Geolocation Field:
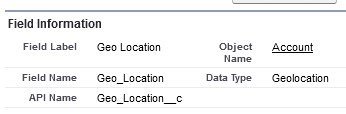
Sample code:
HTML:
<template>
<lightning-card>
<b>Account Geo Location information:</b><br/>
Latitude is {latitude}<br/>
Longitude is {longitude}
</lightning-card>
</template>
JavaScript:
import { LightningElement, api, wire } from 'lwc';
import { getRecord } from 'lightning/uiRecordApi';
const FIELD_Latitude ='Account.Geo_Location__Latitude__s';
const FIELD_Longitude ='Account.Geo_Location__Longitude__s';
const FIELDS = [ FIELD_Latitude, FIELD_Longitude ];
export default class GeoLocationGetRecord extends LightningElement {
@api recordId;
account;
longitude;
latitude;
@wire( getRecord, { recordId: '$recordId', fields: FIELDS } )
wiredRecord({ error, data }) {
if ( error ) {
let message = 'Unknown error';
if ( Array.isArray( error.body ) ) {
message = error.body.map(e => e.message).join( ', ' );
} else if ( typeof error.body.message === 'string' ) {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error loading Account',
message,
variant: 'error',
} ),
);
} else if ( data ) {
this.account = data;
console.log( 'Account is ' + JSON.stringify( this.account ) );
this.longitude = this.account.fields.Geo_Location__Longitude__s.value;
this.latitude = this.account.fields.Geo_Location__Latitude__s.value;
}
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed> <targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
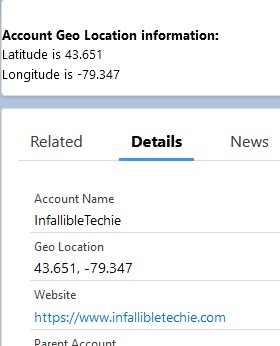