Sample Code:
Apex Class:
public with sharing class ExampleController {
@AuraEnabled(cacheable=true)
public static List < Account > fetchAccounts() {
return [ SELECT Id, Name, Industry FROM Account LIMIT 10 ];
}
}
Sample.html:
<template>
<div class="slds-theme--default">
<template if:true={isLoaded}>
<lightning-spinner alternative-text="Loading..." variant="brand">
</lightning-spinner>
</template>
<template for:each={accounts.data} for:item="acc">
<div key={acc.Id}>
<c-record-view-form rec-id={acc.Id}
s-object-name="Account"
field-name1="Name"
field-name2="Industry"
field-name3="NumberOfEmployees"
onloading={handleLoading}
ondoneloading={handleDoneLoading}>
</c-record-view-form>
</div>
</template>
</div>
</template>
Sample.js:
import { LightningElement, wire } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
import fetchAccounts from '@salesforce/apex/ExampleController.fetchAccounts';
export default class Sample extends NavigationMixin( LightningElement ) {
accounts;
isLoaded = false;
@wire(fetchAccounts)
accounts;
handleLoading() {
this.isLoaded = true;
}
handleDoneLoading() {
this.isLoaded = false;
}
}
Sample.js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
recordViewForm.html:
<template>
<lightning-record-view-form record-id={recId}
object-api-name={sObjectName}
density="compact">
<div class="slds-box">
<lightning-output-field field-name={fieldName1}>
</lightning-output-field>
<lightning-output-field field-name={fieldName2}>
</lightning-output-field>
<lightning-output-field field-name={fieldName3}>
</lightning-output-field>
</div>
</lightning-record-view-form>
</template>
recordViewForm.js:
import { LightningElement, api } from 'lwc';
export default class RecordViewForm extends LightningElement {
@api recId;
@api sObjectName;
@api fieldName1;
@api fieldName2;
@api fieldName3;
}
recordViewForm.js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
</LightningComponentBundle>
Output:
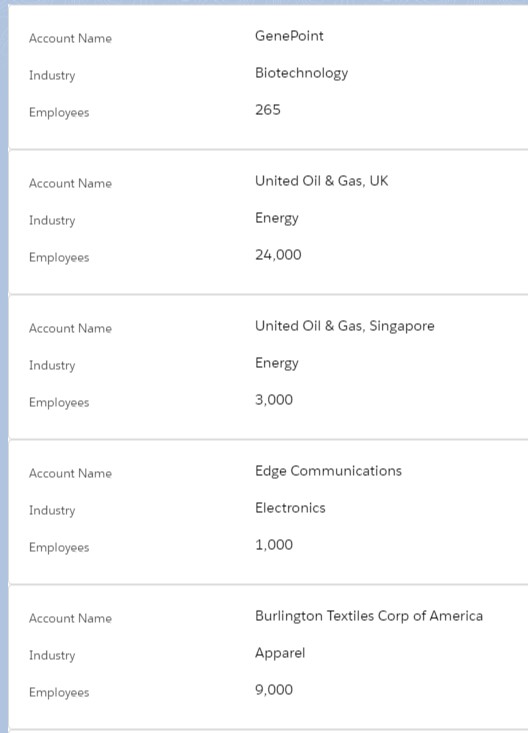