Sample Code:
Apex Class:
public with sharing class AccountController {
@AuraEnabled( cacheable = true )
public static AccountWrapper fetchAccounts() {
AccountWrapper wrap = new AccountWrapper();
wrap.listAcc = [ SELECT Id, Name, Industry, AccountNumber
FROM Account
LIMIT 10 ];
wrap.accCount = wrap.listAcc.size();
return wrap;
}
public class AccountWrapper {
@AuraEnabled
public List < Account > listAcc;
@AuraEnabled
public Integer accCount;
}
}
HTML:
<template>
<div class="slds-box slds-theme--default">
<template if:true = {records}>
No of Accounts : {intCount}
<div style = "height: 300px;">
<table class="slds-table slds-table_cell-buffer slds-table_bordered slds-table_striped">
<tr>
<th scope = "col">Name</th>
<th scope = "col">Industry</th>
<th scope = "col">Account Number</th>
</tr>
<template iterator:it = {records}>
<tr key = {it.value.Id}>
<td>{it.value.Name}</td>
<td>{it.value.Industry}</td>
<td>{it.value.AccountNumber}</td>
</tr>
</template>
</table>
</div>
</template>
<template if:true = {error}>
{error}>
</template>
</div>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountController.fetchAccounts';
export default class Sample extends LightningElement {
records;
error;
intCount
@wire( fetchAccounts )
wiredAccount( { error, data } ) {
if ( data ) {
console.log( 'Data from Apex' + JSON.stringify( data ) );
this.records = data.listAcc;
this.intCount = data.accCount;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.records = undefined;
}
}
}
JavaScript-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>49.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
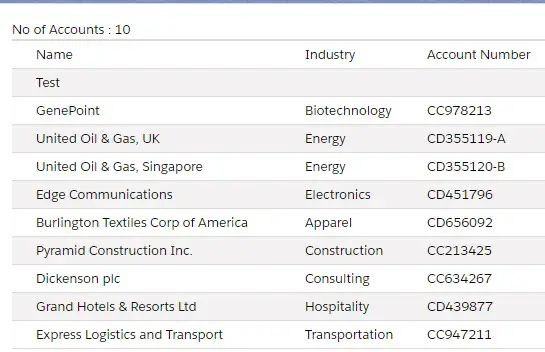