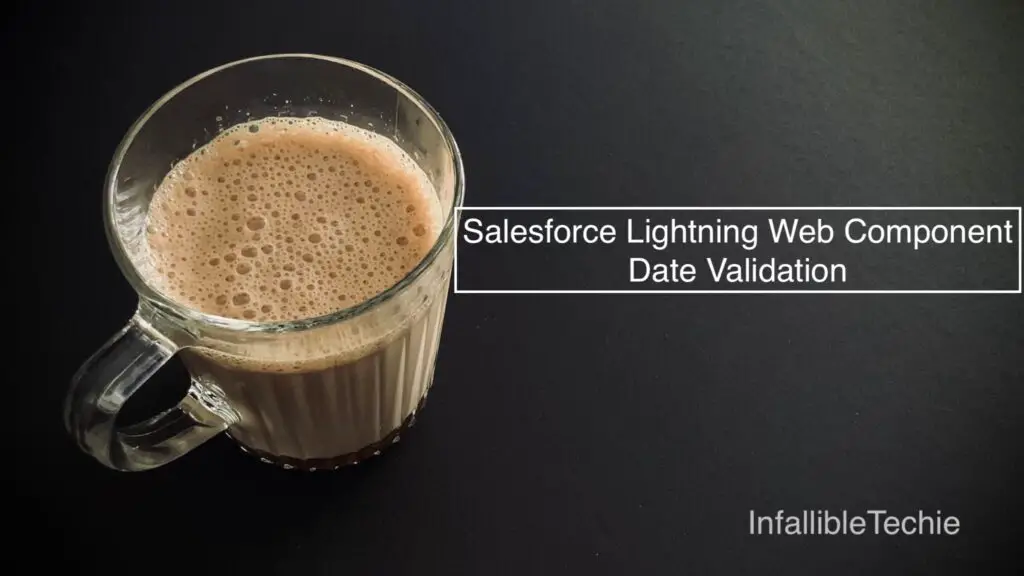
Sample Lightning Web Component:
HTML:
<template>
<lightning-card title="Date Validation">
<lightning-layout vertical-align="center">
<lightning-layout-item padding="around-small">
<lightning-input
type="Date"
label="Start Date"
value={startDate}
onchange={handleChange}
class="slds-p-around_medium">
</lightning-input>
</lightning-layout-item>
<lightning-layout-item padding="around-small">
<lightning-input
type="Date"
label="End Date"
value={endDate}
onchange={handleChange}
class="slds-p-around_medium">
</lightning-input>
</lightning-layout-item>
</lightning-layout>
</lightning-card>
</template>
JavaScript:
import { LightningElement } from 'lwc';
import LightningAlert from 'lightning/alert';
export default class SampleLightningWebComponent extends LightningElement {
endDate;
startDate;
handleChange(event) {
if ( event.target.label == 'Start Date' ) {
let selectedStartDate = event.target.value;
if ( selectedStartDate ) {
selectedStartDate = new Date( event.target.value );
let timeOffset = selectedStartDate.getTimezoneOffset();
selectedStartDate = new Date( selectedStartDate.getTime() + ( timeOffset * 60000 ) );
let dayValue = selectedStartDate.getDay();
console.log('dayValue is', dayValue);
if ( dayValue === 1 ) {
console.log('Inside Monday');
this.startDate = event.target.value;
} else {
LightningAlert.open( {
message: 'Start Date should be Monday',
theme: 'error',
label: 'Error!'
} );
this.startDate = '';
this.endDate = '';
setTimeout( () => {
this.startDate = null;
this.endDate = null;
}, 0);
}
} else {
this.startDate = '';
this.endDate = '';
setTimeout( () => {
this.startDate = null;
this.endDate = null;
}, 0 );
}
} else if ( event.target.label == 'End Date' ) {
if ( this.startDate == null ) {
LightningAlert.open( {
message: 'Start Date should be selected first',
theme: 'error',
label: 'Error!'
} );
this.endDate = '';
setTimeout( () => {
this.endDate = null;
}, 0);
} else {
this.endDate = event.target.value;
if ( this.startDate > this.endDate ) {
LightningAlert.open( {
message: 'End Date should be greater than Start Date',
theme: 'error',
label: 'Error!'
} );
this.endDate = '';
setTimeout( () => {
this.endDate = null;
}, 0 );
} else {
let dateDiff = parseInt(
( new Date( this.endDate ) - new Date( this.startDate ) )
/ (1000 * 60 * 60 * 24), 10
);
console.log(
'dateDiff is',
dateDiff
);
if (
dateDiff <=11 && dateDiff % 11 != 0 ||
( dateDiff - 11 ) % 14 != 0
) {
console.log(
'Date Diff divided by 14 remainder is',
dateDiff % 14
);
LightningAlert.open( {
message: 'End Date should be Friday and differencece should be in multiple of two weeks apart',
theme: 'error',
label: 'Error!'
} );
this.endDate = '';
setTimeout( () => {
this.endDate = null;
}, 0 );
}
}
}
}
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>62.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>