1. Enable Skills-Based Routing
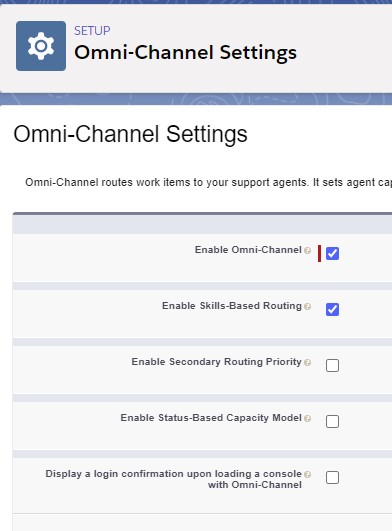
2. Setup Skills.
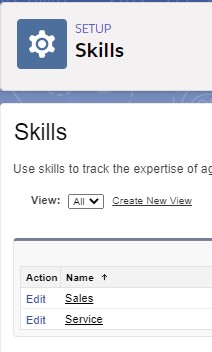
3. To determine the Skill dynamically, I am using the below Custom field.
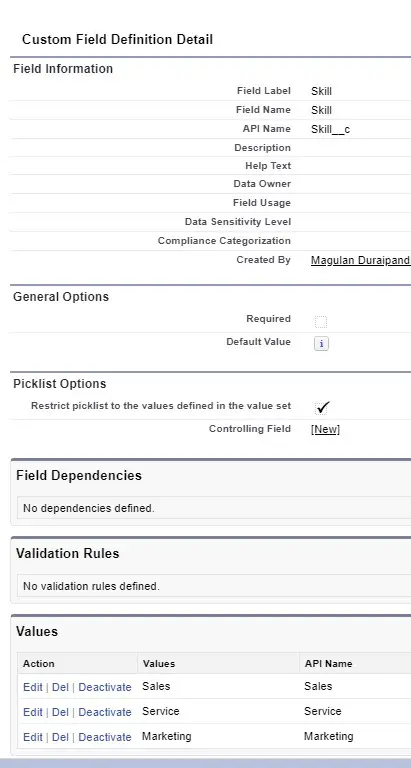
4. Service Resource record for an Agent.
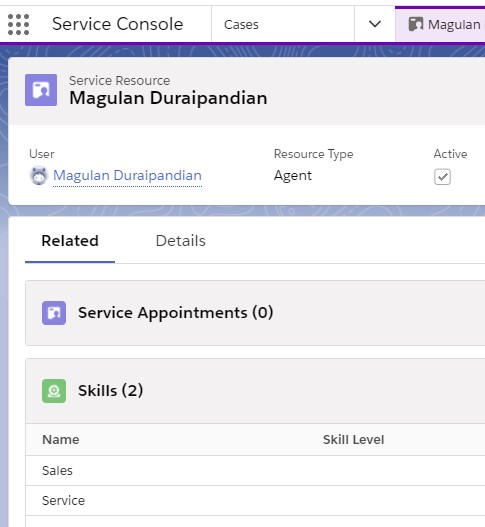
5. Sample Apex Class:
public class SkillsBasedRoutingController {
/*
Primary method to route records through Omni-Channel
*/
public static void routeUsingSkills( List < sObject > listRecords, String strEntity ) {
List < PendingServiceRouting > listPSRs = new List < PendingServiceRouting >();
String strChannelId = getChannelId( strEntity );
Map < String, Id > mapSkillId = getSkillId();
Map < Id, sObject > mapObj = new Map < Id, sObject >( listRecords );
for ( sObject obj : listRecords )
listPSRs.add( createPendingServiceRouting( obj, strChannelId ) );
if ( listPSRs.size() > 0 ) {
List < SkillRequirement > listSRs = new List < SkillRequirement >();
insert listPSRs;
for ( PendingServiceRouting objPSR : listPSRs ) {
objPSR.IsReadyForRouting = TRUE;
sObject obj = mapObj.get( objPSR.WorkItemId );
String strSkill = ( String ) obj.get( 'Skill__c' );
SkillRequirement objSR = new SkillRequirement(
RelatedRecordId = objPSR.Id,
SkillId = mapSkillId.get( strSkill ),
SkillLevel = 5
);
listSRs.add( objSR );
}
insert listSRs;
update listPSRs;
}
}
/*
Method to create Pending Service Routing record for each record
*/
static PendingServiceRouting createPendingServiceRouting( sObject obj, String strChannelId ) {
PendingServiceRouting psrObj = new PendingServiceRouting(
CapacityWeight = 1,
IsReadyForRouting = FALSE,
RoutingModel = 'MostAvailable',
RoutingPriority = 1,
RoutingType = 'SkillsBased',
ServiceChannelId = strChannelId,
WorkItemId = obj.Id,
PushTimeout = 0
);
return psrObj;
}
/*
Method to get the Channel Id based on the Entity
*/
static String getChannelId( String strEntity ) {
ServiceChannel channel = [ SELECT Id From ServiceChannel Where RelatedEntity =: strEntity ];
return channel.Id;
}
/*
Method to get Skills. If you have several skills, use WHERE condition in the SOQL to limit it.
*/
static Map < String, Id > getSkillId() {
Map < String, Id > mapSkillId = new Map < String, Id >();
for ( Skill objSkill : [ SELECT Id, DeveloperName From Skill ] )
mapSkillId.put( objSkill.DeveloperName, objSkill.Id );
return mapSkillId;
}
}
6. Sample Code to call the method.
List < Case > listCases = [
SELECT Id, Skill__c
FROM Case
WHERE Id = '5004W00001a3bSXQAY'
];
SkillsBasedRoutingController.routeUsingSkills(
listCases,
'Case'
);