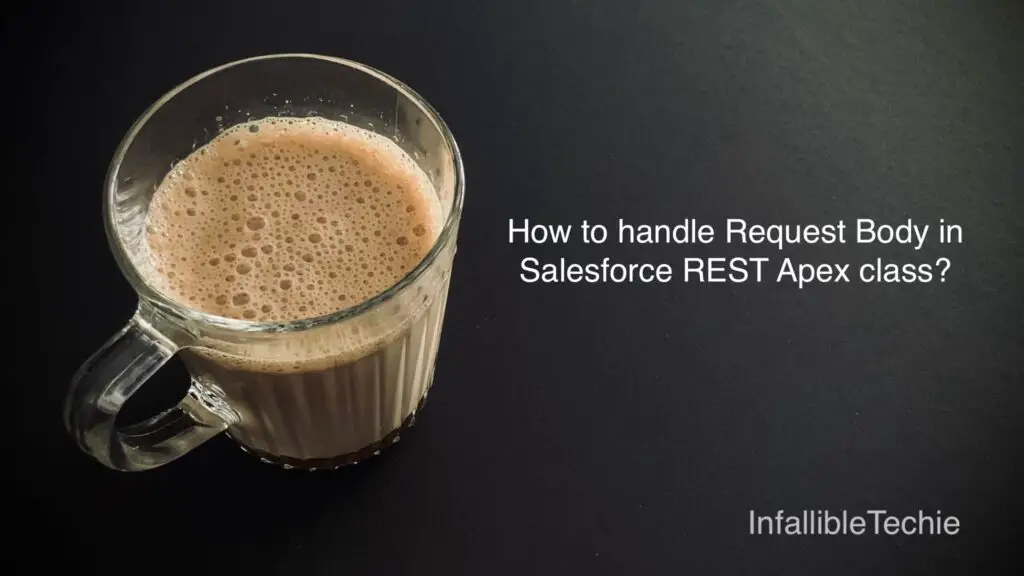
RestContext.request.requestBody contains the Request Body in Salesforce REST API Apex Class. So, we can get the RestContext.request.requestBody to handle Request Body in Salesforce REST API Apex class.
Sample Apex Code:
@RestResource( urlMapping='/AccountCreation/' )
global with sharing class RESTAPIController {
@HttpPost
global static void createAccount() {
String strResponse;
Map < String, String > mapResponse = new Map < String, String >();
RestRequest req = RestContext.request;
String strReqBody = req.requestBody.toString();
Map < String, Object > mapRequestBody = new Map < String, Object >();
mapRequestBody = ( Map < String, Object > )JSON.deserializeUntyped( strReqBody );
String strAccountName = mapRequestBody.containsKey( 'accountName' ) ?
mapRequestBody.get( 'accountName' ).toString() :
null;
System.debug(
'Account Name is ' +
strAccountName
);
if ( String.isNotBlank( strAccountName ) ) {
Account objAccount = new Account(
Name = strAccountName
);
insert objAccount;
mapResponse.put(
'accountId',
objAccount.Id
);
} else {
mapResponse.put(
'errorMessage',
'accountName is missing in the Request Body'
);
}
strResponse = JSON.serialize(
mapResponse
);
RestContext.response.addHeader(
'Content-Type',
'application/json'
);
RestContext.response.responseBody = Blob.valueOf(
strResponse
);
}
}
Output:
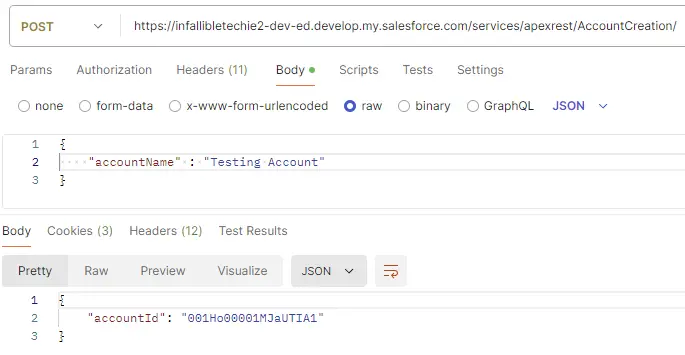