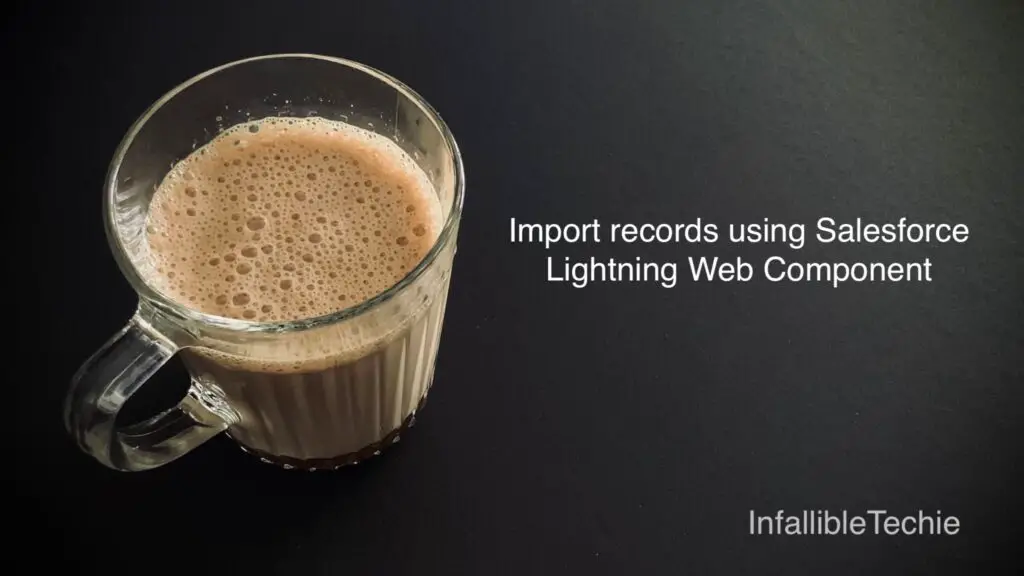
To import/load/upload records using Salesforce Lightning Web Component, lightning-file-upload tag can be used to allow users to upload the file and Apex can be use to insert the records.
Sample Code:
Apex Class:
public with sharing class ContactUploadFromAccountController {
@AuraEnabled
public static Map < String, Object > readFileData(
Id contentDocumentId
) {
String strMessage;
List < Contact > contactList = new List < Contact >();
Map < String, Object > mapResponse = new Map < String, Object >();
if ( contentDocumentId != null ) {
ContentVersion contentVersionObj = [
SELECT Id, VersionData
FROM ContentVersion
WHERE ContentDocumentId =:contentDocumentId
];
List < String > contactRecords = contentVersionObj.VersionData.toString().split( '\n' );
System.debug(
'contactRecords are ' +
contactRecords
);
//Removing the Header
contactRecords.remove( 0 );
for ( String contactRecord : contactRecords ) {
System.debug(
'contactRecord is ' +
contactRecord
);
if ( String.isNotBlank( contactRecord ) ) {
Contact objContact = new Contact();
List < String > contactInfo = contactRecord.split( ',' );
objContact.FirstName = contactInfo[ 0 ];
objContact.LastName = contactInfo[ 1 ];
objContact.Email = contactInfo[ 2 ];
contactList.add( objContact );
}
}
Database.DeleteResult deleteResult = Database.delete(
contentDocumentId,
true
);
mapResponse.put(
'Contacts',
contactList
);
mapResponse.put(
'message',
'File read successfully!!!'
);
}
return mapResponse;
}
@AuraEnabled
public static Map < String, Object > loadFileData(
Id strAccountId,
String contactListObjects
) {
List < Contact > contactList = new List < Contact >();
Map < String, Object > mapResponse = new Map < String, Object >();
try {
System.debug(
'contactListObjects is ' +
contactListObjects
);
contactList = ( List < Contact > ) JSON.deserialize(
contactListObjects,
List < Contact >.class
);
for ( Contact objContact : contactList ) {
objContact.AccountId = strAccountId;
}
if ( contactList.size() > 0 ) {
insert contactList;
mapResponse.put(
'message',
'Contacts loaded successfully'
);
} else {
mapResponse.put(
'message',
'Please check the Contacts before uploading'
);
}
} catch ( Exception e ) {
mapResponse.put(
'message',
'Some error occured. Please reach out to your System Admin'
);
system.debug(
'Exception is ' +
e.getMessage()
);
}
return mapResponse;
}
}
Lightning Web Component:
HTML:
<template>
<div class="slds-p-around_medium">
<lightning-card
variant="Narrow"
icon-name="standard:file"
title="Upload Contacts">
<template lwc:if={isLoaded}>
<lightning-spinner
alternative-text="Loading"
size="large">
</lightning-spinner>
</template>
<template lwc:if={showFileUpload}>
<div class="slds-var-p-horizontal_small">
<lightning-file-upload
accept={acceptedFormats}
label="Attach CSV File"
onuploadfinished={uploadFileHandler}>
</lightning-file-upload>
</div>
</template>
<template lwc:else>
<lightning-card>
<lightning-datatable
key-field="Email"
data={contactList}
columns={contactColumns}
hide-checkbox-column
show-row-number-column>
</lightning-datatable>
<p slot="footer">
<lightning-button
label="Create Contacts"
onclick={loadContacts}>
</lightning-button>
</p>
</lightning-card>
</template>
</lightning-card>
</div>
</template>
JavaScript:
import { LightningElement, api } from 'lwc';
import {ShowToastEvent} from 'lightning/platformShowToastEvent';
import readFileData from '@salesforce/apex/ContactUploadFromAccountController.readFileData';
import loadFileData from '@salesforce/apex/ContactUploadFromAccountController.loadFileData';
import { CloseActionScreenEvent } from 'lightning/actions';
export default class ContactUploadFromAccount extends LightningElement {
error;
isLoaded = false;
showFileUpload = true;
contactList;
@api recordId;
strContentDocumentId;
contactColumns = [
{ label: 'First Name', fieldName: 'FirstName' },
{ label: 'Last Name', fieldName: 'LastName' },
{ label: 'Email', fieldName: 'Email' }
];
get acceptedFormats() {
return ['.csv'];
}
uploadFileHandler( event ) {
this.isLoaded = true;
const uploadedFiles = event.detail.files;
this.strContentDocumentId = uploadedFiles[0].documentId;
readFileData( { contentDocumentId : this.strContentDocumentId } )
.then( result => {
this.isLoaded = false;
window.console.log(
'result',
JSON.stringify( result )
);
let strMessage = result.message;
this.contactList = result.Contacts;
this.showFileUpload = false;
this.dispatchEvent(
new ShowToastEvent( {
title: strMessage.includes("success") ? 'Success' : 'Error',
message: strMessage,
variant: strMessage.includes("success") ? 'success' : 'error',
mode: 'dismissible'
} )
);
})
.catch( error => {
this.isLoaded = false;
this.error = error;
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error!!',
message: JSON.stringify( error ),
variant: 'error',
mode: 'sticky'
} )
);
} )
}
loadContacts() {
this.isLoaded = true;
loadFileData( {
strAccountId : this.recordId,
contactListObjects : JSON.stringify( this.contactList )
} )
.then( result => {
window.console.log(
'result',
JSON.stringify( result )
);
let strMessage = result.message;
if ( strMessage.includes( "success" ) ) {
this.dispatchEvent(
new CloseActionScreenEvent()
);
this.dispatchEvent(
new ShowToastEvent( {
title: 'Success',
message: strMessage,
variant: 'success',
mode: 'dismissible'
} )
);
} else {
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error',
message: strMessage,
variant: 'error',
mode: 'dismissible'
} )
);
}
} )
.catch( error => {
this.dispatchEvent(
new ShowToastEvent( {
title: 'Error!!',
message: JSON.stringify( error ),
variant: 'error',
mode: 'sticky'
} )
);
} )
this.isLoaded = false;
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>61.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>ScreenAction</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>