Sample Code:
AccountListController.apex:
public class AccountListController {
@AuraEnabled
public static List < Account > fetchAccts() {
return [ SELECT Id, Name, Industry, Type FROM Account LIMIT 10 ];
}
}
AccountList.cmp:
<aura:component implements="force:appHostable"
controller="AccountListController">
<aura:attribute type="Account[]" name="acctList"/>
<aura:attribute name="mycolumns" type="List"/>
<aura:handler name="init" value="{!this}" action="{!c.fetchAccounts}"/>
<lightning:datatable data="{! v.acctList }"
columns="{! v.mycolumns }"
keyField="Id"
hideCheckboxColumn="true"
onrowaction="{!c.viewRecord}"/>
</aura:component>
AccountListController.js:
({
fetchAccounts : function(component, event, helper) {
component.set('v.mycolumns', [
{label: 'Account Name', fieldName: 'Name', type: 'text'},
{label: 'Industry', fieldName: 'Industry', type: 'text'},
{label: 'Type', fieldName: 'Type', type: 'Text'},
{type: "button", typeAttributes: {
label: 'View',
name: 'View',
title: 'View',
disabled: false,
value: 'view',
iconPosition: 'left'
}},
{type: "button", typeAttributes: {
label: 'Edit',
name: 'Edit',
title: 'Edit',
disabled: false,
value: 'edit',
iconPosition: 'left'
}}
]);
var action = component.get("c.fetchAccts");
action.setParams({
});
action.setCallback(this, function(response){
var state = response.getState();
if (state === "SUCCESS") {
component.set("v.acctList", response.getReturnValue());
}
});
$A.enqueueAction(action);
},
viewRecord : function(component, event, helper) {
var recId = event.getParam('row').Id;
var actionName = event.getParam('action').name;
if ( actionName == 'Edit' ) {
alert('Edit');
var editRecordEvent = $A.get("e.force:editRecord");
editRecordEvent.setParams({
"recordId": recId
});
editRecordEvent.fire();
} else if ( actionName == 'View') {
alert('view');
var viewRecordEvent = $A.get("e.force:navigateToURL");
viewRecordEvent.setParams({
"url": "/" + recId
});
viewRecordEvent.fire();
}
}
})
Output:
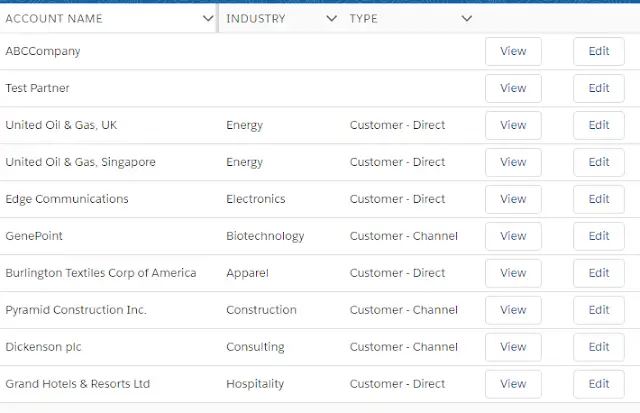
If you click View button, it will take you to the Account detail page.
If you click Edit button, it will popup Account Edit form.