Error Handling in Lightning Component’s JavaScript Controller is important. The try statement in JavaScript helps us to test a block of code for errors. The catch statement in JavaScript helps us to handle the error. Errors from client side JavaScript can be due to error in the code, bad input from the user, etc.
Challenge:
When the user uses Lightning Component and raises an issue, we won’t have any clue what happened in the JavaScript Controller since it is a client-side code. In order to have a clue or an idea what happened in the JavaScript controller, use try and catch block to find the exceptions in the JavaScript controller and store the exceptions in a custom object. Show a user-friendly message on the screen for the user with the reference number of the error log record created for an exception so that they are not feeling lost.
Solution:
High Level Solution Design:
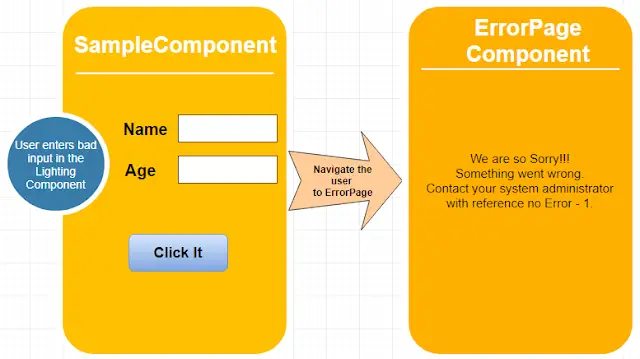
1. Create a Custom Object – Lightning_Error_Log__c to store the exceptions from the Lightning Component’s JavaScript Controller exception block.
Lighting Error Log Object Definition:
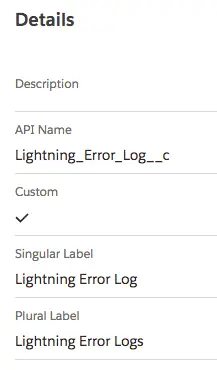
2. Create Error_Message__c custom field in Lightning_Error_Log__c custom object.
Lighting Error Log Object Fields:
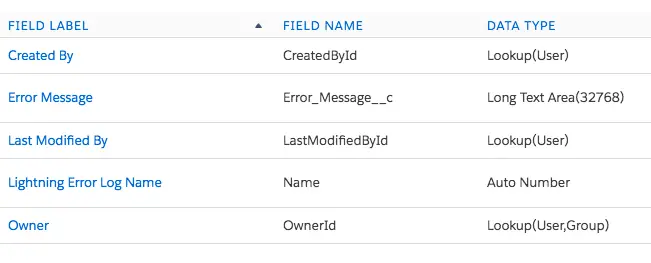
3. Create LightningErrorHandler Apex Class.
LightningErrorHandler Apex Class:
public class LightningErrorHandler {
@auraEnabled
public static Lightning_Error_Log__c createErroroLog(String errMsg, String errNam) {
Lightning_Error_Log__c objErrorLog = new Lightning_Error_Log__c();
objErrorLog.Error_Message__c = 'Error Message is ' + errMsg + ' and Error Name is ' + errNam;
insert objErrorLog;
return [ SELECT Name, Error_Message__c FROM Lightning_Error_Log__c WHERE Id =: objErrorLog.Id ];
}
}
4. Create ErrorPage Lightning Component.
ErrorPage.cmp
<aura:component controller="LightningErrorHandler">
<aura:attribute name="errorMsg" type="String"/>
<aura:attribute name="errorName" type="String"/>
<aura:attribute name="errorLog" type="Lightning_Error_Log__c"/>
<aura:handler name="init" value="{!this}" action="{!c.createLog}"/>
<center>
<div class="slds-box slds-theme_default">
We are so Sorry!!! <br/>
Something went wrong.<br/>
Contact your system administrator with reference no {!v.errorLog.Name}.
</div>
</center>
</aura:component>
ErrorPageController.js:
({
createLog : function(component, event, helper) {
var action = component.get("c.createErroroLog");
action.setParams({
errMsg : component.get("v.errorMsg"),
errNam : component.get("v.errorName")
});
action.setCallback(this, function(response){
var state = response.getState();
if (state === "SUCCESS") {
component.set("v.errorLog", response.getReturnValue());
}
});
$A.enqueueAction(action);
}
})
5. Create SampleComponent Lightning Component to demo the ErrorPage Lightning Component.
SampleComponent.cmp:
<aura:component implements="force:appHostable" >
<aura:attribute name="errMsg" type="String"/>
<aura:attribute name="errNam" type="String"/>
<div class="slds-box slds-theme_default">
<lightning:button variant="brand" label="Click It" onclick="{!c.sample}"/>
</div>
</aura:component>
SampleComponentController.js:
({
sample : function(component, event, helper) {
try {
var j = 1 / abc;
}
catch(e) {
component.set("v.errMsg", e.message);
component.set("v.errNam", e.name);
helper.callErrorLog(component);
}
}
})
SampleComponentHelper.js
({
callErrorLog : function(component) {
var evt = $A.get("e.force:navigateToComponent");
evt.setParams({
componentDef : "c:ErrorPage",
componentAttributes: {
errorMsg : component.get("v.errMsg"),
errorName : component.get("v.errNam")
}
});
evt.fire();
}
})
6. Create a Lighting Component tab.
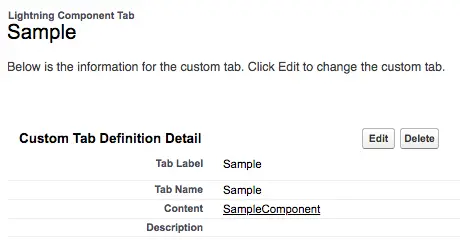
Output:
1. Open the Lightning Component tab created in step 6. Click “Click It” button from the SampleComponent Lightning Component.
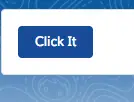
2. You will see ErrorPage Lightning Component with the sorry note and error log record reference number.
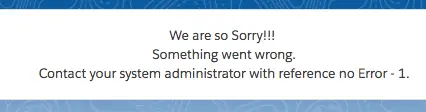
3. The created error log record from the init event of ErrorPage Lightning Component will be like below.
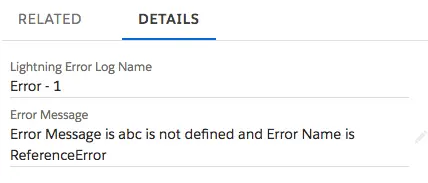
Using this approach, you will be able to track the exceptions thrown by JavaScript during Lightning Component development. I recommend reading more about try and catch block in JavaScript and add all additional information from the exception to the error log record.