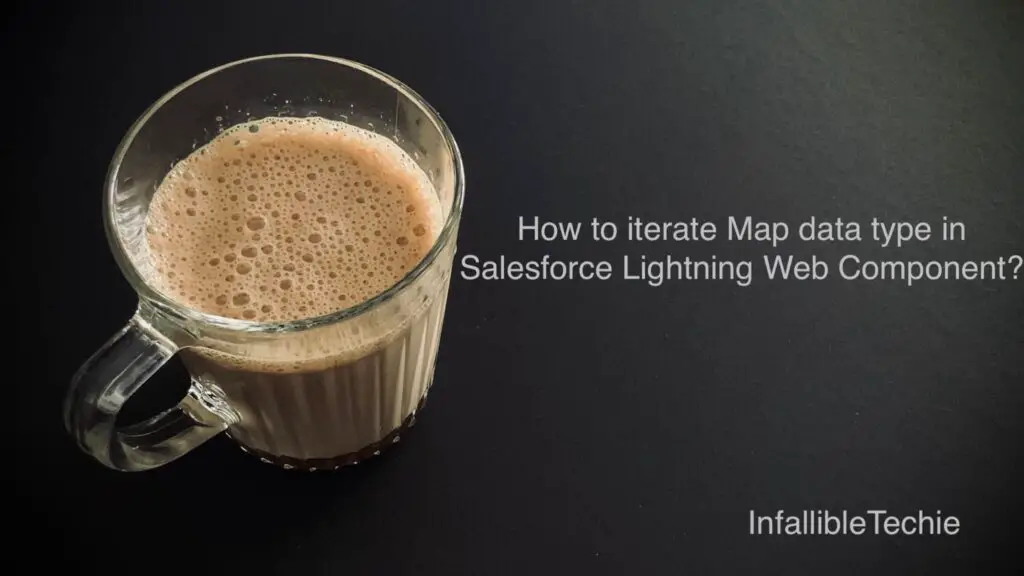
Convert the Map Data Type values returned from the Apex Class to an array to display it on the screen using the Lightning Web Component.
Sample Code:
Apex Controller:
public with sharing class SampleLWCController {
@AuraEnabled( cacheable = true )
public static Map < Id, Account > fetchMapData(){
return new Map < Id, Account > (
[
SELECT Id, Name, Industry
FROM Account
LIMIT 5
]
);
}
}
Lightning Web Component:
HTML:
<template>
<template if:true={mapRecords}>
<lightning-card>
<table class="slds-table slds-table_cell-buffer slds-table_bordered slds-table_col-bordered">
<thead>
<tr>
<th>Name</th>
<th>Industry</th>
</tr>
</thead>
<tbody>
<template for:each={mapRecords} for:item="rec">
<tr key={rec.key}>
<td>
{rec.value.Name}
</td>
<td>
<div>{rec.value.Industry}</div>
</td>
</tr>
</template>
</tbody>
</table>
</lightning-card>
</template>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import fetchMapData from '@salesforce/apex/SampleLWCController.fetchMapData';
export default class sampleLightningWebComponent extends LightningElement {
mapRecords;
error;
@wire( fetchMapData )
wiredMapData( { error, data } ) {
if ( data ) {
for ( let key in data ) {
let tempRec = { value : data[ key ], key : key };
if ( this.mapRecords ) {
this.mapRecords = [ ...this.mapRecords, tempRec ];
} else {
this.mapRecords = [ tempRec ];
}
}
console.log(
'Records are ',
JSON.stringify( this.mapRecords )
);
this.error = undefined;
} else if ( error ) {
console.log(
'Error is ',
JSON.stringify( error )
);
this.error = JSON.stringify( error );
this.records = undefined;
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>57.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
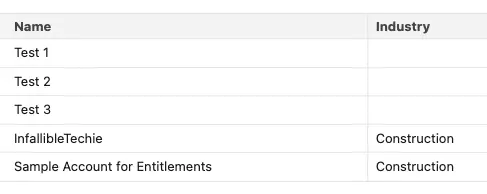