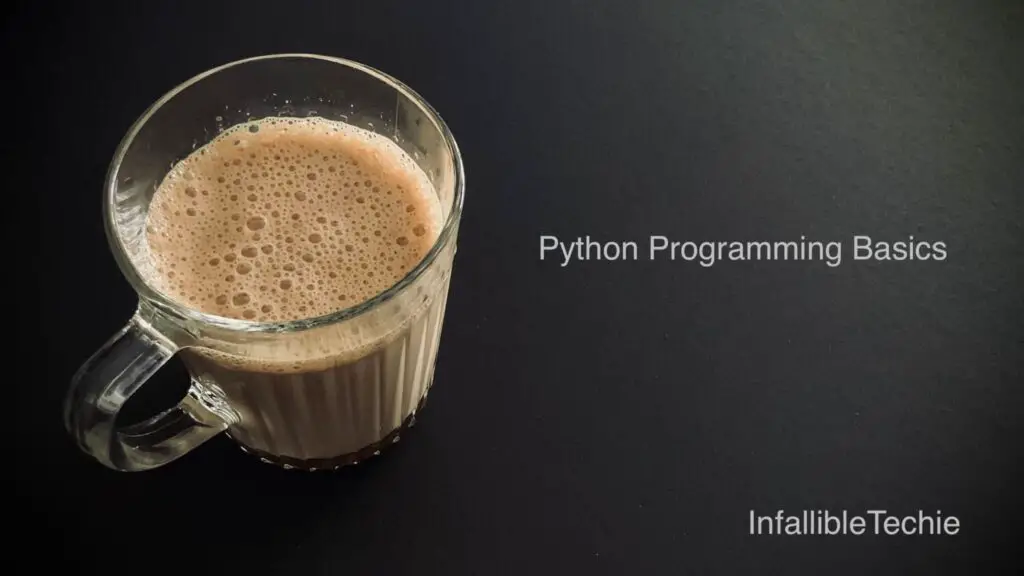
Python Programming Basics are Reserved Keywords, Constants, Variable, type() function, input() function and comments statements.
Reserved Words:
class, return, is, if, while, print, input, global, continue, type, float, int, str, etc are reserved keywords in Python.
Constants:
Constants are fixed values.
Example:
PI = 3.14;
Variable:
Variables are used to declare values and retrieve the data. Variables values can be changed. Variables should start with a letter or underscore.
Example:
a = 100;
print( a );
type() function:
type() function can be used to find the variable or constant type.
Example:
a = 100;
print(
type( a )
);
b = 'test';
print(
type( b )
);
input() function:
input() function can be used to pause the program to get data from the user. input() function always returns a str(string) class object.
Example:
strName = input(
'Please share your name'
);
print(
'Welcome to InfallibleTechie,',
strName + '!!!'
);
Comments Statements:
# is used for Comments in Python.
Example:
#Expecting name value from the user
strName = input(
'Please share your name'
);
#Printing the name with welcome note
print(
'Welcome to InfallibleTechie,',
strName + '!!!'
);