In the below implementation, I have created Custom quick action with Lightning Web Component to forward the records(Accounts) to the target org.
Pre-requisite:
Salesforce to Salesforce Setup:
Sample code:
Apex Class:
public class ForwardRecordToSFController {
@AuraEnabled
public static String forwardRecord( String recId ) {
List < PartnerNetworkRecordConnection > checkConnection = [ SELECT Id FROM PartnerNetworkRecordConnection WHERE LocalRecordId =: recId ];
if ( checkConnection.size() > 0 ) {
return 'Record is already shared to the other org';
} else {
PartnerNetworkConnection network = [ SELECT Id FROM PartnerNetworkConnection WHERE ConnectionStatus = 'Accepted' LIMIT 1 ];
PartnerNetworkRecordConnection connection = new PartnerNetworkRecordConnection();
connection.ConnectionId = network.Id;
connection.LocalRecordId = recId;
insert connection;
return 'Record forwarded successfully';
}
}
}
Lightning Web Component:
HTML:
<template>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import forwardRecord from '@salesforce/apex/ForwardRecordToSFController.forwardRecord';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class SalesforceToSalesforce extends LightningElement {
@api recordId;
@api async invoke() {
await forwardRecord( { recId : this.recordId } )
.then ( result => {
console.log( result );
if ( result.includes( 'success' ) ) {
this.dispatchEvent(
new ShowToastEvent({
title: 'Approval',
message: result,
variant: 'success',
}),
);
} else {
this.dispatchEvent(
new ShowToastEvent({
title: 'Approval',
message: result,
variant: 'error',
}),
);
}
})
.catch( error => {
console.log( JSON.stringify( error ) );
});
}
}
JS-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>Action</actionType>
<objects>
<object>Account</object>
</objects>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Quick Action:
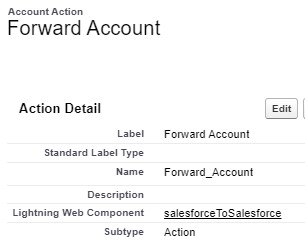
Output:
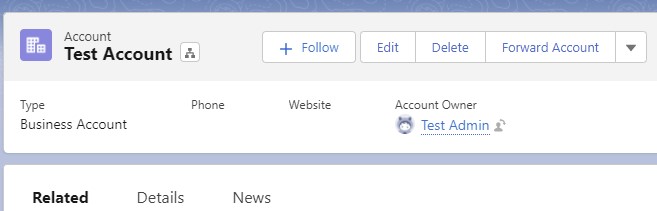