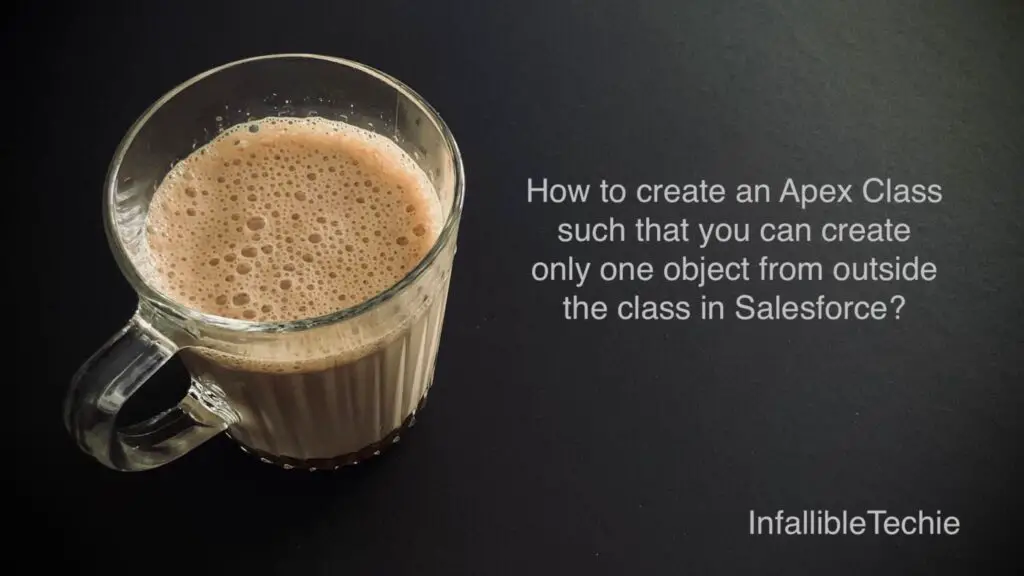
The Singleton pattern attempts to solve the issue of repeatedly using an object instance, but only allowing to instantiate it once within a single transaction context. So, using Singleton pattern , we can create Salesforce Apex Class to create one object per transaction.
Sample Class:
public class SampleClass {
private static SampleClass objInstance = null;
public String str1;
public String str2;
private SampleClass() {
str1 = 'String1';
str2 = 'String2';
System.debug( 'Inside Constructor' );
}
public static SampleClass getInstance () {
if (objInstance == null) {
objInstance = new SampleClass();
}
return objInstance ;
}
}
To test, execute the following the code:
for ( Integer i = 0; i < 3; i++ ) {
SampleClass obj = SampleClass.getInstance();
System.debug( 'str1 is ' + obj.str1 );
System.debug( 'str2 is ' + obj.str2 );
}
Output:
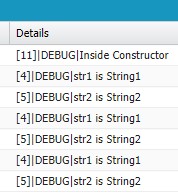
In the above example, Constructor was called only once. So, the object was initialised only once.