Sample Code:
Component:
<aura:component implements="force:appHostable"
controller="AccountListController">
<aura:attribute type="Account[]" name="acctList"/>
<aura:attribute name="mycolumns" type="List"/>
<aura:handler name="init" value="{!this}" action="{!c.init}"/>
<div style="height: 300px">
<lightning:datatable data="{! v.acctList }"
columns="{! v.mycolumns }"
keyField="Id"
hideCheckboxColumn="false"
enableInfiniteLoading="true"
onloadmore="{! c.loadMoreData }"
onrowselection="{! c.updateSelectedText }"
loadMoreOffset="5"/>
</div>
</aura:component>
Controller:
({
init : function(component, event, helper) {
console.log( 'Inside Init Method' );
component.set('v.mycolumns', [
{label: 'Account Name', fieldName: 'linkName', type: 'url',
typeAttributes: {label: { fieldName: 'Name' }, target: '_blank'}},
{label: 'Industry', fieldName: 'Industry', type: 'text'},
{label: 'Type', fieldName: 'Type', type: 'Text'}
]);
helper.fetchAccounts( component, event, 0 );
},
loadMoreData : function( component, event, helper ) {
event.getSource().set("v.isLoading", true);
component.set('v.loadMoreStatus', 'Loading');
helper.fetchAccounts(component, event, component.get('v.acctList').length);
},
updateSelectedText: function ( component, event, helper ) {
console.log( 'Inside updateSelectedText Method' );
let selectedRows = event.getParam( 'selectedRows' );
console.log( selectedRows.length );
}
})
Helper:
({
fetchAccounts : function(component, event, offSetCount) {
var action = component.get("c.fetchAccts");
action.setParams({
"intOffSet" : offSetCount
});
action.setCallback(this, function(response) {
var state = response.getState();
if (state === "SUCCESS") {
var records = response.getReturnValue();
records.forEach(function(record){
record.linkName = '/'+record.Id;
});
var currentData = component.get('v.acctList');
component.set("v.acctList", currentData.concat(records));
}
event.getSource().set("v.isLoading", false);
});
$A.enqueueAction(action);
}
})
Apex Class:
public class AccountListController {
@AuraEnabled
public static List < Account > fetchAccts( Integer intOffSet ) {
return [
SELECT Id, Name, Industry, Type
FROM Account
LIMIT 5 OFFSET : Integer.valueOf( intOffSet )
];
}
}
Output:
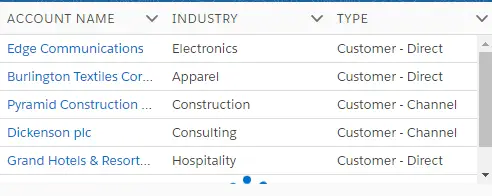