Sample Code:
HTML:
<template>
<div class="slds-box slds-theme_default">
<lightning-button label="Expand All"
onclick={handleClick}
name="Expand"
class="slds-m-left_x-small"></lightning-button>
<lightning-button label="Collapse All"
onclick={handleClick}
name="Collapse"
class="slds-m-left_x-small"></lightning-button>
<lightning-accordion allow-multiple-sections-open
active-section-name={sections}>
<template iterator:it={records}>
<lightning-accordion-section key={it.value.Id}
label={it.value.strTitle}
name={it.value.Id}>
{it.value.CommentBody}
</lightning-accordion-section>
</template>
</lightning-accordion>
</div>
</template>
JavaScript:
import { LightningElement, api, wire } from 'lwc';
import fetchCaseComments from '@salesforce/apex/CaseCommentController.fetchCaseComments';
export default class caseCommentLWC extends LightningElement {
@api recordId;
records;
error;
sections;
recIds = [];
@wire( fetchCaseComments, { strRecId: '$recordId' } )
wiredCaseComments( { error, data } ) {
if ( data ) {
let rows = JSON.parse( JSON.stringify( data ) );
console.log( 'Rows are ' + JSON.stringify( rows ) );
for ( let i = 0; i < rows.length; i++ ) {
let dataParse = rows[ i ];
dataParse.strTitle = dataParse.CreatedBy.Name + ' - ' + new Date( dataParse.CreatedDate ).toLocaleString();
this.recIds.push( dataParse.Id );
}
this.records = rows;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.records = undefined;
this.recIds = [];
}
}
handleClick( event ) {
const buttonName = event.target.name;
console.log( 'Button Name is ' + buttonName );
switch( buttonName ) {
case "Collapse":
this.sections = [];
break;
case "Expand":
this.sections = this.recIds;
break;
}
}
}
meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>49.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<property name="recordId" type="String" label="Record Id" description="Record Id"/>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Apex Class:
public with sharing class CaseCommentController {
@AuraEnabled( cacheable=true )
public static List < CaseComment > fetchCaseComments( String strRecId ) {
return [ SELECT Id, CommentBody, CreatedBy.Name, CreatedDate
FROM CaseComment
WHERE ParentId =: strRecId
ORDER BY CreatedDate DESC];
}
}
Output:
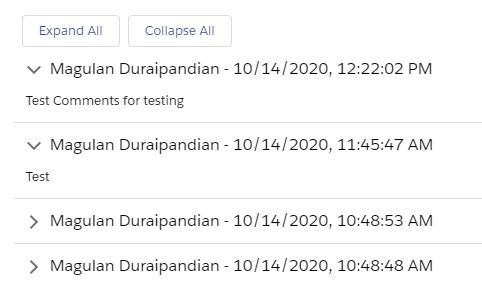