Sample Code:
Apex Class:
public class SampleAuraController {
@AuraEnabled
public static List < Account > fetchAccts() {
return [
SELECT Id, Name, Industry, Is_Active__c
FROM Account
LIMIT 10
];
}
@AuraEnabled
public static void updateAccounts( List < Account > listAccounts ) {
System.debug( 'Accounts are ' + listAccounts );
update listAccounts;
}
}
Aura Component:
<aura:component implements="force:appHostable" controller="SampleAuraController">
<aura:attribute type="Account[]" name="acctList"/>
<aura:handler name="init" value="{!this}" action="{!c.fetchAccounts}"/>
<lightning:card>
<table class="slds-table slds-table_bordered slds-table_cell-buffer">
<thead>
<tr class="slds-text-title_caps">
<th scope="col">
<div class="slds-truncate" title="Account Name">Account Name</div>
</th>
<th scope="col">
<div class="slds-truncate" title="Industry">Industry</div>
</th>
<th scope="col">
<div class="slds-truncate" title="Is Active?">Is Active?</div>
</th>
</tr>
</thead>
<tbody>
<aura:iteration items="{!v.acctList}" var="a" indexVar="index">
<tr>
<td data-label="Account Name">
<div class="slds-truncate">{!a.Name}</div>
</td>
<td data-label="Industry">
<div class="slds-truncate">{!a.Industry}</div>
</td>
<td data-label="Is Active?">
<div class="slds-truncate" data-record-id="{!index}">
<lightning:input type="toggle" variant="label-hidden" checked="{!a.Is_Active__c}" name="{!a.Is_Active__c}" onchange="{! c.onToggleChange }"></lightning:input>
</div>
</td>
</tr>
</aura:iteration>
</tbody>
</table>
<aura:set attribute="footer">
<lightning:button variant="brand" label="Save Records" onclick="{! c.saveRecords }"/>
</aura:set>
</lightning:card>
</aura:component>
Java Script:
({
fetchAccounts : function( component, event, helper ) {
let action = component.get( "c.fetchAccts" );
/*action.setParams({
});*/
action.setCallback(this, function(response){
let state = response.getState();
if ( state === "SUCCESS" ) {
component.set( "v.acctList", response.getReturnValue() );
}
});
$A.enqueueAction( action );
},
saveRecords : function ( component, event, helper ) {
console.log( "Updated Account values " + JSON.stringify( component.get( "v.acctList" ) ) );
let action = component.get( "c.updateAccounts" );
action.setParams({
listAccounts : component.get( "v.acctList" )
});
action.setCallback(this, function(response){
let state = response.getState();
if ( state === "SUCCESS" ) {
console.log( 'Success' );
}
});
$A.enqueueAction( action );
},
onToggleChange : function ( component, event, helper ) {
let indexVal = event.target.dataset.recordId;
console.log( "indexVal is " + JSON.stringify( indexVal ) );
let tempAccList = component.get( "v.acctList" );
let currentRec = tempAccList[ indexVal ];
console.log( "Current Value is " + JSON.stringify( currentRec ) );
}
})
Note:
Is_Active__c is a check box field in Account object.
Output:
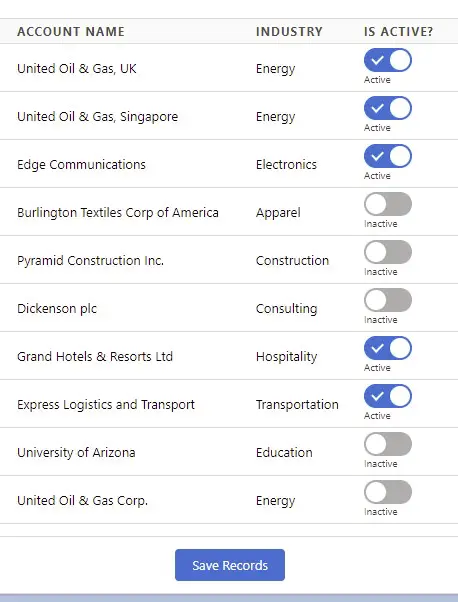