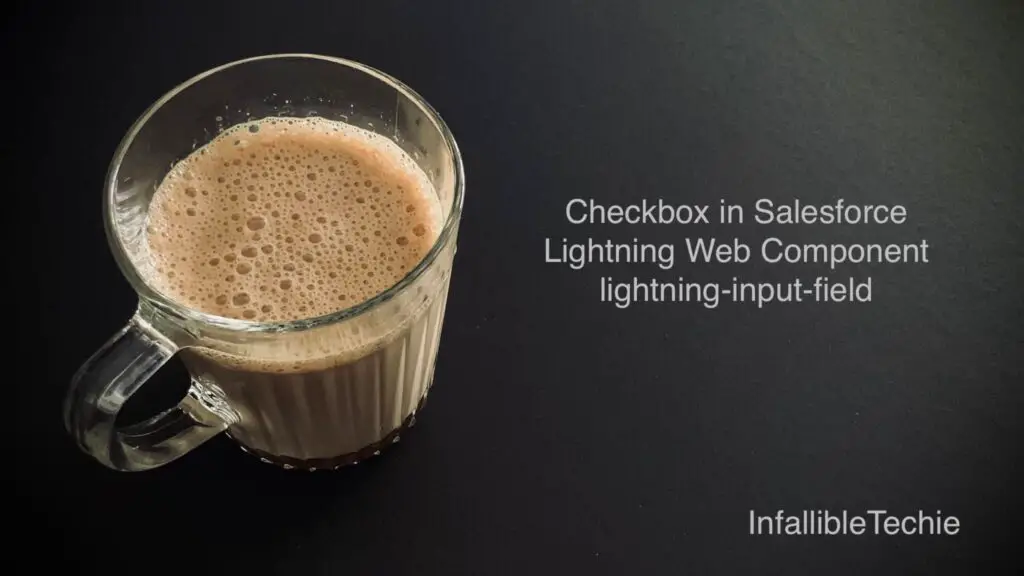
In Salesforce Lightning Web Component lightning-input-field, we can make use of value attribute to pass and set the value.
Sample Checkbox Field:
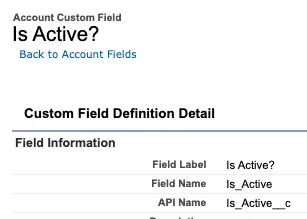
Sample Lightning Web Component:
HTML:
<template>
<lightning-card class="slds-var-p-around_small">
<lightning-record-edit-form
object-api-name="Account"
record-id={recordId}
onsuccess={handleSuccess}
onsubmit={handleSubmit}>
<lightning-messages></lightning-messages>
<lightning-input-field
field-name="Name"
read-only>
</lightning-input-field>
<lightning-input-field
field-name="Is_Active__c"
read-only
value={activeFlag}>
</lightning-input-field>
<template if:true={activeFlag}>
<lightning-button
label="Set Inactive"
class="slds-var-p-around_medium"
onclick={setActiveFlag}>
</lightning-button>
</template>
<template if:false={activeFlag}>
<lightning-button
label="Set Active"
class="slds-var-p-around_medium"
onclick={setActiveFlag}>
</lightning-button>
</template>
<lightning-button
type="submit"
name="submit"
label="Update Account"
class="slds-var-p-around_medium">
</lightning-button>
</lightning-record-edit-form>
</lightning-card>
</template>
JavaScript:
import { LightningElement, api, wire } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { getRecord } from 'lightning/uiRecordApi';
const FIELDS = [ 'Account.Is_Active__c' ];
export default class AccountUpdate extends LightningElement {
@api recordId;
activeFlag;
@wire( getRecord, { recordId: '$recordId', fields: FIELDS } )
wiredRecord({ error, data }) {
if ( error ) {
console.log( 'Inside error block' );
let message = 'Unknown error';
if ( Array.isArray( error.body ) ) {
message = error.body.map(e => e.message).join(', ');
} else if ( typeof error.body.message === 'string' ) {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent({
title: 'Some error occurred',
message,
variant: 'error',
}),
);
} else if ( data ) {
console.log(
'Active Flag Value is',
JSON.stringify( data.fields.Is_Active__c.value )
);
this.activeFlag = data.fields.Is_Active__c.value;
}
}
handleSuccess() {
console.log( 'Inside Handle Success' );
this.dispatchEvent(
new ShowToastEvent( {
title: 'Account Update',
message: 'Account Updated Successfully!!!',
variant: 'success'
} ),
);
}
handleSubmit(event){
event.preventDefault();
const fields = event.detail.fields;
fields.Is_Active__c = this.activeFlag;
this.template.querySelector( 'lightning-record-edit-form' )
.submit( fields );
}
setActiveFlag() {
this.activeFlag = !this.activeFlag;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>57.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
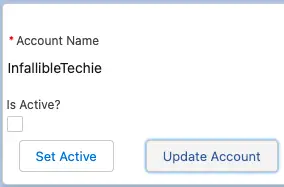