Sample Code:
CMP:
<aura:component implements="force:appHostable" controller="SampleAuraController">
<lightning:card>
<lightning:recordEditForm aura:id="recordEditForm" objectApiName="Lead" onsubmit="{!c.doSubmit}">
<lightning:messages />
<lightning:inputField fieldName="FirstName" />
<lightning:inputField fieldName="LastName" />
<lightning:inputField fieldName="Email" />
<lightning:inputField fieldName="Phone" />
<lightning:inputField fieldName="Company" />
<div id="recaptchaCheckbox"></div>
<br/>
<lightning:button aura:id="myButton" label="Create Lead" type="submit"/>
</lightning:recordEditForm>
</lightning:card>
</aura:component>
JS:
({
doSubmit: function ( component, event, helper ) {
console.log( 'Inside Submit' );
event.preventDefault();
let fields = event.getParam( 'fields' );
fields = Object.assign( { 'sobjectType': 'Lead' }, fields );
console.log( 'Fields are ' + JSON.stringify( fields ) );
let action = component.get( "c.insertRecord" );
action.setParams( {
record: fields
} );
action.setCallback( this, function( response ) {
document.dispatchEvent(new Event( "grecaptchaReset" ) );
let myButton = component.find( "myButton" );
myButton.set( 'v.disabled', true );
let state = response.getState();
if ( state === "SUCCESS" ) {
let result = response.getReturnValue();
console.log( 'Result is ' + JSON.stringify( result ) );
if ( result === 'Success' ) {
let showToast = $A.get( "e.force:showToast" );
showToast.setParams( {
title : 'Lead Creation',
message : 'Lead Submitted Sucessfully.' ,
type : 'success',
mode : 'dismissible'
} );
showToast.fire();
}
} else {
let errors = response.getError();
if ( errors ) {
console.log( errors[ 0 ] );
}
let showToast = $A.get( "e.force:showToast" );
showToast.setParams( {
title : 'Lead Creation',
message : 'Lead is not Submitted due to some error.' ,
type : 'error',
mode : 'dismissible'
} );
showToast.fire();
}
});
$A.enqueueAction(action);
}
})
Apex Class:
public class SampleAuraController {
@AuraEnabled
public static String insertRecord( SObject record ) {
try {
insert record;
return 'Success';
} Catch( Exception e ) {
return 'Failed';
}
}
}
Output:
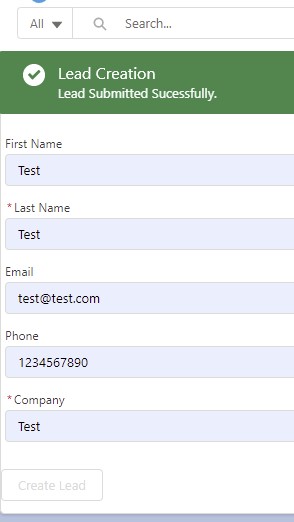