Sample Code:
Visualforce Page:
<apex:page controller="SampleVFPageController">
<apex:form >
<apex:pageBlock >
<apex:pageBlockSection >
<apex:inputText label="Enter Account Name" value="{!strAccName}"/>
</apex:pageBlockSection>
<apex:pageBlockButtons >
<apex:commandButton value="Fetch Account" action="{!fetchAccounts}"/>
</apex:pageBlockButtons>
</apex:pageBlock>
<apex:pageBlock rendered="{! listAccounts.size > 0 }">
<apex:pageBlockTable value="{!listAccounts}" var="objAcc">
<apex:column value="{!objAcc.Name}"/>
<apex:column value="{!objAcc.Industry}"/>
</apex:pageBlockTable>
</apex:pageBlock>
<apex:pageMessage summary="No Matching Accounts found!!!"
severity="info"
rendered="{! AND( NOT( ISBLANK( strAccName ) ), listAccounts.size == 0 ) }" />
</apex:form>
</apex:page>
Apex Class:
public class SampleVFPageController {
public String strAccName { get; set; }
public List < Account > listAccounts { get; set; }
public SampleVFPageController() {
listAccounts = new List < Account >();
}
public void fetchAccounts() {
try {
String strAccNamePattern = '%' + strAccName + '%';
listAccounts = [
SELECT Id, Name, Industry
FROM Account
WHERE Name LIKE: String.escapeSingleQuotes( strAccNamePattern )
];
} catch ( Exception e ) {
ApexPages.addMessage(
new ApexPages.Message( ApexPages.Severity.ERROR, e.getMessage() )
);
}
}
}
Output:
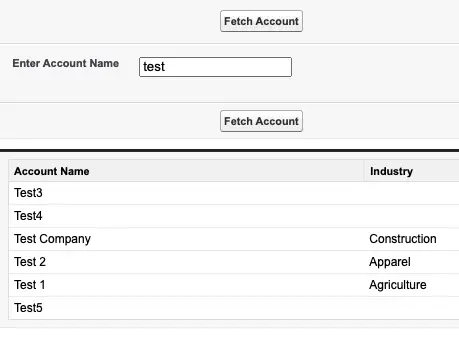
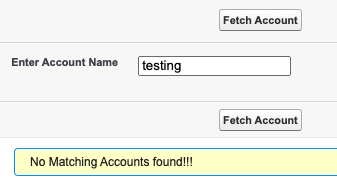