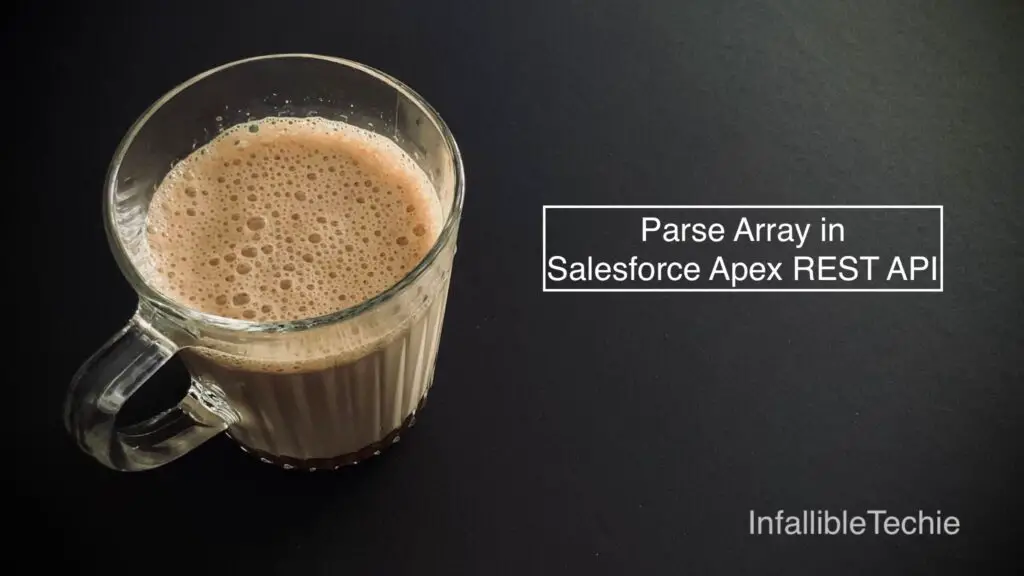
JSON.deserialize() can be used in Salesforce Apex to parse Array payload in Apex REST API.
Please check the following Apex Class for reference.
Sample Apex Class:
@RestResource( urlMapping='/ApexRESTAPI/' )
global with sharing class RESTAPIController {
@HttpPost
global static void checkArrayInput() {
String strResponse;
Map < String, String > mapResponse = new Map < String, String >();
RestRequest req = RestContext.request;
String strReqBody = req.requestBody.toString();
System.debug(
JSON.deserialize(
strReqBody,
List < String >.class
)
);
List < String > listInputs = ( List < String > ) JSON.deserialize(
strReqBody,
List < String >.class
);
if ( listInputs.size() > 0 ) {
mapResponse.put(
'message',
'Received ' + listInputs.size() + ' items'
);
} else {
mapResponse.put(
'errorMessage',
'Request Body is empty'
);
}
strResponse = JSON.serialize(
mapResponse
);
RestContext.response.addHeader(
'Content-Type',
'application/json'
);
RestContext.response.responseBody = Blob.valueOf(
strResponse
);
}
}
Output:
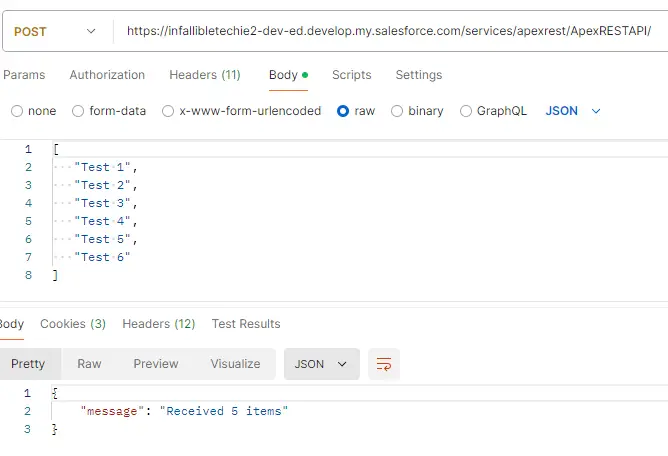