Using lightning:workspaceAPI and force:serviceCloudVoiceToolkitApi can be used to focus Voice Call records tab for Salesforce Service Cloud Voice Outbound Calls when made by the agents.
Sample Lightning Component:
Component:
<aura:component implements="flexipage:availableForAllPageTypes" access="global">
<lightning:workspaceAPI aura:id="workspace"/>
<force:serviceCloudVoiceToolkitApi aura:id="voiceToolkitApi"/>
<aura:handler name="init" value="{!this}" action="{!c.onInit}"/>
</aura:component>
Controller:
({
onInit : function( component, event, helper ) {
console.log( 'Inside onInit Method' );
helper.subscribeToVoiceToolkit( component );
}
})
Helper:
({
subscribeToVoiceToolkit: function( component ) {
component.callStartedListener = $A.getCallback(
this.onCallStarted.bind( this, component )
);
component.callConnectedListener = $A.getCallback(
this.onCallConnected.bind( this, component )
);
component.callEndedListener = $A.getCallback(
this.onCallEnded.bind( this, component )
);
component.find( 'voiceToolkitApi' ).addTelephonyEventListener(
'CALL_STARTED', component.callStartedListener
);
component.find( 'voiceToolkitApi' ).addTelephonyEventListener(
'CALL_CONNECTED', component.callConnectedListener
);
component.find( 'voiceToolkitApi' ).addTelephonyEventListener(
'CALL_ENDED', component.callEndedListener
);
},
onCallStarted: function( component, event ) {
console.log( 'Call Started' );
/*
* Adding 3 seconds delay so that Voice Call record will load
*/
setTimeout( $A.getCallback( function() {
let strCallType = event.detail.callType;
if ( strCallType ) {
console.log(
'Call Type is',
strCallType
);
if ( strCallType == 'outbound' ) {
console.log(
'Inside Outbound block'
);
let workspaceAPI = component.find( "workspace" );
/*
* Getting current Tab record from which the outbound call is made
* Example: Contact Record
*/
workspaceAPI.getFocusedTabInfo().then( function( response ) {
workspaceAPI.getTabInfo({
tabId: response.tabId
}).then( function( response ) {
if ( response.subtabs ) {
/*
* When the call is made, Voice Call will open as Sub-tab.
* So, getting all the Sub-tabs.
*/
let allSubtabs = response.subtabs;
console.log(
'Response is',
JSON.stringify( allSubtabs )
);
for ( let i = 0; i < allSubtabs.length; i++ ) {
let tempArrayItem = allSubtabs[ i ];
let tempRecordId = tempArrayItem.recordId;
if ( tempRecordId ) {
/*
* Checking whether the Sub-tab is Voice Call Record
*/
if ( tempRecordId.startsWith( '0LQ' ) ) {
let voiceCallTabId = tempArrayItem.tabId;
console.log(
'voiceCallTabId is',
voiceCallTabId
);
if ( voiceCallTabId ) {
workspaceAPI.focusTab(
{ tabId : voiceCallTabId }
);
}
break;
}
}
}
}
} );
} )
.catch( function( error ) {
console.log(
'Some Error occurred',
JSON.stringify( error )
);
} );
}
}
}), 3000 );
},
onCallConnected: function( component, event ) {
console.log( 'Call Connected' );
},
onCallEnded: function( component, event ) {
console.log( 'Call Ended' );
}
})
Add the Lightning Aura Component to the App’s Utility Items.
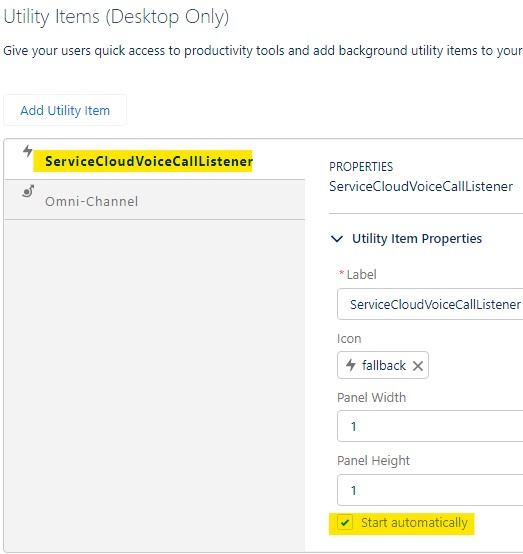
Output:
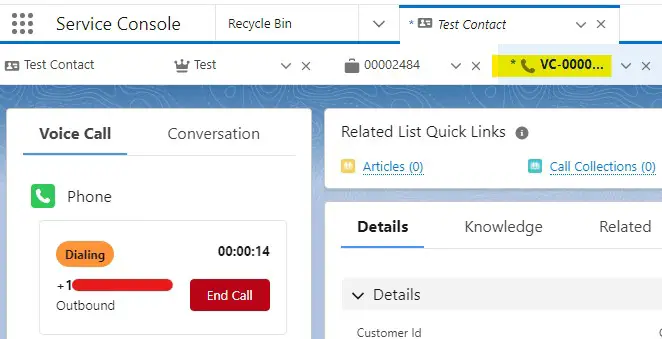