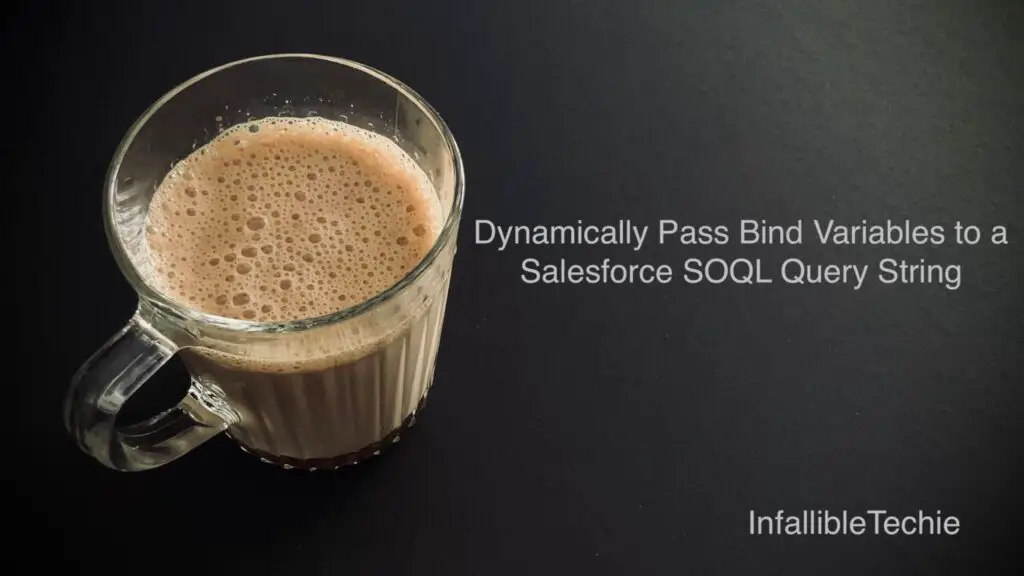
Database.queryWithBinds() in Salesforce Apex allows us to dynamically pass bind variables values to a Salesforce SOQL Query string. Map < String, Object > datatype reduces the number of parameters declaration in the method.
Sample Code:
public static List < Contact > fetchContacts(
Map < String, Object > bindParams
) {
String queryString =
'SELECT Id, Name, Email ' +
'FROM Contact ' +
'WHERE FirstName = :FirstName ' +
'AND LastName = :LastName ' +
'AND Email = :Email ';
return Database.queryWithBinds(
queryString,
bindParams,
AccessLevel.USER_MODE
);
}
insert new Contact(
FirstName = 'Test 1',
LastName = 'Test 2',
Email = '[email protected]'
);
Map < String, Object > contactBind = new Map < String, Object > {
'FirstName' => 'Test 1',
'LastName' => 'Test 2',
'Email' => '[email protected]'
};
List < Contact > listContacts =
fetchContacts( contactBind );
System.debug(
listContacts
);