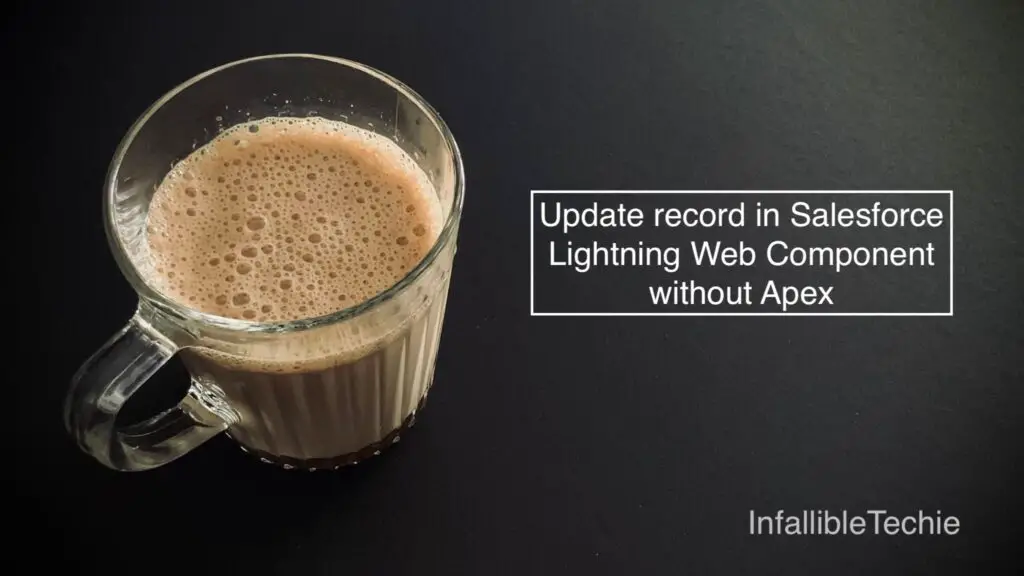
updateRecord from the ‘lightning/uiRecordApi’ library can be used to update records without using Apex in Salesforce Lightning Web Component.
Sample Lightning Web Component:
HTML:
<template>
<lightning-card icon-name="standard:case">
<p class="slds-p-horizontal_small">
<lightning-input
type="text"
label="Subject"
placeholder="Enter new Subject"
onchange={handleInputChange}
></lightning-input>
<lightning-input
type="text"
label="Description"
placeholder="Enter new Description"
onchange={handleInputChange}
></lightning-input>
<lightning-input
type="text"
label="Comments"
placeholder="Enter Case Comment"
onchange={handleInputChange}
></lightning-input>
</p>
<p slot="footer">
<lightning-button
label="Update Case"
onclick={updateCaseRecord}
variant="brand"
icon-name="standard:record_update"
></lightning-button>
</p>
</lightning-card>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import { updateRecord } from 'lightning/uiRecordApi';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { RefreshEvent } from "lightning/refresh";
import DESCRIPTION_FIELD from '@salesforce/schema/Case.Description';
import SUBJECT_FIELD from '@salesforce/schema/Case.Subject';
import COMMENTS_FIELD from '@salesforce/schema/Case.Comments';
import ID_FIELD from '@salesforce/schema/Case.Id';
export default class QuickCaseUpdate extends LightningElement {
@api recordId;
subject;
description;
comments;
handleInputChange( event ) {
const label = event.target.label;
if ( label == 'Subject' ) {
this.subject = event.detail.value;
}
if ( label == 'Description' ) {
this.description = event.detail.value;
}
if ( label == 'Comments' ) {
this.comments = event.detail.value;
}
}
updateCaseRecord() {
const fields = {};
if (
this.description &&
this.description.trim().length > 0
) {
fields[ DESCRIPTION_FIELD.fieldApiName] = this.description;
}
if (
this.subject &&
this.subject.trim().length > 0
) {
fields[ SUBJECT_FIELD.fieldApiName] = this.subject;
}
if (
this.comments &&
this.comments.trim().length > 0
) {
fields[ COMMENTS_FIELD.fieldApiName] = this.comments;
}
if (
Object.keys( fields ).length > 0
) {
fields[ ID_FIELD.fieldApiName ] = this.recordId;
const recordInput = { fields };
updateRecord( recordInput )
.then( () => {
this.dispatchEvent(
new RefreshEvent()
);
this.dispatchEvent(
new ShowToastEvent( {
title: 'Success',
message: 'Case updated',
variant: 'success'
} )
);
} ).catch( error => {
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error updating Case',
message: error.body.message,
variant: 'error'
} )
);
} );
} else {
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error updating Case',
message: 'Please enter Case details',
variant: 'error'
} )
);
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>61.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
Output:
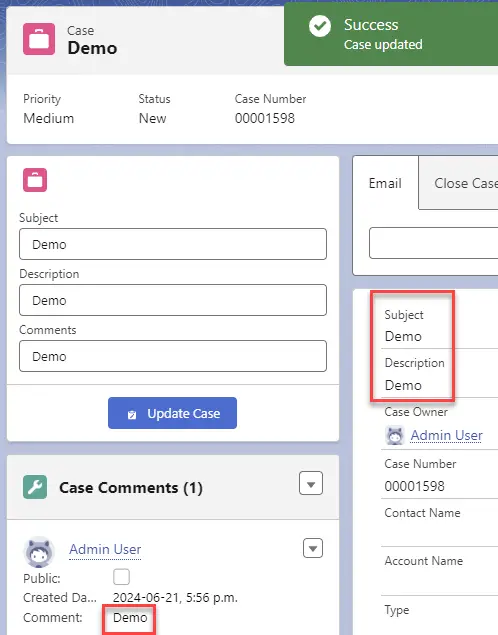