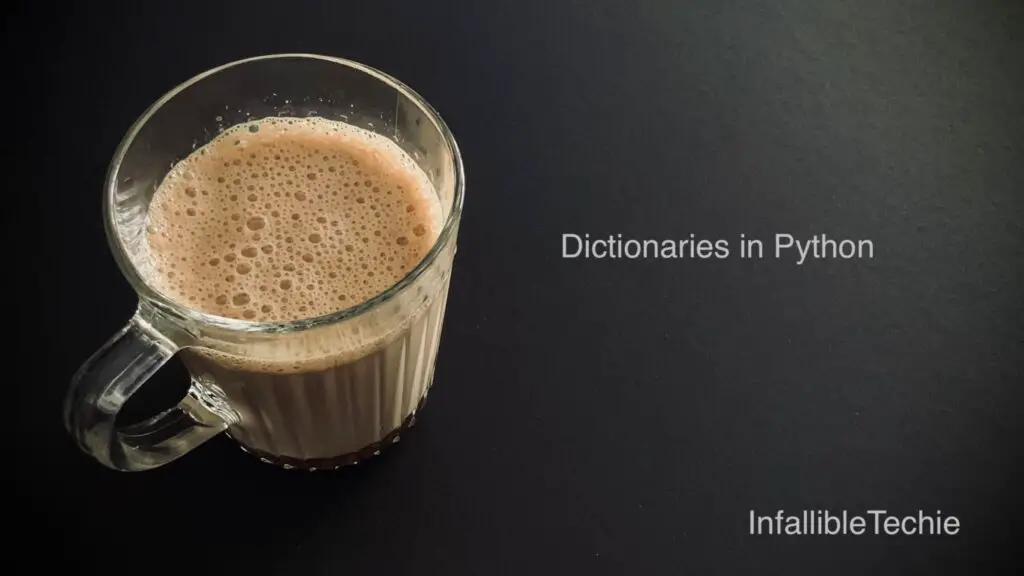
Dictionaries in Python are used for data collection.
They are similar to map data type in other programming languages where we use key and value pairs.
dict():
dict() is used to define the Dictionaries in Python.
Example:
colorsValues = dict();
colorsValues[ 1 ] = 'Red';
colorsValues[ 2 ] = 'Green';
colorsValues[ 3 ] = 'blue';
print(
colorsValues[ 2 ]
);
get():
get() method can be used to find the value by passing the key.
Example:
colorsValues = dict();
colorsValues[ 1 ] = 'Red';
colorsValues[ 2 ] = 'Green';
colorsValues[ 3 ] = 'blue';
print(
colorsValues.get( 2 )
);
How to check whether Dictionary contains a specific key?
in statement can be used to check whether Dictionary contains a specific key.
Example:
colorsValues = dict();
colorsValues[ 1 ] = 'Red';
colorsValues[ 2 ] = 'Green';
colorsValues[ 3 ] = 'blue';
if ( 2 in colorsValues ) :
print(
colorsValues.get( 2 )
);
else :
print( '2 is not available' );
if ( 5 in colorsValues ) :
print(
colorsValues.get( 3 )
);
else :
print( '5 is not available' );