Sample code:
Lightning Web Component:
HTML:
<template>
<lightning-card>
Contact Name is {name}<br/><br/>
Contact Phone Number is {phone}
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire, api } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { getRecord } from 'lightning/uiRecordApi';
const FIELDS = ['Contact.Name', 'Contact.Phone'];
export default class GetRecordLwc extends LightningElement {
@api recordId;
contact;
name;
phone;
@wire( getRecord, { recordId: '$recordId', fields: FIELDS } )
wiredRecord({ error, data }) {
if ( error ) {
let message = 'Unknown error';
if (Array.isArray(error.body)) {
message = error.body.map(e => e.message).join(', ');
} else if (typeof error.body.message === 'string') {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent({
title: 'Error loading contact',
message,
variant: 'error',
}),
);
} else if ( data ) {
this.contact = data;
console.log( 'Contact is ' + JSON.stringify( this.contact ) );
this.name = this.contact.fields.Name.value;
this.phone = this.contact.fields.Phone.value;
}
}
}
JavaScript-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
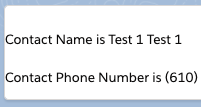