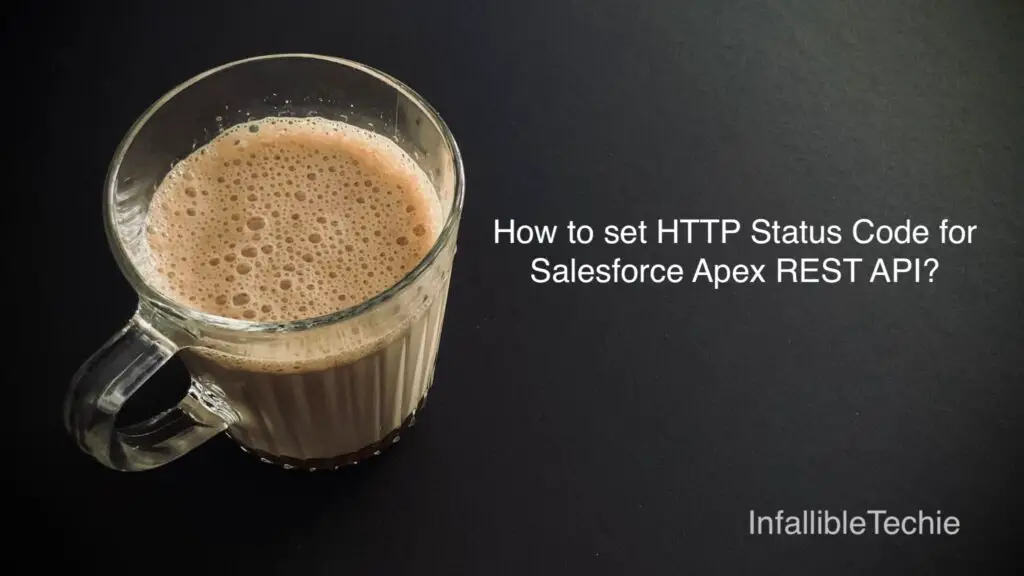
RestContext.response.statusCode can be used to set HTTP Status Code for Salesforce Apex REST API.
Sample Apex Code:
@RestResource( urlMapping='/RecordFinder/V1/*' )
global with sharing class RESTAPIController {
@HttpGet
global static void doGet() {
switch on RestContext.request.requestURI.substringAfterLast( '/' ) {
when 'CheckAccountAvailability' {
verifyExistingAccount();
} when 'CheckContactAvailability' {
verifyExistingContact();
} when else {
String strResponse;
Map < String, String > mapResponse = new Map < String, String >();
mapResponse.put(
'errorMessage',
'Please check the endpoint URL and Path'
);
strResponse = JSON.serialize(
mapResponse
);
RestContext.response.addHeader(
'Content-Type',
'application/json'
);
RestContext.response.responseBody = Blob.valueOf(
strResponse
);
RestContext.response.statusCode = 406;
}
}
}
static void verifyExistingAccount() {
RestRequest req = RestContext.request;
String strResponse;
Map < String, String > mapResponse = new Map < String, String >();
String strAcctName = req.params.containsKey( 'AccountName' ) ?
req.params.get( 'AccountName' ) :
null;
if ( String.isNotBlank( strAcctName ) ) {
List < Account > listAccounts = [
SELECT Id, Name, Industry
FROM Account
WHERE Name =: strAcctName
];
if ( listAccounts.size() > 0 ) {
Account objAccount = listAccounts.get( 0 );
mapResponse.put(
'accountIndustry',
objAccount.Industry
);
mapResponse.put(
'accountName',
objAccount.Name
);
mapResponse.put(
'accountId',
objAccount.Id
);
System.debug(
'Response is ' +
JSON.serialize(
mapResponse
)
);
} else {
mapResponse.put(
'errorMessage',
'Account for this Name is not available'
);
}
} else {
mapResponse.put(
'errorMessage',
'Please pass Account Name to search'
);
}
strResponse = JSON.serialize(
mapResponse
);
RestContext.response.addHeader(
'Content-Type',
'application/json'
);
RestContext.response.responseBody = Blob.valueOf(
strResponse
);
}
static void verifyExistingContact() {
RestRequest req = RestContext.request;
String strResponse;
Map < String, String > mapResponse = new Map < String, String >();
String strEmail = req.params.containsKey( 'ContactEmail' ) ?
req.params.get( 'ContactEmail' ) :
null;
if ( String.isNotBlank( strEmail ) ) {
List < Contact > listContacts = [
SELECT Id, Account.Name, Email, AccountId,
FirstName, LastName
FROM Contact
WHERE Email =: strEmail
];
if ( listContacts.size() > 0 ) {
Contact objContact = listContacts.get( 0 );
mapResponse.put(
'email',
objContact.Email
);
mapResponse.put(
'contactId',
objContact.Id
);
mapResponse.put(
'contactLastName',
objContact.LastName
);
mapResponse.put(
'contactFirstName',
objContact.FirstName
);
mapResponse.put(
'accountId',
objContact.AccountId
);
mapResponse.put(
'accountName',
objContact.Account.Name
);
} else {
mapResponse.put(
'errorMessage',
'Contact for this email is not available'
);
}
} else {
mapResponse.put(
'errorMessage',
'Please pass Email to search'
);
}
strResponse = JSON.serialize(
mapResponse
);
RestContext.response.addHeader(
'Content-Type',
'application/json'
);
RestContext.response.responseBody = Blob.valueOf(
strResponse
);
}
}
Output:
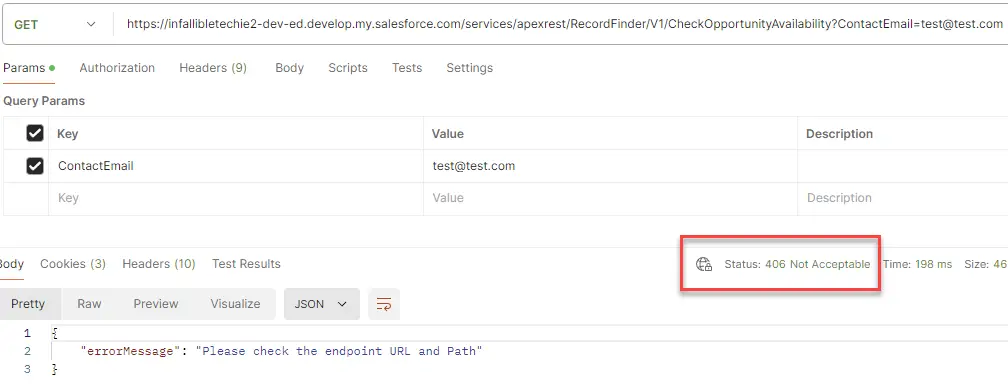