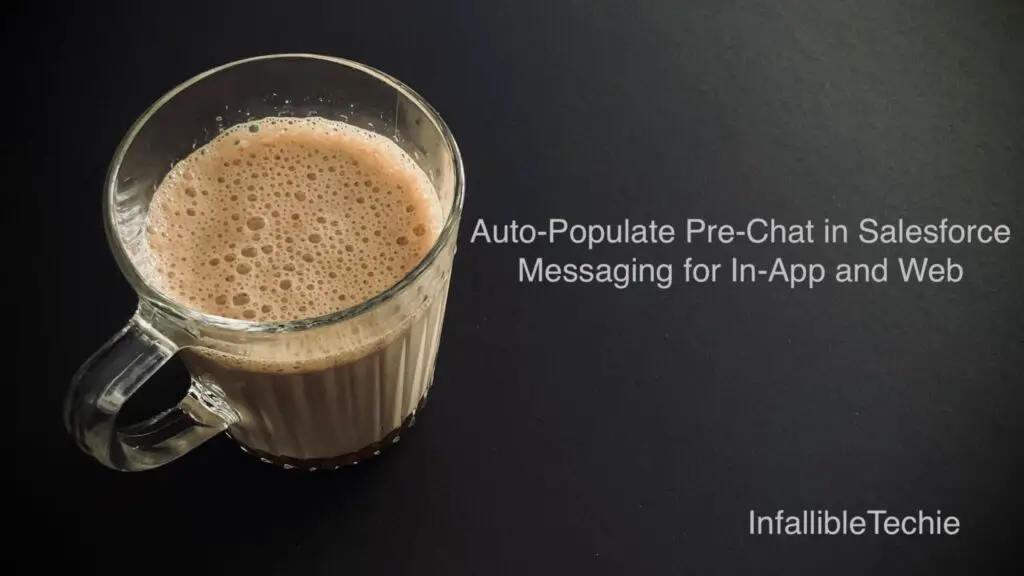
To Auto-Populate Pre-Chat in Salesforce Messaging for In-App and Web, we can make use of Lightning Web Component.
Pre-Chat Form in the Embedded Service Deployment for Messaging for In-App and Web, should be overridden with the Lightning Web Component.
Lightning Web Component:
Child Component:
HTML:
<template>
<template lwc:if={isTypeChoiceList}>
<lightning-combobox
key={fieldInfo.name}
label={fieldInfo.labels.display}
options={choiceListOptions}
value={choiceListDefaultValue}
required={fieldInfo.required}>
</lightning-combobox>
</template>
<template lwc:else>
<lightning-input
key={fieldInfo.name}
type={type}
label={fieldInfo.labels.display}
max-length={fieldInfo.maxLength}
required={fieldInfo.required}
value={fieldInfo.value}>
</lightning-input>
</template>
</template>
JavaScript:
import { api, LightningElement } from "lwc";
export default class CustomPreChatFormField extends LightningElement {
choiceListDefaultValue;
@api fieldInfo = {};
@api
get name() {
return this.fieldInfo.name;
}
@api
get value() {
const lightningCmp =
this.isTypeChoiceList ?
this.template.querySelector( "lightning-combobox" ):
this.template.querySelector( "lightning-input" );
return this.isTypeCheckbox ?
lightningCmp.checked :
lightningCmp.value;
}
@api
reportValidity() {
const lightningCmp
= this.isTypeChoiceList ?
this.template.querySelector("lightning-combobox") :
this.template.querySelector("lightning-input");
return lightningCmp.reportValidity();
}
get type() {
switch (this.fieldInfo.type) {
case "Phone":
return "tel";
case "Text":
case "Email":
case "Number":
case "Checkbox":
case "ChoiceList":
return this.fieldInfo.type.toLowerCase();
default:
return "text";
}
}
get isTypeCheckbox() {
return this.type === "Checkbox".toLowerCase();
}
get isTypeChoiceList() {
return this.type === "ChoiceList".toLowerCase();
}
get choiceListOptions() {
let choiceListOptions = [];
const choiceListValues = [ ...this.fieldInfo.choiceListValues ];
choiceListValues.sort(
(valueA, valueB) => valueA.order - valueB.order
);
for ( const listValue of choiceListValues ) {
if ( listValue.isDefaultValue ) {
this.choiceListDefaultValue =
listValue.choiceListValueName;
}
choiceListOptions.push(
{
label: listValue.label,
value: listValue.choiceListValueName
}
);
}
console.log(
'choiceListDefaultValue is',
this.choiceListDefaultValue
);
if ( !this.choiceListDefaultValue ) {
console.log(
'Default value is set since isDefaultValue is not set'
);
this.choiceListDefaultValue = 'High';
}
return choiceListOptions;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>58.0</apiVersion>
<isExposed>true</isExposed>
</LightningComponentBundle>
Parent Component:
HTML:
<template>
<template
class="slds-m-around_medium"
for:each={fields}
for:item="field">
<c-custom-pre-chat-form-field
key={field.name}
field-info={field}>
</c-custom-pre-chat-form-field>
</template>
<lightning-button
label="Start Chat"
title={startConversationLabel}
onclick={onStartConversationClick}
class="slds-m-left_x-small">
</lightning-button>
</template>
JavaScript:
import { api, LightningElement } from "lwc";
export default class CustomPreChatForm extends LightningElement {
@api configuration = {};
get prechatForm() {
const forms = this.configuration.forms || [];
return forms.find(
form => form.formType === "PreChat"
) || {};
}
get prechatFormFields() {
return this.prechatForm.formFields || [];
}
get fields() {
let fields = JSON.parse(
JSON.stringify(
this.prechatFormFields
)
);
this.handleFields(
fields
);
return fields.sort(
( fieldA, fieldB ) => fieldA.order - fieldB.order
);
}
handleFields( fields ) {
for ( let field of fields ) {
if ( field.name === "_firstName" ) {
field.value = "Test 1";
} else if ( field.name === "_lastName" ) {
field.value = "Test 2";
} else if ( field.name === "_email" ) {
field.value = "[email protected]";
} else if ( field.name === "_subject" ) {
field.value = "Testing";
}
if ( field.type === "ChoiceList" ) {
const valueList =
this.configuration.choiceListConfig.choiceList.find(
list => list.choiceListId === field.choiceListId
) || {};
field.choiceListValues = valueList.choiceListValues || [];
}
}
}
isValid() {
let isFormValid = true;
this.template.querySelectorAll(
"c-custom-pre-chat-form-field"
).forEach( formField => {
if ( !formField.reportValidity() ) {
isFormValid = false;
}
} );
return isFormValid;
}
onStartConversationClick() {
const prechatData = {};
if ( this.isValid() ) {
this.template.querySelectorAll(
"c-custom-pre-chat-form-field"
).forEach( formField => {
prechatData[ formField.name ] = String(
formField.value
);
});
this.dispatchEvent(
new CustomEvent(
"prechatsubmit",
{
detail: { value: prechatData }
}
)
);
}
}
}
CSS:
:host {
display: flex;
flex-direction: column;
flex: 1 1 auto;
overflow: hidden;
background: #FFFFFF;
padding: 2em;
}
lightning-button {
padding-top: 2em;
text-align: center;
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningSnapin__MessagingPreChat</target>
</targets>
</LightningComponentBundle>
Embedded Service Deployment Pre-Chat Configuration:
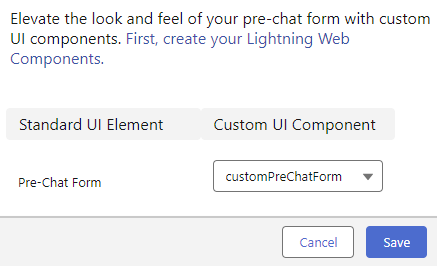
Output:
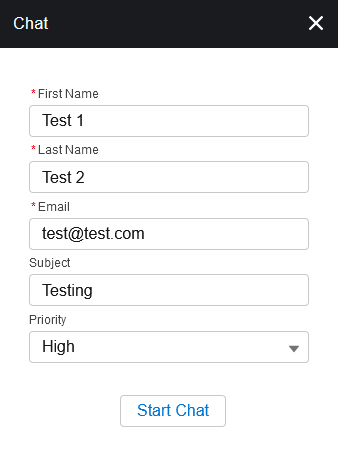