Use ‘$variableName’ while calling wire method instead of using this.variableName. This will avoid the undefined variable issue while calling Wire method in Salesforce.
Check the following sample code. ‘$userId’ will not throw any error. But, if this.userId is used, it will throw undefined exception.
Sample Code:
Lightning Web Component:
HTML:
<template>
<lightning-card>
<b>{name} - </b>
<img
src={imageURL}
alt="User Image"
height="100px"
width="100px"/>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import IdOfUser from '@salesforce/user/Id';
import { getRecord } from 'lightning/uiRecordApi';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
const FIELDS = [
'User.Name',
'User.Image__c'
];
export default class UserImage extends LightningElement {
userId = IdOfUser;
name;
imageURL;
connectedCallback() {
console.log( 'Id of the user is ' + this.userId );
console.log( 'Fields are ' + JSON.stringify( FIELDS ) );
}
@wire(getRecord, { recordId: '$userId', fields: FIELDS })
wiredRecord({ error, data }) {
if ( error ) {
let message = 'Unknown error';
if (Array.isArray(error.body)) {
message = error.body.map(e => e.message).join(', ');
} else if (typeof error.body.message === 'string') {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent({
title: 'Error loading Account',
message,
variant: 'error',
}),
);
} else if ( data ) {
console.log( 'Data is ' + JSON.stringify( data ) );
this.name = data.fields.Name.value;
this.imageURL = data.fields.Image__c.value;
}
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
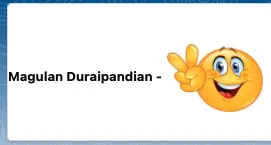