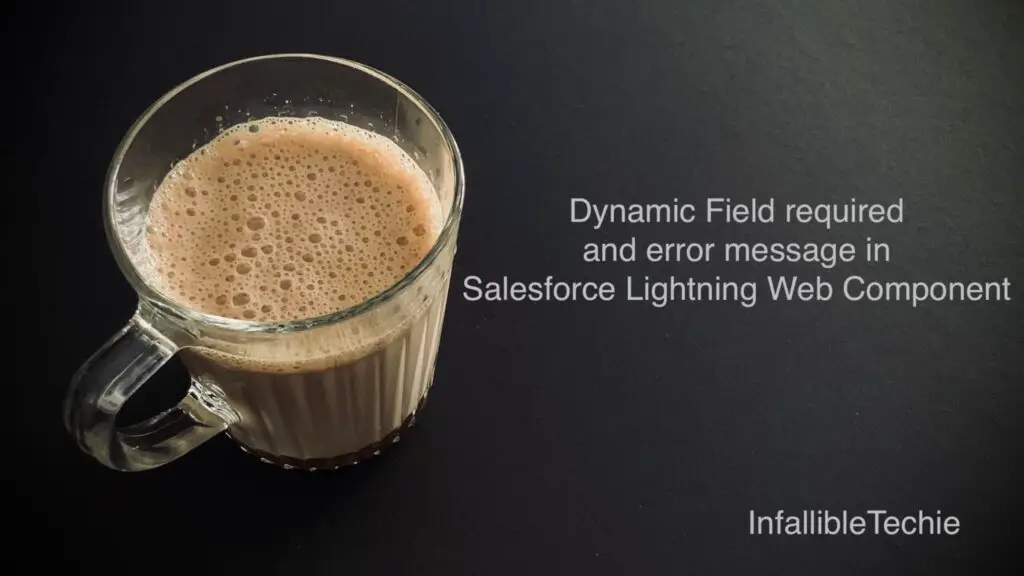
setCustomValidity() and reportValidity() methods can be used for dynamic Field required and error message in Salesforce Lightning Web Component.
Sample Code:
HTML:
<template>
<div class="slds-theme--default slds-p-around_large">
<lightning-input
type="toggle"
label="Basic option"
name="toggle"
onchange={onHandleValueChange}>
</lightning-input><br/><br/>
<lightning-input
type="text"
label="Enter some text"
name="text"
required={requiredBool}
class="inputText">
</lightning-input>
</div>
</template>
JavaScript:
import { LightningElement } from "lwc";
export default class sampleLightningWebComponent extends LightningElement {
requiredBool = false;
onHandleValueChange( event ) {
let selectedValue = event.detail.checked;
console.log(
"Event is",
JSON.stringify(
event.detail
)
);
console.log(
"Selected Value is",
selectedValue
);
let inputText = this.template.querySelector(
".inputText"
);
if ( selectedValue === true ) {
this.requiredBool = true;
if ( !inputText.value ) {
inputText.setCustomValidity(
"This field is required/mandatory"
);
inputText.reportValidity();
}
}
else {
let tempBool = false;
if ( !inputText.value ) {
/* Setting value to Temp to avoid
Value Missing error message */
inputText.value = "Temp";
tempBool = true;
}
inputText.setCustomValidity( '' );
inputText.reportValidity();
this.requiredBool = false;
if ( tempBool ) {
inputText.value = undefined;
}
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
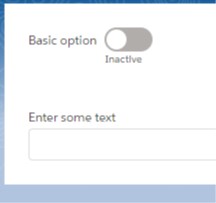
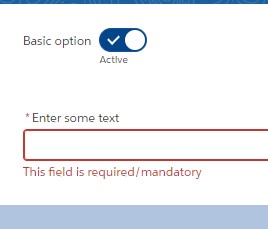