Sample Code:
Apex Class:
public with sharing class ProductController {
@AuraEnabled( cacheable = true )
public static List< PricebookEntry > fetchProducts() {
return [
SELECT Id, Product2.Name, IsActive, UnitPrice, Product2.Family
FROM PricebookEntry
LIMIT 10
];
}
}
Lightning Web Component:
HTML:
<template>
<lightning-card>
<lightning-datatable
key-field="Id"
data={records}
columns={columns}
hide-checkbox-column="true">
</lightning-datatable>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchProducts from '@salesforce/apex/ProductController.fetchProducts';
const columns = [
{ label: 'Product Name', fieldName: 'Name' },
{ label: 'Product Family', fieldName: 'Family' },
{ label: 'Unit Price', fieldName: 'UnitPrice', type: 'currency' },
{ label: 'Is Active?', fieldName: 'IsActive', type: 'boolean' }
];
export default class SampleLWC extends LightningElement {
records;
wiredRecords;
error;
columns = columns;
draftValues = [];
@wire( fetchProducts )
wiredAccount( value ) {
this.wiredRecords = value;
const { data, error } = value;
if ( data ) {
let tempRecords = JSON.parse( JSON.stringify( data ) );
tempRecords = tempRecords.map( row => {
return { ...row, Name: row.Product2.Name, Family: row.Product2.Family };
})
this.records = tempRecords;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.records = undefined;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>50.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
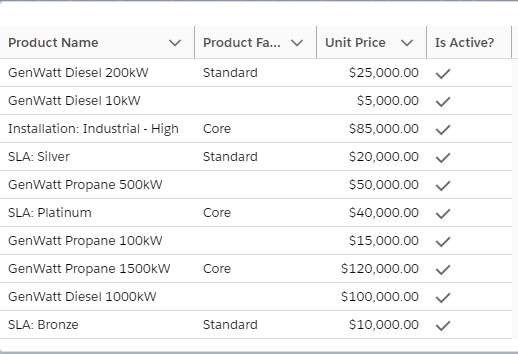