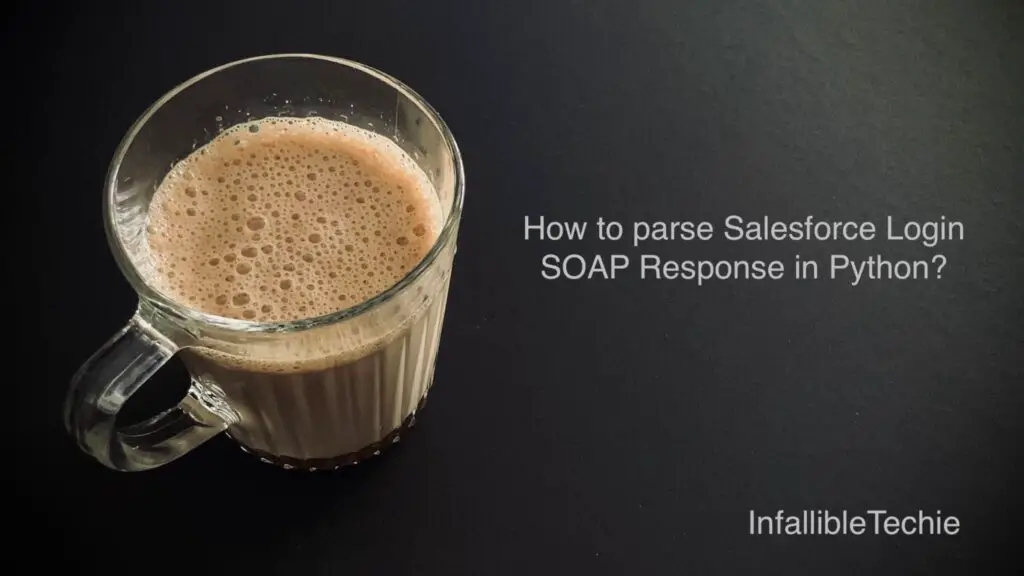
RegEx(Regular Expression) can be used to parse to parse Salesforce Login SOAP Response in Python.
Sample Code:
import re
strXMLString = '''<?xml version="1.0" encoding="UTF-8"?>
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns="urn:enterprise.soap.sforce.com" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<soapenv:Body>
<loginResponse>
<result>
<metadataServerUrl>https://infallibletechie.my.salesforce.com/services/Soap/m/29.0/00Di0000000icUB</metadataServerUrl>
<passwordExpired>false</passwordExpired>
<sandbox>false</sandbox>
<serverUrl>https://infallibletechie.my.salesforce.com/services/Soap/c/29.0/00Di0000000icUB/0DFi00000008UYO</serverUrl>
<sessionId>SampleSessionId</sessionId>
<userId>005i0000002MUqLAAK</userId>
<userInfo>
<accessibilityMode>false</accessibilityMode>
<currencySymbol>$</currencySymbol>
<orgAttachmentFileSizeLimit>5242880</orgAttachmentFileSizeLimit>
<orgDefaultCurrencyIsoCode>USD</orgDefaultCurrencyIsoCode>
<orgDisallowHtmlAttachments>false</orgDisallowHtmlAttachments>
<orgHasPersonAccounts>false</orgHasPersonAccounts>
<organizationId>00Di0000000icUBKDI</organizationId>
<organizationMultiCurrency>false</organizationMultiCurrency>
<organizationName>InfallibleTechie</organizationName>
<profileId>00ei0000001CMKcAAO</profileId>
<roleId xsi:nil="true" />
<sessionSecondsValid>6000</sessionSecondsValid>
<userDefaultCurrencyIsoCode xsi:nil="true" />
<userEmail>[email protected]</userEmail>
<userFullName>TestUser</userFullName>
<userId>005i0000002MUqLAAK</userId>
<userLanguage>en_US</userLanguage>
<userLocale>en_US</userLocale>
<userName>[email protected]</userName>
<userTimeZone>America/Los_Angeles</userTimeZone>
<userType>Standard</userType>
<userUiSkin>Theme3</userUiSkin>
</userInfo>
</result>
</loginResponse>
</soapenv:Body>
</soapenv:Envelope>''';
organizationId = re.findall( "<organizationId>(.*?)</organizationId>", strXMLString )[ 0 ];
print( 'Organization Id is', organizationId );
serverUrl = re.findall( "<serverUrl>(.*?)</serverUrl>", strXMLString )[ 0 ];
print( 'Server URL is', serverUrl );
strURL = re.findall( "(.*?)services/Soap", serverUrl )[ 0 ];
print( 'strURL is', strURL );
Output:
