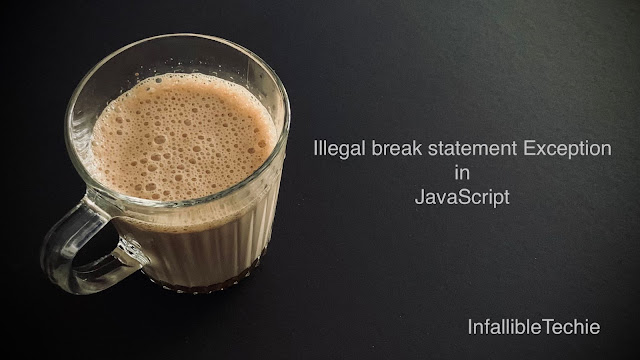
break Statement is used to break out of a loop statements like for, while, switch etc. We cannot use it for other purpose.
Sample Code to Reproduce the Exception:
let numArray = [ 1, 2, 3, 4, 5, 6 ];
let output = 0;
numArray.forEach( val => {
output += val;
if ( output > 5 ) {
break;
}
} );
console.log( 'Output is', output );
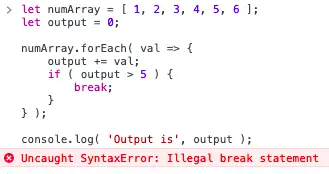
every method should be used if you are not using loop statements.
Fix for the above code:
let numArray = [ 1, 2, 3, 4, 5, 6 ];
let output = 0;
numArray.every( val => {
output += val;
if ( output > 5 ) {
return false;
}
return true;
} );
console.log( 'Output is', output );
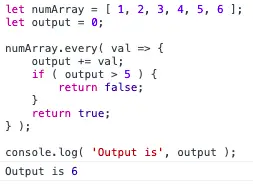