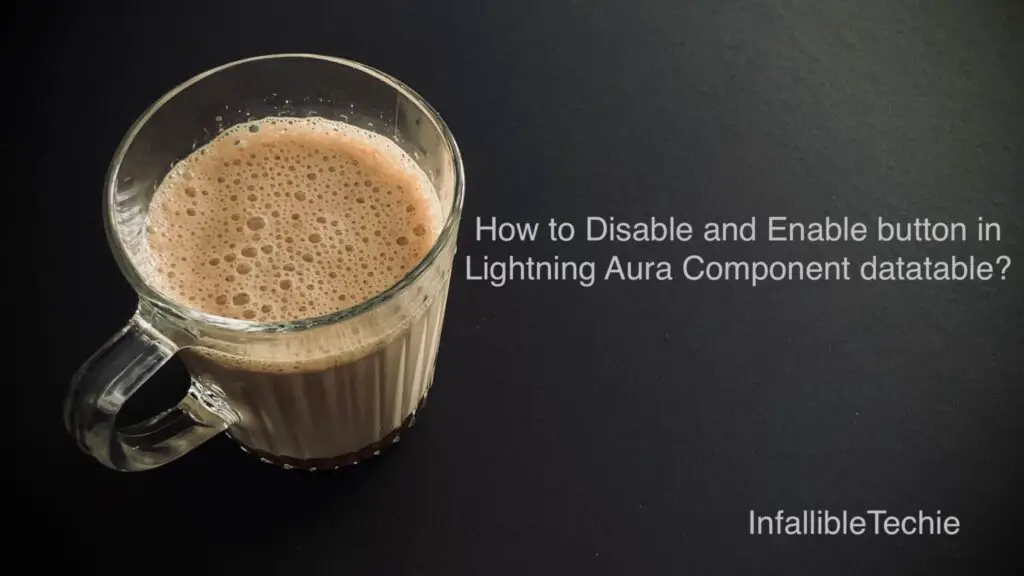
disabled attribute on the button type in the lightning:datatable can be used to Disable and Enable button.
Sample Code:
Apex Classes:
Wrapper Class:
public class AccountWrapper {
@AuraEnabled
public String accName;
@AuraEnabled
public Boolean isActive;
@AuraEnabled
public String accIndustry;
@AuraEnabled
public String accType;
@AuraEnabled
public String accActive;
@AuraEnabled
public String accId;
}
Controller Class:
public class AccountListController {
@AuraEnabled
public static List < AccountWrapper > fetchAccts() {
List < AccountWrapper > listAcctWrapper = new List < AccountWrapper >();
for ( Account acc : [
SELECT Id, Name, Industry, Type, Active__c
FROM Account
LIMIT 10
] ) {
AccountWrapper AccountWrap = new AccountWrapper();
AccountWrap.accName = acc.Name;
AccountWrap.isActive = acc.Active__c == 'Yes' ? true : false;
AccountWrap.accIndustry = acc.Industry;
AccountWrap.accType = acc.Type;
AccountWrap.accActive = acc.Active__c;
AccountWrap.accId = acc.Id;
listAcctWrapper.add(AccountWrap);
}
return listAcctWrapper;
}
}
Lightning Aura Component:
Component:
<aura:component implements="force:appHostable"
controller="AccountListController">
<aura:attribute type="AccountWrapper[]" name="acctList"/>
<aura:attribute name="mycolumns" type="List"/>
<aura:handler name="init" value="{!this}" action="{!c.fetchAccounts}"/>
<lightning:datatable data="{! v.acctList }"
columns="{! v.mycolumns }"
keyField="accId"
hideCheckboxColumn="true"
onrowaction="{!c.viewRecord}"/>
</aura:component>
Component Controller:
({
fetchAccounts : function( component, event, helper ) {
component.set( 'v.mycolumns', [
{ label: 'Account Name', fieldName: 'accName', type: 'text' },
{ label: 'Industry', fieldName: 'accIndustry', type: 'text' },
{ label: 'Type', fieldName: 'accType', type: 'text' },
{ label: 'Active?', fieldName: 'accActive', type: 'text' },
{ type: "button", typeAttributes: {
label: 'View',
name: 'View',
title: 'View',
disabled: { fieldName: 'isActive'},
value: 'view',
iconPosition: 'left'
} }
] );
let action = component.get( "c.fetchAccts" );
action.setCallback( this, function( response ) {
let state = response.getState();
if ( state === "SUCCESS" ) {
component.set(
"v.acctList",
response.getReturnValue()
);
}
});
$A.enqueueAction( action );
},
viewRecord : function( component, event, helper ) {
let recId = event.getParam( 'row' ).accId;
let actionName = event.getParam( 'action' ).name;
if ( actionName == 'View') {
let viewRecordEvent = $A.get( "e.force:navigateToURL" );
viewRecordEvent.setParams({
"url": "/" + recId
});
viewRecordEvent.fire();
}
}
})
Output:
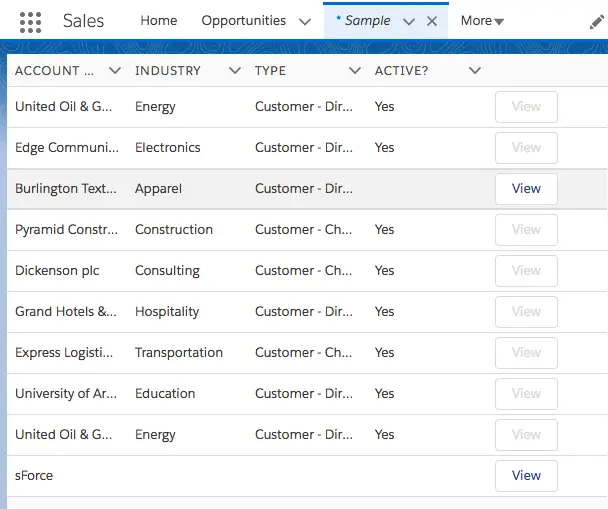