Opportunity'}"/>
<aura:attribute name="columns" type="List"/>
<aura:attribute name="opportunityList" type="Opportunity[]"/>
<aura:attribute name="PaginationList" type="Opportunity[]"/>
<aura:attribute name="startPage" type="Integer" />
<aura:attribute name="endPage" type="Integer"/>
<aura:attribute name="totalRecords" type="Integer"/>
<aura:attribute name="pageSize" type="Integer" default="3"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}"/>
<div class="slds-box slds-theme_default">
<div class="slds-grid slds-grid_vertical">
<div class="slds-col">
Close Date<force:inputField value="{!v.oppty.CloseDate}"/>
</div>
<div class="slds-col">
Amount<force:inputField value="{!v.oppty.Amount}"/>
</div>
<div class="slds-col">
<lightning:button label="Search" onclick="{!c.searchOppty}"/>
</div>
</div>
</div>
<aura:if isTrue="true">
<div class="slds-grid slds-grid_vertical">
<lightning:datatable data="{!v.PaginationList}"
columns="{!v.columns}"
keyField="id"
hideCheckboxColumn="true"/>
<lightning:buttonGroup>
<lightning:button label="Previous"
disabled="{!v.startPage == 0}"
onclick="{!c.previous}"
variant="brand"
iconName='utility:back'/>
<lightning:button label="Next"
disabled="{!v.endPage + 1 >= v.totalRecords}"
onclick="{!c.next}"
variant="brand"
iconName='utility:forward'/>
</lightning:buttonGroup>
</div>
</aura:if>
</aura:component>
Controller:
({
doInit: function (component, event, helper) {
component.set('v.columns', [
{label: 'Name', fieldName: 'Name', type: 'text'},
{label: 'Closed Date', fieldName: 'CloseDate', type: 'date'},
{label: 'Stage', fieldName: 'StageName', type: 'text'}
]);
},
searchOppty : function(component) {
var action = component.get('c.searchOpportunities');
action.setParams({
clsDate : new Date(component.get("v.oppty.CloseDate")).toJSON(),
amt : component.get("v.oppty.Amount")
});
action.setCallback(this, function(response) {
var state = response.getState();
if ( state === 'SUCCESS' && component.isValid() ) {
var pageSize = component.get("v.pageSize");
component.set('v.opportunityList', response.getReturnValue());
component.set("v.totalRecords", component.get("v.opportunityList").length);
component.set("v.startPage", 0);
component.set("v.endPage", pageSize - 1);
var PagList = [];
for ( var i=0; i< pageSize; i++ ) {
if ( component.get("v.opportunityList").length> i )
PagList.push(response.getReturnValue()[i]);
}
component.set('v.PaginationList', PagList);
} else {
alert('ERROR');
}
});
$A.enqueueAction(action);
},
next: function (component, event, helper) {
var sObjectList = component.get("v.opportunityList");
var end = component.get("v.endPage");
var start = component.get("v.startPage");
var pageSize = component.get("v.pageSize");
var PagList = [];
var counter = 0;
for ( var i = end + 1; i < end + pageSize + 1; i++ ) {
if ( sObjectList.length > i ) {
PagList.push(sObjectList[i]);
}
counter ++ ;
}
start = start + counter;
end = end + counter;
component.set("v.startPage", start);
component.set("v.endPage", end);
component.set('v.PaginationList', PagList);
},
previous: function (component, event, helper) {
var sObjectList = component.get("v.opportunityList");
var end = component.get("v.endPage");
var start = component.get("v.startPage");
var pageSize = component.get("v.pageSize");
var PagList = [];
var counter = 0;
for ( var i= start-pageSize; i < start ; i++ ) {
if ( i > -1 ) {
PagList.push(sObjectList[i]);
counter ++;
} else {
start++;
}
}
start = start - counter;
end = end - counter;
component.set("v.startPage", start);
component.set("v.endPage", end);
component.set('v.PaginationList', PagList);
}
})
Apex Class:
public class OpportunitySearchController {
@auraEnabled
public static List < Opportunity > searchOpportunities(String clsDate, Double amt) {
system.debug(clsDate + ' - ' + amt);
Date closeDate = Date.valueOf(clsDate);
return [ SELECT Id, Name, CloseDate, StageName FROM Opportunity WHERE CloseDate =: closeDate AND Amount >: amt LIMIT 100 ];
}
}
Output:
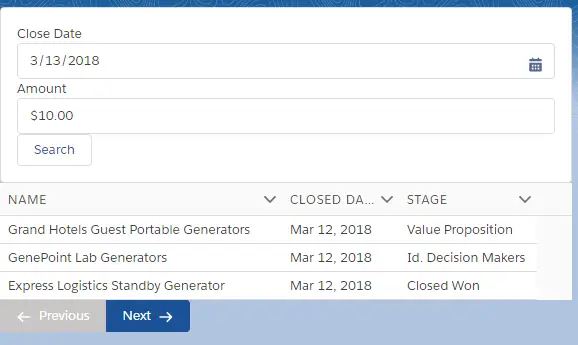