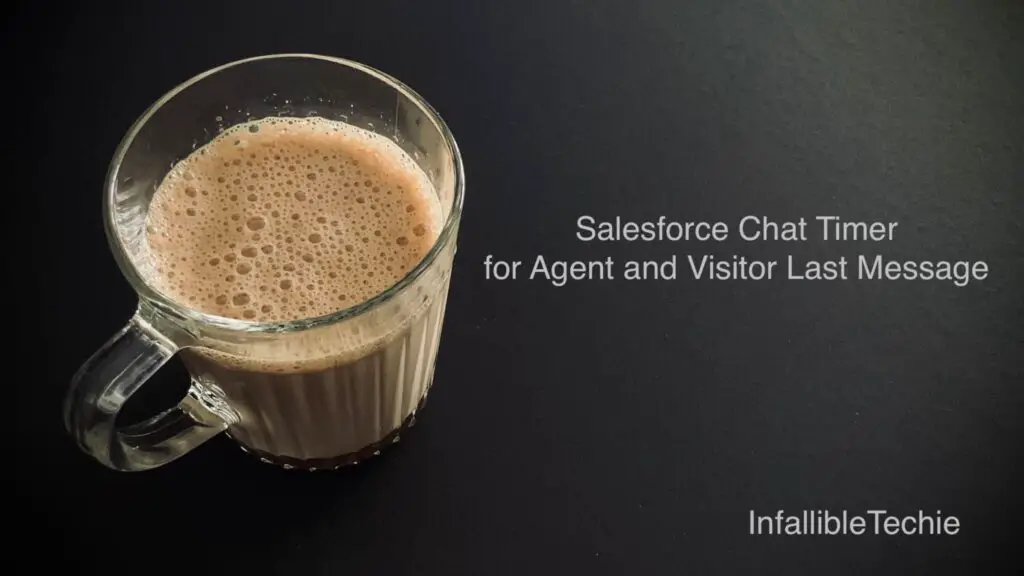
For Salesforce Chat Timer for Agent and Visitor Last Messages, we can make use of Lightning Aura Component. In the Lightning Aura Component, we can make use of lightning:conversationAgentSend, lightning:conversationNewMessage and lightning:conversationChatEnded events to start and reset the Salesforce Chat Timer.
Sample Aura Component:
Component:
<aura:component implements="flexipage:availableForAllPageTypes">
<aura:attribute type="String" name="agentTimer"/>
<aura:attribute type="String" name="visitorTimer"/>
<aura:attribute type="String" name="agentIntervalId"/>
<aura:attribute type="String" name="visitorIntervalId"/>
<aura:handler
event="lightning:conversationAgentSend"
action="{!c.onAgentMessage}"
/>
<aura:handler
event="lightning:conversationNewMessage"
action="{!c.onVisitorMessage}"
/>
<aura:handler
event="lightning:conversationChatEnded"
action="{!c.onChatEnded}"
/>
<lightning:card class="slds-var-p-around_small">
Last Agent Message was before: {!v.agentTimer}
<br/><br/>
Last Vistor Message was before: {!v.visitorTimer}
<br/><br/>
</lightning:card>
</aura:component>
Controller:
({
onAgentMessage : function( component, event, helper ) {
helper.clearAgentTimer( component );
let totalMilliseconds = 0;
let agentIntervalId = window.setInterval(
$A.getCallback(function() {
let totalSeconds = parseInt(
Math.floor( totalMilliseconds / 1000 )
);
let totalMinutes = parseInt(
Math.floor( totalSeconds / 60 ) );
let totalHours = parseInt(
Math.floor( totalMinutes / 60 )
);
let seconds = parseInt(
totalSeconds % 60
);
let minutes = parseInt(
totalMinutes % 60
);
let hours = parseInt(
totalHours % 24
);
let milliseconds = Math.floor(
( totalMilliseconds % ( 1000 ) )
);
let agentTimer = hours + " hours " + minutes + " minutes "
+ seconds + " seconds " + milliseconds + " milliseconds ";
totalMilliseconds += 100;
component.set( "v.agentTimer", agentTimer );
}), 100
);
component.set( "v.agentIntervalId", agentIntervalId );
},
onVisitorMessage : function( component, event, helper ) {
helper.clearVisitorTimer( component );
let totalMilliseconds = 0;
let visitorIntervalId = window.setInterval(
$A.getCallback(function() {
let totalSeconds = parseInt(
Math.floor( totalMilliseconds / 1000 )
);
let totalMinutes = parseInt(
Math.floor( totalSeconds / 60 ) );
let totalHours = parseInt(
Math.floor( totalMinutes / 60 )
);
let seconds = parseInt(
totalSeconds % 60
);
let minutes = parseInt(
totalMinutes % 60
);
let hours = parseInt(
totalHours % 24
);
let milliseconds = Math.floor(
( totalMilliseconds % ( 1000 ) )
);
let visitorTimer = hours + " hours " + minutes + " minutes "
+ seconds + " seconds " + milliseconds + " milliseconds ";
totalMilliseconds += 100;
component.set( "v.visitorTimer", visitorTimer );
}), 100
);
component.set( "v.visitorIntervalId", visitorIntervalId );
},
onChatEnded: function( component, event, helper ) {
console.log( 'Inside End Chat' );
helper.clearAgentTimer( component );
helper.clearVisitorTimer( component );
component.set( "v.visitorTimer", "" );
component.set( "v.agentTimer", "" );
console.log( 'End of End Chat' );
}
})
Helper:
({
clearAgentTimer : function( component ) {
let agentIntervalId = component.get( "v.agentIntervalId" );
if ( agentIntervalId ) {
window.clearInterval( agentIntervalId );
}
},
clearVisitorTimer : function( component ) {
let visitorIntervalId = component.get( "v.visitorIntervalId" );
if ( visitorIntervalId ) {
window.clearInterval( visitorIntervalId );
}
}
})
Output:
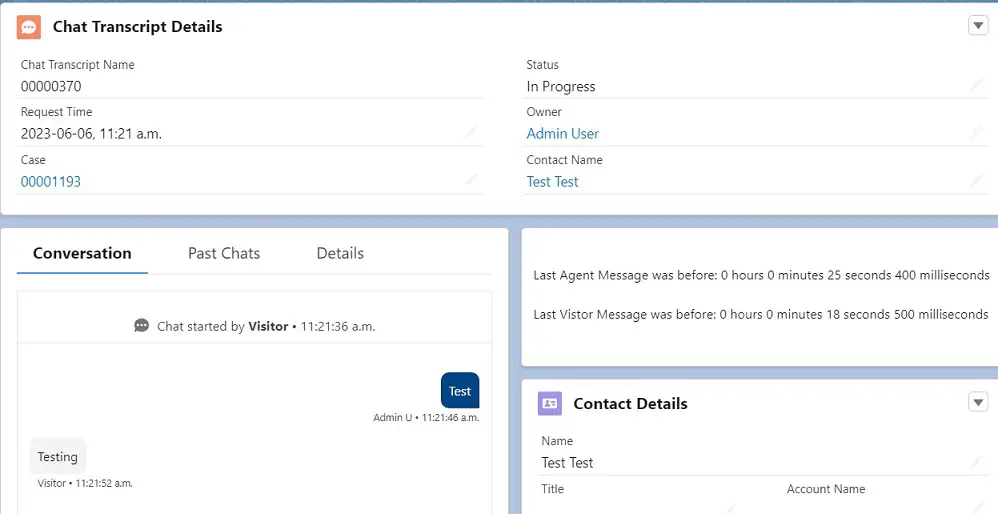
Use the following code if your agents work on multiple chats. The events are fired in all the active chats. So, I have used the record ids from the chat and from the event.
Sample Aura Component:
Component:
<aura:component implements="flexipage:availableForAllPageTypes,force:hasRecordId">
<aura:attribute type="String" name="agentTimer"/>
<aura:attribute type="String" name="visitorTimer"/>
<aura:attribute type="String" name="agentIntervalId"/>
<aura:attribute type="String" name="visitorIntervalId"/>
<aura:handler
event="lightning:conversationAgentSend"
action="{!c.onAgentMessage}"
/>
<aura:handler
event="lightning:conversationNewMessage"
action="{!c.onVisitorMessage}"
/>
<aura:handler
event="lightning:conversationChatEnded"
action="{!c.onChatEnded}"
/>
<lightning:card class="slds-var-p-around_small">
Last Agent Message was before: {!v.agentTimer}
<br/><br/>
Last Vistor Message was before: {!v.visitorTimer}
<br/><br/>
</lightning:card>
</aura:component>
Controller:
({
onAgentMessage : function( component, event, helper ) {
let eventRecordId = event.getParam( "recordId" );
console.log(
'eventRecordId is',
eventRecordId
);
let checkBool = helper.checkRecordId( component, eventRecordId );
console.log(
'checkBool is',
checkBool
);
if ( checkBool ) {
helper.clearAgentTimer( component );
let totalMilliseconds = 0;
let agentIntervalId = window.setInterval(
$A.getCallback(function() {
let totalSeconds = parseInt(
Math.floor( totalMilliseconds / 1000 )
);
let totalMinutes = parseInt(
Math.floor( totalSeconds / 60 ) );
let totalHours = parseInt(
Math.floor( totalMinutes / 60 )
);
let seconds = parseInt(
totalSeconds % 60
);
let minutes = parseInt(
totalMinutes % 60
);
let hours = parseInt(
totalHours % 24
);
let milliseconds = Math.floor(
( totalMilliseconds % ( 1000 ) )
);
let agentTimer = hours + " hours " + minutes + " minutes "
+ seconds + " seconds " + milliseconds + " milliseconds ";
totalMilliseconds += 100;
component.set( "v.agentTimer", agentTimer );
}), 100
);
component.set( "v.agentIntervalId", agentIntervalId );
}
},
onVisitorMessage : function( component, event, helper ) {
let eventRecordId = event.getParam( "recordId" );
console.log(
'eventRecordId is',
eventRecordId
);
let checkBool = helper.checkRecordId( component, eventRecordId );
console.log(
'checkBool is',
checkBool
);
if ( checkBool ) {
helper.clearVisitorTimer( component );
let totalMilliseconds = 0;
let visitorIntervalId = window.setInterval(
$A.getCallback(function() {
let totalSeconds = parseInt(
Math.floor( totalMilliseconds / 1000 )
);
let totalMinutes = parseInt(
Math.floor( totalSeconds / 60 ) );
let totalHours = parseInt(
Math.floor( totalMinutes / 60 )
);
let seconds = parseInt(
totalSeconds % 60
);
let minutes = parseInt(
totalMinutes % 60
);
let hours = parseInt(
totalHours % 24
);
let milliseconds = Math.floor(
( totalMilliseconds % ( 1000 ) )
);
let visitorTimer = hours + " hours " + minutes + " minutes "
+ seconds + " seconds " + milliseconds + " milliseconds ";
totalMilliseconds += 100;
component.set( "v.visitorTimer", visitorTimer );
}), 100
);
component.set( "v.visitorIntervalId", visitorIntervalId );
}
},
onChatEnded: function( component, event, helper ) {
let eventRecordId = event.getParam( "recordId" );
console.log(
'eventRecordId is',
eventRecordId
);
let checkBool = helper.checkRecordId( component, eventRecordId );
console.log(
'checkBool is',
checkBool
);
if ( checkBool ) {
console.log( 'Inside End Chat' );
helper.clearAgentTimer( component );
helper.clearVisitorTimer( component );
component.set( "v.visitorTimer", "" );
component.set( "v.agentTimer", "" );
console.log( 'End of End Chat' );
}
}
})
Helper:
({
clearAgentTimer : function( component ) {
let agentIntervalId = component.get( "v.agentIntervalId" );
if ( agentIntervalId ) {
window.clearInterval( agentIntervalId );
}
},
clearVisitorTimer : function( component ) {
let visitorIntervalId = component.get( "v.visitorIntervalId" );
if ( visitorIntervalId ) {
window.clearInterval( visitorIntervalId );
}
},
checkRecordId : function( component, varRecordId ) {
console.log(
'varRecordId is',
varRecordId
);
let currentRecId = component.get(
"v.recordId"
).substr(0, 15);
console.log(
'currentRecId is',
currentRecId
);
return varRecordId === currentRecId;
}
})