Sample Code:
HTML:
<template>
<div class="slds-p-around_medium lgc-bg">
<lightning-tree-grid columns={gridColumns}
data={gridData}
key-field="Name"
hide-checkbox-column=true>
</lightning-tree-grid>
</div>
</template>
JavaScript:
import { LightningElement, api, track, wire } from 'lwc';
import fetchAssetHierarchy from '@salesforce/apex/AssetHierarchyController.fetchAssetHierarchy';
export default class AssetHierarchy extends LightningElement {
@api recordId;
@track gridColumns = [{
type: 'text',
fieldName: 'Name',
label: 'Name'
},
{
type: 'text',
fieldName: 'SerialNumber',
label: 'Serial Number'
}];
@track gridData;
@wire(fetchAssetHierarchy, { assetId: '$recordId' })
AssetRecords( { error, data } ) {
console.log( 'Inside Wire Method' );
if ( data ) {
console.log( 'Data is ' + JSON.stringify( data ) );
this.gridData = JSON.parse( JSON.stringify( data ).split( 'children' ).join( '_children' ) );
} else if ( error ) {
console.log( 'Error is ' + JSON.stringify( error ) );
}
}
}
Apex Class:
public with sharing class AssetHierarchyController {
@AuraEnabled(cacheable=true)
public static List < HierarchyWrapper > fetchAssetHierarchy( String assetId ) {
Map < Id, HierarchyWrapper > mapAssetId = new Map < Id, HierarchyWrapper >();
Map < Id, List < Asset > > mapParentId = new Map < Id, List < Asset > >();
List < HierarchyWrapper > listWrap = new List < HierarchyWrapper >();
Asset currentAsset = [ SELECT Id, RootAssetId FROM Asset WHERE Id =: assetId ];
List < Asset > listAssets = [ SELECT Id, Name, SerialNumber, ParentId FROM Asset WHERE RootAssetId =: currentAsset.RootAssetId ORDER BY ParentId NULLS FIRST ];
Asset rootAsset = [ SELECT Id, Name, SerialNumber FROM Asset WHERE Id =: currentAsset.RootAssetId ];
HierarchyWrapper rootAssetWrap = new HierarchyWrapper();
rootAssetWrap.Name = rootAsset.Name;
rootAssetWrap.SerialNumber = rootAsset.SerialNumber;
rootAssetWrap.children = findRelations( listAssets, currentAsset.RootAssetId );
listWrap.add( rootAssetWrap );
return listWrap;
}
public static List < HierarchyWrapper > findRelations( List < Asset > listAssets, Id rootAssetId ) {
List < HierarchyWrapper > listWrap = new List < HierarchyWrapper >();
for ( Asset objAsset : listAssets ) {
if ( objAsset.ParentId == rootAssetId ) {
HierarchyWrapper wrap = new HierarchyWrapper();
wrap.Name = objAsset.Name;
wrap.SerialNumber = objAsset.SerialNumber;
wrap.children = findRelations( listAssets, objAsset.Id );
listWrap.add( wrap );
}
}
if ( listWrap.size() > 0 )
return listWrap;
else
return null;
}
}
public class HierarchyWrapper {
@AuraEnabled
public String Name;
@AuraEnabled
public String SerialNumber;
@AuraEnabled
public List < HierarchyWrapper > children;
}
JavaScript-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
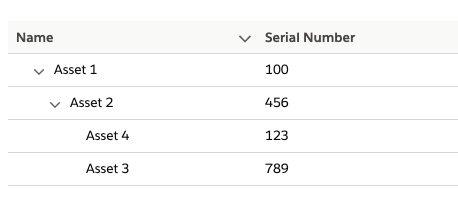