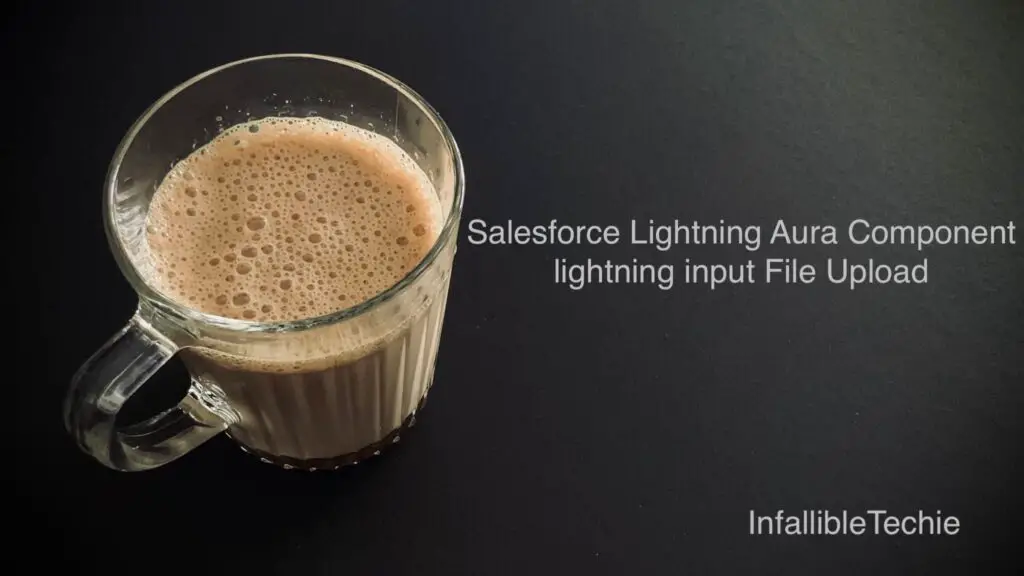
Using Salesforce Apex and FileReader() and encodeURIComponent() from JavaScript, we can easily upload a file using Aura Component lightning:input of type file.
Sample Code:
Apex Class:
public class FileUploadController {
@AuraEnabled
public static String uploadFile(
String filename, String base64Content
) {
ContentVersion objContentVersion = new ContentVersion();
base64Content = EncodingUtil.urlDecode(
base64Content,
'UTF-8'
);
objContentVersion.VersionData = EncodingUtil.base64Decode(
base64Content
);
objContentVersion.Title = filename;
objContentVersion.PathOnClient = filename;
try {
insert objContentVersion;
} catch( DMLException e ) {
System.debug(
'Error while uploading the file ' +
e.getMessage()
);
return e.getMessage();
}
ContentDocumentLink objContentDocumentLink = new ContentDocumentLink();
objContentDocumentLink.ContentDocumentId = [
SELECT ContentDocumentId
FROM ContentVersion
WHERE Id =: objContentVersion.Id
].ContentDocumentId;
objContentDocumentLink.ShareType = 'V';
try {
insert objContentDocumentLink;
} catch( DMLException e ) {
System.debug(
'Error while uploading the file ' +
e.getMessage()
);
return e.getMessage();
}
return 'File Uploaded Successfully';
}
}
Lightning Aura Component:
Component:
<aura:component
implements="force:appHostable"
controller="FileUploadController">
<aura:attribute name="fileName" type="String"/>
<lightning:card class="slds-p-around_x-small" title="Upload Section">
<lightning:input
label="Upload File/Attachment/Document"
type="file"
onchange="{!c.handleFileUpload}"
multiple="false"
aura:id="fileId"/>
{!v.fileName}
<aura:set attribute="footer">
<lightning:button
label="Upload"
onclick="{!c.submitFile}"/>
</aura:set>
</lightning:card>
</aura:component>
JavaScript Controller:
( {
handleFileUpload : function( component, event, helper ) {
let selectedFiles = component.find(
"fileId"
).get(
"v.files"
);
let selectedFile = selectedFiles[ 0 ];
console.log(
'File Name is',
selectedFile.name
);
component.set(
"v.fileName",
selectedFile.name
);
console.log(
'File Size is',
selectedFile.size
);
},
submitFile : function( component, event, helper ) {
console.log(
'Inside the Submit File'
);
let selectedFiles = component.find(
"fileId"
).get(
"v.files"
);
let selectedFile = selectedFiles[ 0 ];
let objFileReader = new FileReader();
objFileReader.onload = $A.getCallback( function() {
let fileContent = objFileReader.result;
let base64 = 'base64,';
let dataStart = fileContent.indexOf( base64 ) + base64.length;
fileContent = fileContent.substring( dataStart );
helper.processFileUpload( component, fileContent, selectedFile );
} );
objFileReader.readAsDataURL(
selectedFile
);
}
} )
JavaScript Helper:
({
processFileUpload : function( component, fileContent, selectedFile ) {
console.log(
'Inside the Process File'
);
console.log(
'File Name is',
selectedFile.name
);
console.log(
'Encoded value is',
encodeURIComponent( fileContent )
);
let action = component.get( "c.uploadFile" );
console.log(
'Action for Upload File called'
);
action.setParams( {
filename: selectedFile.name,
base64Content: encodeURIComponent( fileContent )
} );
action.setCallback( this, function( response ) {
let state = response.getState();
console.log(
'State is',
state
);
console.log(
'Return Value is',
response.getReturnValue()
);
if ( state === "SUCCESS" ) {
console.log(
'Success'
);
component.set(
"v.fileName",
"File Successfully Uploaded"
);
} else {
console.log(
'Failed'
);
component.set(
"v.fileName",
"File Upload Failed. Please try again."
);
}
} );
$A.enqueueAction( action );
}
})
Output:
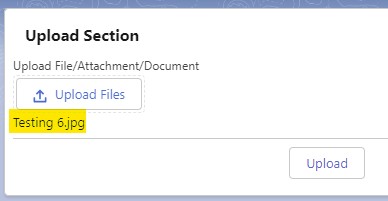
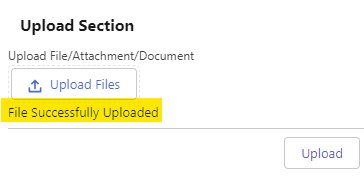
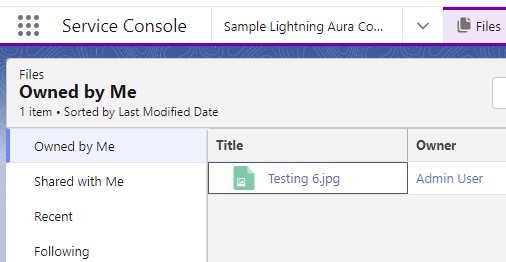