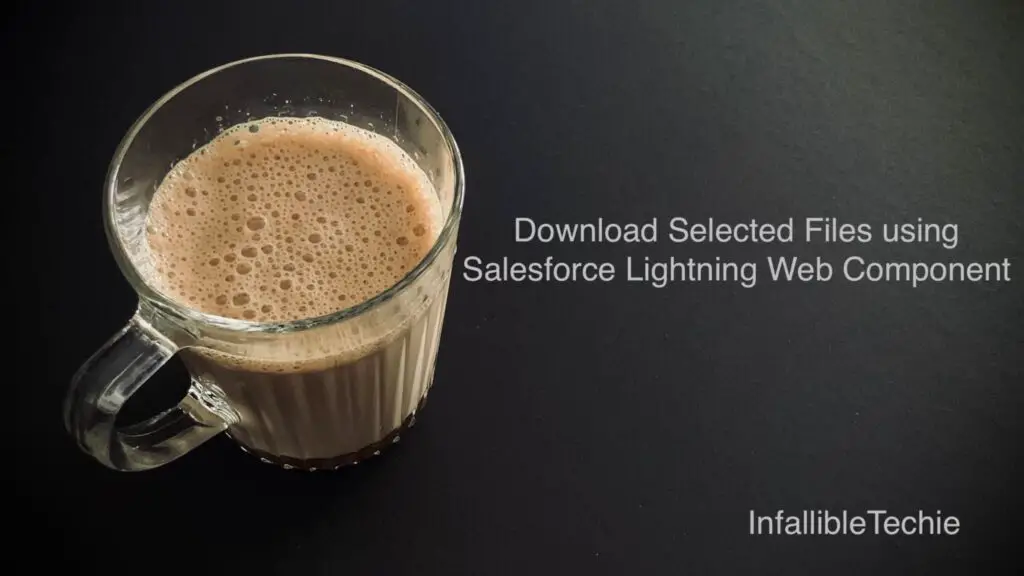
In Salesforce, we can use Screen Action using Lightning Web Component to allow users to select the files to Download Selected Files.
Apex Class:
public with sharing class MassDownloadFilesController {
@AuraEnabled( cacheable = true )
public static List < FilesWrapper > fetchRelatedFiles(
Id strRecordId
) {
List < FilesWrapper > listFilesWrapper = new List < FilesWrapper >();
for ( ContentDocumentLink objCDL: [
SELECT ContentDocument.LatestPublishedVersionId,
ContentDocument.Title
FROM ContentDocumentLink
WHERE LinkedEntityId =: strRecordId
] ) {
listFilesWrapper.add(
new FilesWrapper(
objCDL.ContentDocument.LatestPublishedVersionId,
objCDL.ContentDocument.Title
)
);
}
return listFilesWrapper;
}
public class FilesWrapper {
@AuraEnabled
public Id documentId;
@AuraEnabled
public String documentName;
public FilesWrapper(
Id documentId, String documentName
) {
this.documentId = documentId;
this.documentName = documentName;
}
}
}
Sample Lightning Web Component:
HTML:
<template>
<lightning-quick-action-panel header="Files Download">
<lightning-datatable
key-field="documentId"
data={files}
columns={columns}
onrowselection={handleRowSelection}>
</lightning-datatable>
<div slot="footer">
<lightning-button
variant="brand"
label="Download Selected File(s)"
onclick={downloadFiles}
class="slds-var-p-around_medium">
</lightning-button>
<lightning-button
variant="brand"
label="Close"
onclick={closeQuickActionDialog}
class="slds-var-p-around_medium">
</lightning-button>
</div>
</lightning-quick-action-panel>
</template>
JavaScript:
import { LightningElement, api, wire } from 'lwc';
import { CloseActionScreenEvent } from 'lightning/actions';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import fetchRelatedFiles from '@salesforce/apex/MassDownloadFilesController.fetchRelatedFiles';
import { NavigationMixin } from 'lightning/navigation';
export default class MassDownloadFiles extends NavigationMixin (
LightningElement
) {
@api recordId;
selectedFileIds = [];
files = [];
columns = [
{ label: 'Name', fieldName: 'documentName' },
];
@wire( fetchRelatedFiles, {
strRecordId: '$recordId',
} )
wiredRecord( { error, data } ) {
if ( data ) {
console.log(
'data is',
JSON.stringify( data )
);
if ( data.length > 0 ) {
this.files = data;
} else {
this.dispatchEvent(
new ShowToastEvent({
title: 'File(s) Download',
message: 'Check whether File(s) are attached!!!',
variant: 'error'
}),
);
}
} else if ( error ) {
this.dispatchEvent(
new ShowToastEvent({
title: 'File(s) Download Failed',
message: 'System Error Occurred',
variant: 'error'
}),
);
}
}
handleRowSelection( event ) {
const selectedRows = event.detail.selectedRows;
this.selectedFileIds = [];
for ( let i = 0; i < selectedRows.length; i++ ) {
this.selectedFileIds.push( selectedRows[ i ].documentId );
}
}
downloadFiles(){
let filesDownloadUrl = '/sfc/servlet.shepherd/version/download';
this.selectedFileIds.forEach( item => {
filesDownloadUrl += '/' + item
});
console.log(
'filesDownloadUrl is',
filesDownloadUrl
);
this[ NavigationMixin.Navigate ]( {
type: 'standard__webPage',
attributes: {
url: filesDownloadUrl
}
}, false );
this.dispatchEvent(
new ShowToastEvent( {
title: 'File(s) Download',
message: 'File(s) Downloaded Successfully!!!',
variant: 'success'
} ),
);
/*
Closing the dialog after 3 seconds
*/
setTimeout( () => {
this.closeQuickActionDialog();
}, 3000 );
}
closeQuickActionDialog() {
console.log(
'Inside Close Method'
);
this.dispatchEvent(
new CloseActionScreenEvent()
);
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>ScreenAction</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Create a Quick Action and add it to the page layout.
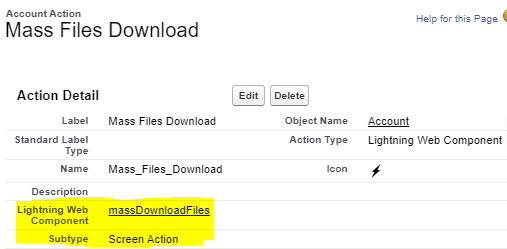
Output:
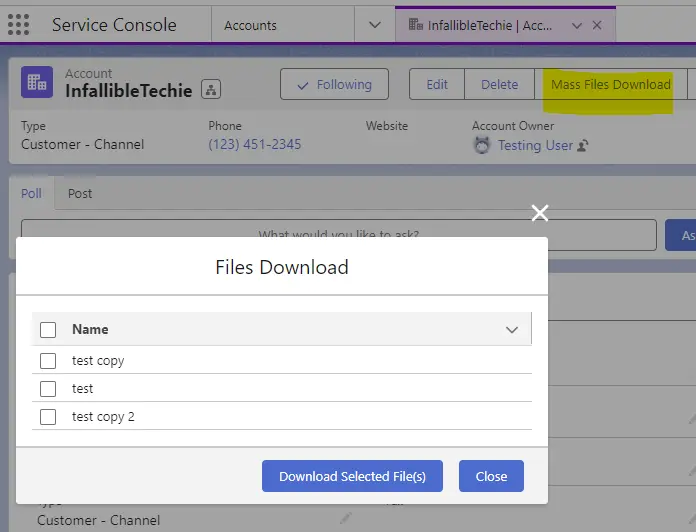