Sample code:
HTML:
<template>
<div class="slds-box slds-theme_default">
<table class="slds-table slds-table_cell-buffer slds-table_bordered slds-table_col-bordered">
<thead>
<tr>
<th class="" scope="col">
<div class="slds-truncate" title="Account Name">Name</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Account Industry">Industry</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Account Type">Type</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Account Number">Account Number</div>
</th>
<th class="" scope="col">
<div class="slds-truncate" title="Site">Site</div>
</th>
</tr>
</thead>
<tbody>
<template for:each={accounts} for:item="acc">
<tr key={acc.Id}>
<td>{acc.Name}</td>
<td>{acc.Industry}</td>
<td>{acc.Type}</td>
<td>{acc.AccountNumber}</td>
<td>{acc.Site}</td>
</tr>
</template>
</tbody>
</table>
</div>
</template>
JavaScript:
import { LightningElement, wire, track } from 'lwc';
import fetchAccounts from '@salesforce/apex/ExampleController.fetchAccounts';
export default class Example extends LightningElement {
@track accounts;
@wire( fetchAccounts )
caseRecord({ error, data }) {
if ( data ) {
this.accounts = data;
} else if ( error )
console.log( 'Error is ' + JSON.stringify( error ) );
}
}
JavaScript meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Apex Class:
public with sharing class ExampleController {
@AuraEnabled(cacheable=true)
public static List < Account > fetchAccounts() {
return [ SELECT Id, Name, Industry, Type, AccountNumber, Site FROM Account LIMIT 10 ];
}
}
Output:
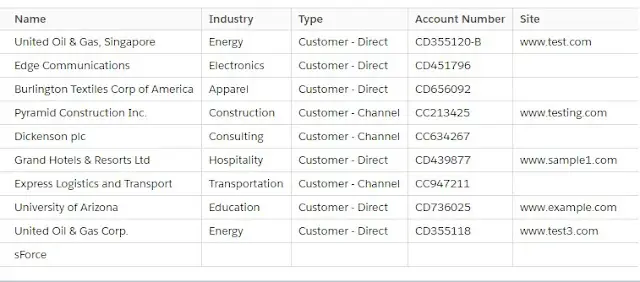