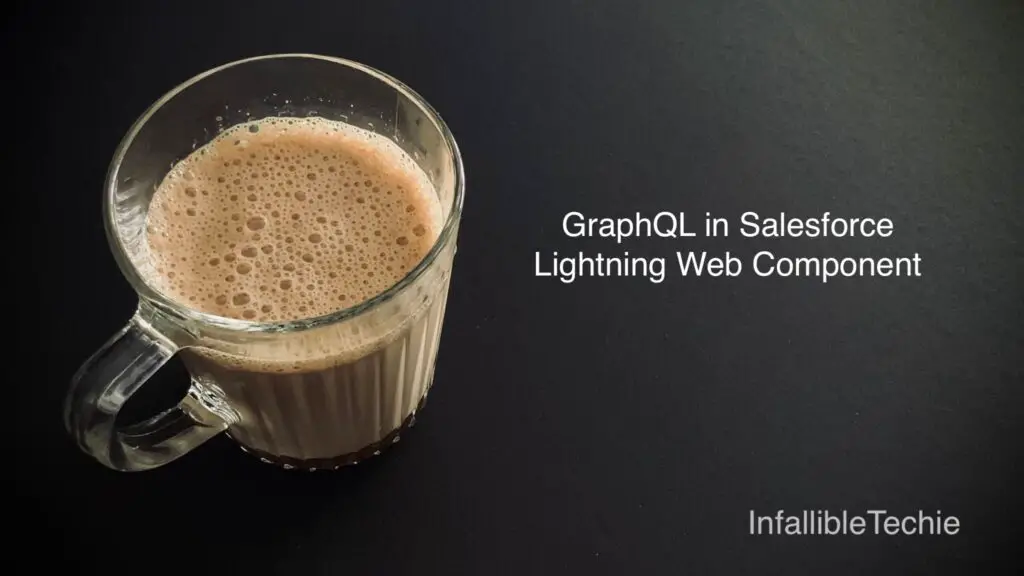
The GraphQL wire adapter can be used in the Salesforce Lightning Web Component.
Sample Lightning Web Component:
HTML:
<template>
<lightning-card>
<div class="slds-m-around_medium">
<lightning-datatable
key-field="Id"
data={accountRecords}
columns={columns}
hide-checkbox-column>
</lightning-datatable>
</div>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import { gql, graphql } from 'lightning/uiGraphQLApi';
export default class SampleLightningWebComponent extends LightningElement {
columns = [
{ label: 'Id', fieldName: 'Id' },
{ label: 'Name', fieldName: 'Name' },
{ label: 'Active', fieldName: 'Active__c' },
{ label: 'Industry', fieldName: 'Industry' },
{ label: 'Type', fieldName: 'Type' }
];
@wire(graphql, {
query: gql`
query getAccounts {
uiapi {
query {
Account(
where: {
Active__c : {
eq : 'Yes'
}
}
first: 15
orderBy: {
Name: {
order: ASC
}
}
) {
edges {
node {
Id
Name {
value
}
Type {
value
}
Active__c : Active__c {
value
}
Industry {
value
}
}
}
}
}
}
}
`
})
graphql;
get accountRecords() {
return this.graphql.data?.uiapi.query.Account.edges.map(
( edge ) => ( {
Id: edge.node.Id,
Name: edge.node.Name.value,
Type: edge.node.Type.value,
Active__c: edge.node.Active__c.value,
Industry: edge.node.Industry.value
}
));
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>59.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
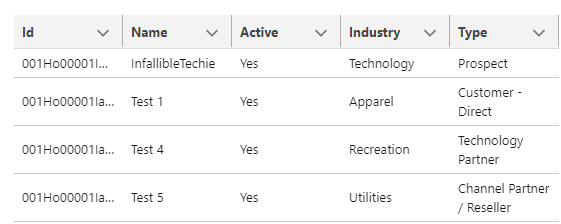