Sample Code:
HTML:
<template>
<div class="slds-box slds-theme--default">
<div class="slds-text-color_inverse slds-text-heading_large" style="padding:0.5rem;background:#16325c">
Accounts
</div>
<table class="slds-table slds-table_cell-buffer slds-table_bordered slds-table_striped">
<thead>
<tr class="slds-line-height_reset">
<th class="" scope="col">
<a class="slds-th__action slds-text-link_reset" href="javascript:void(0);" role="button" tabindex="0" onclick={sortRecs} name="Name">
Account Name
<lightning-icon icon-name="utility:arrowup" size="x-small" if:true={nameUpBool}></lightning-icon>
<lightning-icon icon-name="utility:arrowdown" size="x-small" if:true={nameDWBool}></lightning-icon>
</a>
</th>
<th class="" scope="col">
<a class="slds-th__action slds-text-link_reset" href="javascript:void(0);" role="button" tabindex="0" onclick={sortRecs} name="AccountNumber">
Account Number
<lightning-icon icon-name="utility:arrowup" size="x-small" if:true={noUpBool}></lightning-icon>
<lightning-icon icon-name="utility:arrowdown" size="x-small" if:true={noDWBool}></lightning-icon>
</a>
</th>
<th class="" scope="col">
<a class="slds-th__action slds-text-link_reset" href="javascript:void(0);" role="button" tabindex="0" onclick={sortRecs} name="Industry">
Industry
<lightning-icon icon-name="utility:arrowup" size="x-small" if:true={indUpBool}></lightning-icon>
<lightning-icon icon-name="utility:arrowdown" size="x-small" if:true={indDWBool}></lightning-icon>
</a>
</th>
<th class="" scope="col">
<a class="slds-th__action slds-text-link_reset" href="javascript:void(0);" role="button" tabindex="0" onclick={sortRecs} name="Rating">
Rating
<lightning-icon icon-name="utility:arrowup" size="x-small" if:true={rateUpBool}></lightning-icon>
<lightning-icon icon-name="utility:arrowdown" size="x-small" if:true={rateDWBool}></lightning-icon>
</a>
</th>
<th class="" scope="col">
<a class="slds-th__action slds-text-link_reset" href="javascript:void(0);" role="button" tabindex="0" onclick={sortRecs} name="Type">
Type
<lightning-icon icon-name="utility:arrowup" size="x-small" if:true={typeUpBool}></lightning-icon>
<lightning-icon icon-name="utility:arrowdown" size="x-small" if:true={typeDWBool}></lightning-icon>
</a>
</th>
<th class="" scope="col">
<a class="slds-th__action slds-text-link_reset" href="javascript:void(0);" role="button" tabindex="0" onclick={sortRecs} name="Phone">
Phone
<lightning-icon icon-name="utility:arrowup" size="x-small" if:true={phoneUpBool}></lightning-icon>
<lightning-icon icon-name="utility:arrowdown" size="x-small" if:true={phoneDWBool}></lightning-icon>
</a>
</th>
</tr>
</thead>
<tbody>
<template iterator:it={records}>
<tr class="slds-hint-parent" key = {it.value.Id}>
<td data-label="Account Name">
<div class="slds-cell-wrap">{it.value.Name}</div>
</td>
<td data-label="Account Number">
<div class="slds-cell-wrap">{it.value.AccountNumber}</div>
</td>
<td data-label="Industry">
<div class="slds-cell-wrap">{it.value.Industry}</div>
</td>
<td data-label="Rating">
<div class="slds-cell-wrap">{it.value.Rating}</div>
</td>
<td data-label="Type">
<div class="slds-cell-wrap">{it.value.Type}</div>
</td>
<td data-label="Phone">
<div class="slds-cell-wrap">{it.value.Phone}</div>
</td>
</tr>
</template>
</tbody>
</table>
</div>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
export default class Sample extends LightningElement {
records;
sortedColumn;
sortedDirection;
nameUpBool;
nameDWBool;
noUpBool;
noDWBool;
indUpBool;
indDWBool;
rateUpBool;
rateDWBool;
typeUpBool;
typeDWBool;
phoneUpBool;
phoneDWBool;
@wire( fetchAccounts )
wiredAccount( { error, data } ) {
if (data) {
this.records = data;
this.initialRecords = data;
this.error = undefined;
this.sortedColumn = "Name";
this.sortRecs();
} else if ( error ) {
this.error = error;
this.initialRecords = undefined;
this.records = undefined;
}
}
sortRecs( event ) {
this.nameUpBool = false;
this.nameDWBool = false;
this.noUpBool = false;
this.noDWBool = false;
this.indUpBool = false;
this.indDWBool = false;
this.rateUpBool = false;
this.rateDWBool = false;
this.typeUpBool = false;
this.typeDWBool = false;
this.phoneUpBool = false;
this.phoneDWBool = false;
let colName = event ? event.target.name : undefined;
console.log( 'Column Name is ' + colName );
if ( this.sortedColumn === colName )
this.sortedDirection = ( this.sortedDirection === 'asc' ? 'desc' : 'asc' );
else
this.sortedDirection = 'asc';
let isReverse = this.sortedDirection === 'asc' ? 1 : -1;
if ( colName )
this.sortedColumn = colName;
else
colName = this.sortedColumn;
switch ( colName ) {
case "Name":
if ( this.sortedDirection == 'asc' )
this.nameUpBool = true;
else
this.nameDWBool = true;
break;
case "AccountNumber":
if ( this.sortedDirection == 'asc' )
this.noUpBool = true;
else
this.noDWBool = true;
break;
case "Industry":
if ( this.sortedDirection == 'asc' )
this.indUpBool = true;
else
this.indDWBool = true;
break;
case "Rating":
if ( this.sortedDirection == 'asc' )
this.rateUpBool = true;
else
this.rateDWBool = true;
break;
case "Type":
if ( this.sortedDirection == 'asc' )
this.typeUpBool = true;
else
this.typeDWBool = true;
break;
case "Phone":
if ( this.sortedDirection == 'asc' )
this.phoneUpBool = true;
else
this.phoneDWBool = true;
break;
}
this.records = JSON.parse( JSON.stringify( this.records ) ).sort( ( a, b ) => {
a = a[ colName ] ? a[ colName ].toLowerCase() : 'z';
b = b[ colName ] ? b[ colName ].toLowerCase() : 'z';
return a > b ? 1 * isReverse : -1 * isReverse;
});
}
}
Apex Class:
public with sharing class AccountController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [ SELECT Id, Name, Industry, AccountNumber, Rating, Type, Phone
FROM Account
LIMIT 10 ];
}
}
Output:
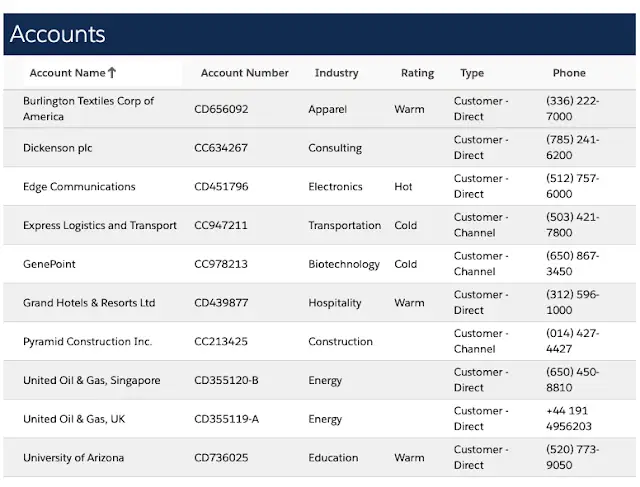
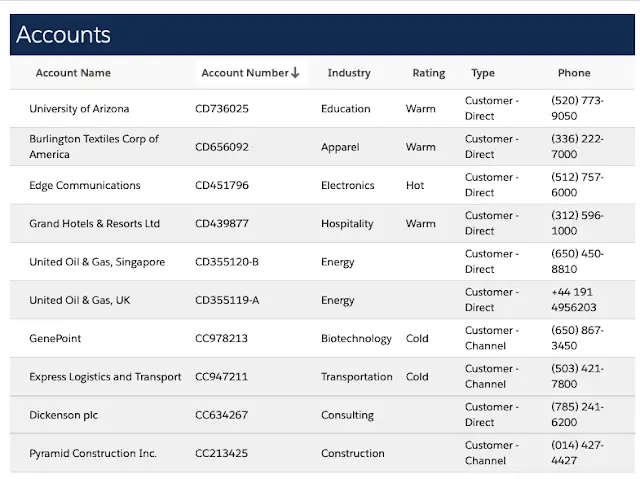