Refs in Salesforce Lightning Web Component allows us to locate DOM elements without a selector querySelector. This will be very helpful when the querySelector has to iterate multiple elements.
Sample Lightning Web Component:
HTML:
<template>
<lightning-card>
<div class="slds-m-around_medium">
<div lwc:ref="divRef">
Test Div
</div>
<br/>
<lightning-input
label="Name"
type="text"
lwc:ref="inputTextRef">
</lightning-input>
<br/>
<lightning-input
label="Date"
type="date"
lwc:ref="inputDateRef">
</lightning-input>
<br/>
<lightning-button
label="Capture Details"
onclick={captureDetails}>
</lightning-button>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
export default class SampleLightningWebComponent extends LightningElement {
captureDetails() {
console.log(
'Div value is',
this.refs.divRef.innerHTML
);
console.log(
'Input Text value is',
this.refs.inputTextRef.value
);
console.log(
'Input Date value is',
this.refs.inputDateRef.value
);
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
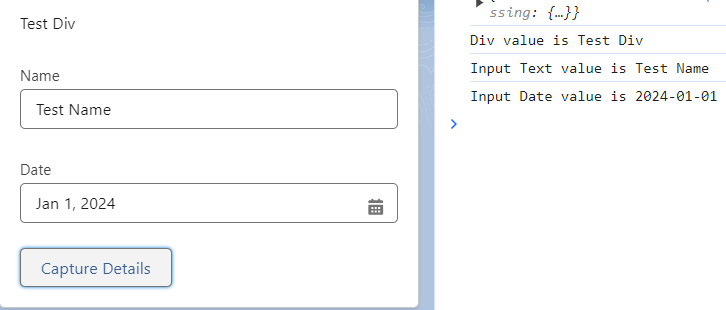