Quick Action Component:
HTML:
<template>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
export default class SampleQuickAction extends NavigationMixin( LightningElement ) {
@api recordId;
@api invoke() {
this[NavigationMixin.Navigate]( {
type: 'standard__navItemPage',
attributes: {
apiName: 'Sample_Tab'
},
state: {
c__recId: this.recordId
}
} );
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>Action</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Tab Component:
HTML:
<template>
<lightning-card>
<template if:true={isLoaded}>
<lightning-spinner alternative-text="Loading..." variant="brand"></lightning-spinner>
</template>
<template if:true={recordBool}>
{recId}
</template>
<template if:false={recordBool}>
Record Id is not passed
</template>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import { CurrentPageReference } from 'lightning/navigation';
export default class SampleTab extends LightningElement {
recordBool = false;
recId;
currentPageReference;
connectedBool = false;
constructor() {
super();
console.log( 'Inside Constructor' );
this.connectedBool = true;
}
@wire( CurrentPageReference )
setCurrentPageReference( currentPageReference ) {
this.currentPageReference = currentPageReference;
let tempRecId = this.currentPageReference.state.c__recId;
console.log( 'Rec Id is ' + tempRecId );
if ( tempRecId ) {
this.recordBool = true;
this.recId = tempRecId;
} else {
this.recordBool = false;
this.recId = undefined;
if ( !this.connectedBool )
location.reload();
this.connectedBool = false;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Quick Action:
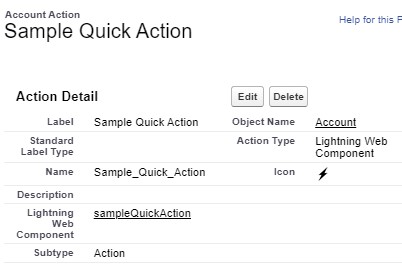
Tab:
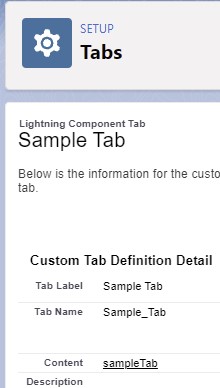
Output:
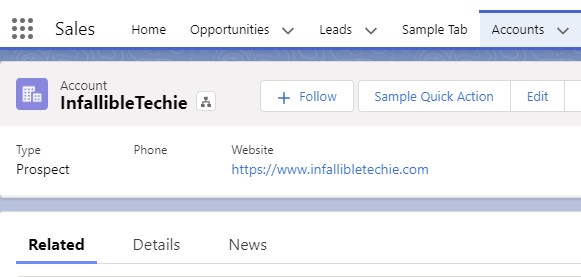
Navigated from Account:
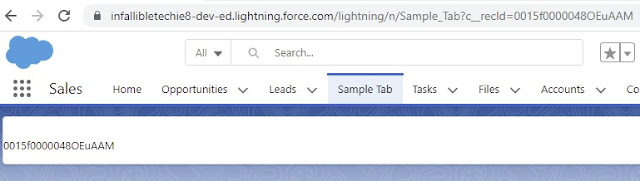
Clicking Sample Tab after navigating from Account(This removes the query parameter):
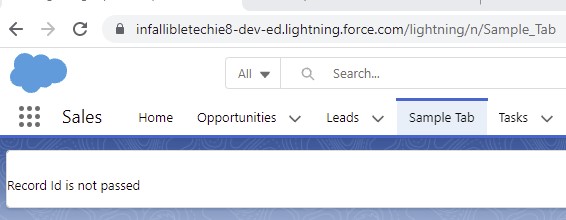