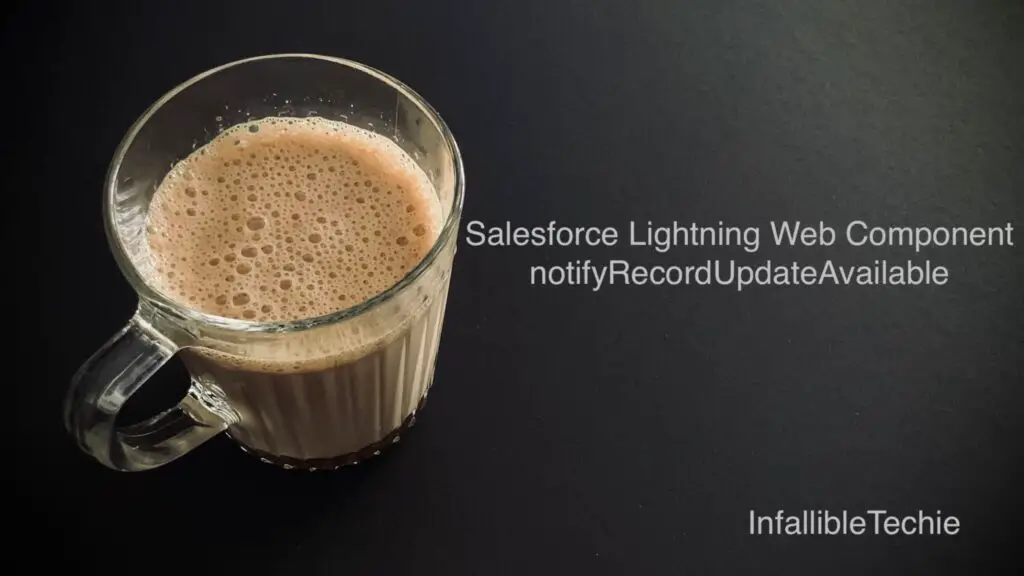
notifyRecordUpdateAvailable in Salesforce Lightning Web Component is used to notify the Lightning Data Service that the record data has modified/changed on the server so that the Lightning Data Service can take the appropriate actions to keep wire adapters updated with the latest record data.
Sample Code:
Apex Class:
public class AccountController {
@AuraEnabled
public static void updateAccount( String strRecordId ) {
update new Account(
Id = strRecordId,
Description = String.valueOf(
System.now()
)
);
}
}
Sample Lightning Web Component:
HTML:
<template>
<lightning-card>
<template if:true={isLoaded}>
<lightning-spinner
alternative-text="Loading..."
variant="brand"
></lightning-spinner>
</template>
<div class="slds-var-p-around_medium">
Name is {name}
<br/><br/>
Description is {description}
<br/><br/><br/>
<lightning-button
label="Update Account"
onclick={updateAccountRecord}
></lightning-button>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire, api } from 'lwc';
import {
getRecord,
notifyRecordUpdateAvailable,
getFieldValue
} from 'lightning/uiRecordApi';
import updateAccount from '@salesforce/apex/AccountController.updateAccount';
import ACCOUNT_NAME from "@salesforce/schema/Account.Name";
import ACCOUNT_DESCRIPTION from "@salesforce/schema/Account.Description";
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class AccountUpdate extends LightningElement {
@api recordId;
name;
description;
isLoaded = false;
@wire( getRecord, {
recordId: '$recordId',
fields: [ ACCOUNT_NAME, ACCOUNT_DESCRIPTION ]
} )
wiredRecord( { error, data } ) {
if ( error ) {
console.log(
'Inside error block'
);
let message = 'Unknown error';
if ( Array.isArray( error.body ) ) {
message = error.body.map(e => e.message).join(', ');
} else if ( typeof error.body.message === 'string' ) {
message = error.body.message;
}
this.dispatchEvent(
new ShowToastEvent( {
title: 'Some error occurred',
message,
variant: 'error',
} ),
);
} else if ( data ) {
console.log(
'Data is',
JSON.stringify(
data
)
);
this.name = getFieldValue(
data,
ACCOUNT_NAME
);
this.description = getFieldValue(
data,
ACCOUNT_DESCRIPTION
);
}
}
async updateAccountRecord() {
this.isLoaded = true;
await updateAccount(
{ strRecordId : this.recordId }
);
await notifyRecordUpdateAvailable(
[ { recordId: this.recordId } ]
);
this.isLoaded = false;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<supportedFormFactors>
<supportedFormFactor type="Large" />
<supportedFormFactor type="Small" />
</supportedFormFactors>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Output:
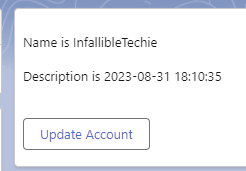