Sample CSV File:
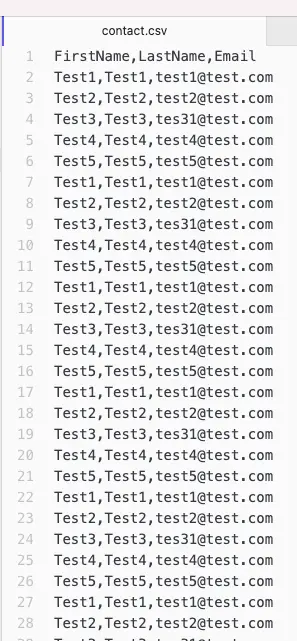
Sample code:
Apex:
public with sharing class FileUploadController {
@AuraEnabled
public static String loadData( Id contentDocumentId ) {
String strMessage;
List < Contact > contactList = new List < Contact >();
if ( contentDocumentId != null ) {
ContentVersion contentVersionObj = [ SELECT Id, VersionData FROM ContentVersion WHERE ContentDocumentId =:contentDocumentId ];
List < String > records = contentVersionObj.VersionData.toString().split( 'n' );
//Removing the Header
records.remove( 0 );
for ( String record : records ) {
if ( record != null ) {
Contact objContact = new Contact();
List < String > row = record.split( ',' );
objContact.FirstName = row[ 0 ];
objContact.LastName = row[ 1 ];
objContact.Email = row[ 2 ];
contactList.add( objContact );
}
}
try {
if ( contactList.size() > 0 ) {
Database.DeleteResult deleteResult = Database.delete( contentDocumentId, true );
insert contactList;
strMessage = 'Records loaded successfully';
}
}
catch ( Exception e ) {
strMessage = 'Some error occured. Please reach out to your System Admin';
system.debug( e.getMessage() );
}
}
return strMessage;
}
}
HTML:
<template>
<template if:true={isLoaded}>
<lightning-spinner
alternative-text="Loading"
size="large">
</lightning-spinner>
</template>
<lightning-card variant="Narrow" icon-name="standard:file" title="File Upload">
<p class="slds-var-p-horizontal_small">
<lightning-file-upload
accept={acceptedFormats}
label="Attach CSV File"
multiple="multiple"
onuploadfinished={uploadFileHandler}>
</lightning-file-upload>
</p>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import {ShowToastEvent} from 'lightning/platformShowToastEvent';
import loadData from '@salesforce/apex/FileUploadController.loadData';
export default class FileUpload extends LightningElement {
error;
isLoaded = false;
get acceptedFormats() {
return ['.csv'];
}
uploadFileHandler( event ) {
this.isLoaded = true;
const uploadedFiles = event.detail.files;
loadData( { contentDocumentId : uploadedFiles[0].documentId } )
.then( result => {
this.isLoaded = false;
window.console.log('result ===> '+result);
this.strMessage = result;
this.dispatchEvent(
new ShowToastEvent( {
title: 'Success',
message: result,
variant: result.includes("success") ? 'success' : 'error',
mode: 'sticky'
} ),
);
})
.catch( error => {
this.isLoaded = false;
this.error = error;
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error!!',
message: JSON.stringify( error ),
variant: 'error',
mode: 'sticky'
} ),
);
} )
}
}
meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
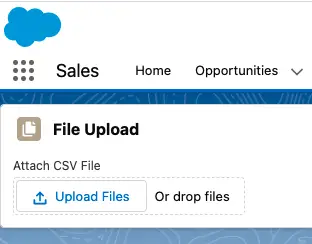
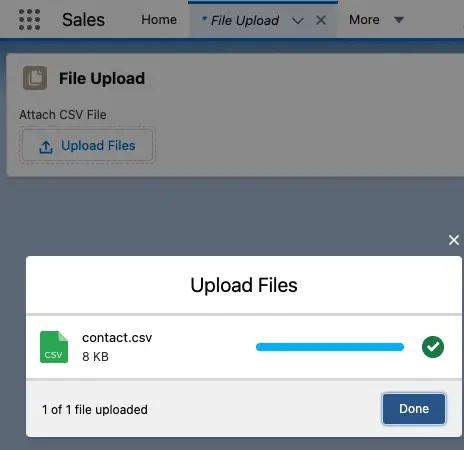
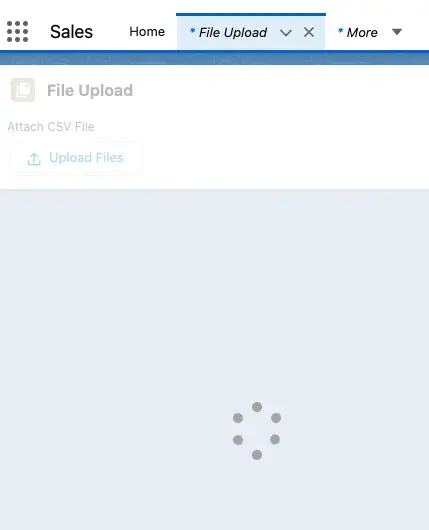
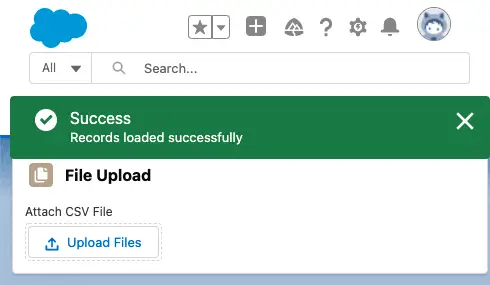
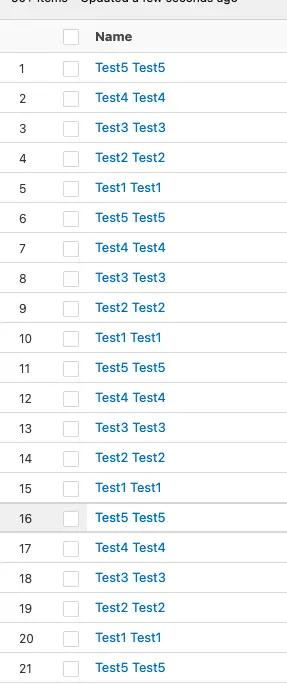