value attribute on the lightning:inputField tag can be used to set field values on lightning:recordEditForm. Check the following sample code for reference.
Sample Code:
Sample.cmp:
<aura:component implements="force:appHostable" >
<aura:attribute name="AccountId" type="String"/>
<div class="slds-box slds-theme_default">
<lightning:input type = "text" value = "{! v.AccountId }" label = "Account Id"/><br/>
<lightning:button label = "Create Opportunity" variant = "brand" onclick = "{! c.navigateToNewRecForm }"/>
</div>
</aura:component>
SampleController.js:
({
navigateToNewRecForm : function(component, event, helper) {
var evt = $A.get("e.force:navigateToComponent");
evt.setParams({
componentDef : "c:NewRecordForm",
componentAttributes: {
AccountId : component.get("v.AccountId")
}
});
evt.fire();
}
})
NewRecordForm.cmp:
<aura:component controller="NewRecordFormController">
<aura:attribute name="AccountId" type="String"/>
<aura:attribute name="AccountRec" type="Account"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}"/>
<div class="slds-box slds-theme_default">
<lightning:recordEditForm objectApiName = "Opportunity"
onsuccess = "{! c.handleSuccess }">
<lightning:inputField fieldName="Name" value="{! v.AccountRec.Name }"/>
<lightning:inputField fieldName="StageName"/>
<lightning:inputField fieldName="CloseDate"/>
<lightning:inputField fieldName="AccountId" value="{! v.AccountRec.Id }"/>
<lightning:button class="slds-m-top_small"
variant="brand"
type="submit"
name="Create" label="Update" />
</lightning:recordEditForm>
</div>
</aura:component>
NewRecordFormController.js:
({
doInit: function (component, event, helper) {
var action = component.get('c.getAccount');
action.setParams({
acctId : component.get("v.AccountId")
});
action.setCallback(this, function(response) {
var state = response.getState();
if ( state === 'SUCCESS' && component.isValid() ) {
component.set('v.AccountRec', response.getReturnValue());
}
});
$A.enqueueAction(action);
},
handleSuccess : function(component, event, helper) {
var params = event.getParams();
alert(params.response.id);
}
})
NewRecordFormController.apxc:
public class NewRecordFormController {
@auraEnabled
public static Account getAccount(String acctId) {
return [ SELECT Id, Name FROM Account WHERE Id =: acctId ];
}
}
Output:
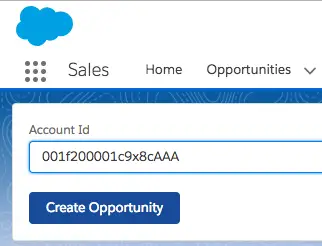
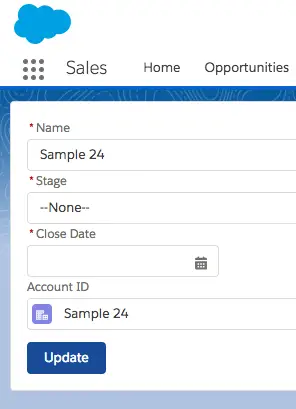