In the Embedded Service Deployment for Messaging for In-App Web, we can add the Logo in the Branding section.
I have used Asset File in Option 1. Check step 1 in the following link for your reference regarding Asset File.
Option 1:
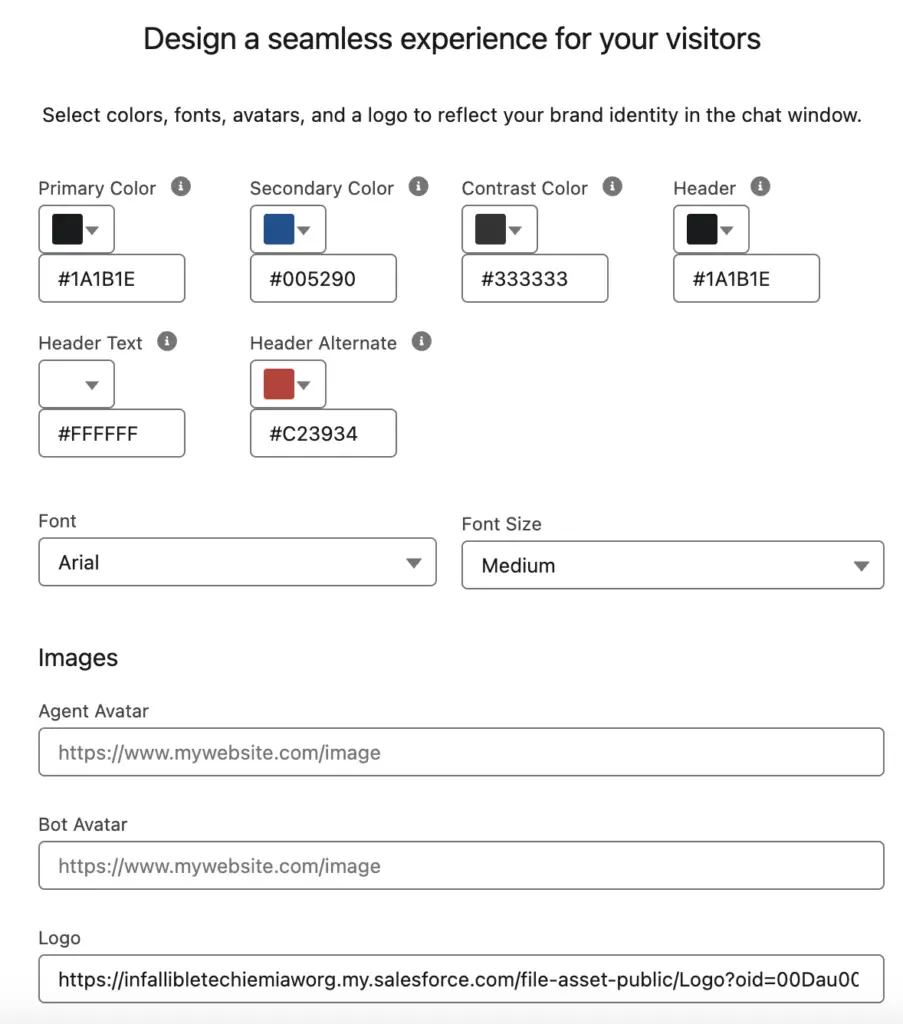
Option 2:
Using Salesforce Lightning Web Component, we can add logo to the Messaging for In-App and Web header. Use lightningSnapin__MessagingHeader as the target to set the Salesforce Lightning Web Component as the header in the Salesforce Embedded Service Deployment configuration for the Messaging for In-App and Web.
Sample Lightning Web Component for Header:
HTML:
<template>
<section class={menuClass}>
<template if:true={showMenuButton}>
<button
class="headerButton menuButton"
onclick={onMenuButtonClick}>
<lightning-icon
icon-name="utility:threedots_vertical"
variant="inverse"
size="x-small">
</lightning-icon>
</button>
</template>
<section class="optionsMenu slds-dropdown slds-dropdown_left slds-nubbin_top-left">
<ul class="slds-dropdown__list">
<template
for:each={chatbotOptionsMenu}
for:item="option">
<li
class="slds-dropdown__item"
key={option.optionIdentifier}>
<button class="chatbotMenuOption"
value={option.optionIdentifier}
onclick={onMenuOptionClick}>
<span value={option.optionIdentifier}>
{option.title}
</span>
</button>
</li>
</template>
<li class="slds-dropdown__item">
<button class="chatbotMenuOption"
onclick={onEndConversationClick}>
<span class="slds-text-color_error">End Conversation</span>
</button>
</li>
</ul>
</section>
</section>
<img
src="https://infallibletechiemiaworg--c.vf.force.com/resource/1730748259000/Logo"
alt="Logo">
</img>
<h2>Chat</h2>
<button
class="headerButton minimizeButton"
onclick={onMinimizeButtonClick}>
<lightning-icon
icon-name="utility:chevrondown"
variant="inverse"
size="x-small">
</lightning-icon>
</button>
<template if:true={showCloseButton}>
<button
class="headerButton closeButton"
onclick={onCloseButtonClick}>
<lightning-icon
icon-name="utility:close"
variant="inverse"
size="x-small">
</lightning-icon>
</button>
</template>
</template>
JavaScript:
import { LightningElement, api } from "lwc";
import {
dispatchMessagingEvent,
assignMessagingEventHandler,
MESSAGING_EVENT
} from "lightningsnapin/eventStore";
const SLDS_MENU_SELECTOR = "slds-dropdown-trigger slds-dropdown-trigger_click";
export default class MessagingHeader extends LightningElement {
@api configuration = {};
@api conversationStatus;
menuClass = SLDS_MENU_SELECTOR;
chatbotOptionsMenu = [];
get showMenuButton() {
return this.conversationStatus === "OPEN";
}
_showMenu = false;
get showMenu() {
return this._showMenu;
}
set showMenu(shouldShow) {
this.menuClass = SLDS_MENU_SELECTOR + (shouldShow ? " slds-is-open" : "");
this._showMenu = shouldShow;
}
get showCloseButton() {
return this.conversationStatus !== "OPEN";
}
onMenuButtonClick() {
this.showMenu = !this.showMenu;
}
onMinimizeButtonClick() {
dispatchMessagingEvent(MESSAGING_EVENT.MINIMIZE_BUTTON_CLICK, {});
}
onCloseButtonClick() {
dispatchMessagingEvent(MESSAGING_EVENT.CLOSE_CONTAINER, {});
}
onMenuOptionClick(event) {
event.preventDefault();
dispatchMessagingEvent( MESSAGING_EVENT.MENU_ITEM_SELECTED, {
selectedOption: this.chatbotOptionsMenu.find(
option => option.optionIdentifier === event.target.getAttribute( "value" )
)
});
this.showMenu = false;
}
onEndConversationClick() {
dispatchMessagingEvent( MESSAGING_EVENT.CLOSE_CONVERSATION, {} );
this.showMenu = false;
}
connectedCallback() {
assignMessagingEventHandler(MESSAGING_EVENT.PARTICIPANT_JOINED, ( data ) => {
console.log(
'Participant joined'
);
if ( data.options && Array.isArray( data.options ) ) {
data.options.forEach( ( participantOption ) => {
this.chatbotOptionsMenu.push( participantOption );
} );
}
} );
assignMessagingEventHandler( MESSAGING_EVENT.PARTICIPANT_LEFT, ( data ) => {
console.log(
'Participant left',
JSON.stringify( data )
);
this.chatbotOptionsMenu = [];
});
assignMessagingEventHandler( MESSAGING_EVENT.UPDATE_HEADER_TEXT, ( data ) => {
console.log(`Update header text: ${data.text}`);
} );
assignMessagingEventHandler(MESSAGING_EVENT.TOGGLE_BACK_BUTTON, ( data ) => {
console.log(`Toggle back button visibility.`);
this.showBackButton = data.showBackButton;
} );
}
}
CSS:
:host {
width: 100%;
max-width: 100%;
min-height: 50px;
display: flex;
align-items: center;
box-sizing: border-box;
position: relative;
padding: 0 14px;
height: 50px;
max-height: 50px;
background-color: black;
}
img {
margin-right: 4px;
max-height: 40px;
max-width: 40px;
}
h2 {
margin: 0;
text-align: initial;
align-self: center;
flex-grow: 1;
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
font-weight: lighter;
font-size: inherit;
color: white;
}
.headerButton {
background: none;
border: none;
display: inline-flex;
height: 32px;
min-height: 32px;
width: 32px;
min-width: 32px;
align-items: center;
justify-content: center;
}
.headerButton:hover:before {
content: " ";
position: absolute;
top: 9px;
width: 32px;
height: 32px;
background-color: #fff;
opacity: .2;
border-radius: 4px;
box-sizing: border-box;
pointer-events: none;
}
.headerButton.menuButton:hover:before {
top: 0;
}
.headerButton.closeButton:hover:before {
right: 14px;
}
lightning-icon {
fill: white;
}
.optionsMenu {
width: 100vw;
left: -0.9rem;
}
.optionsMenu > .slds-dropdown__list {
max-height: 20rem;
overflow-y: auto;
}
.slds-nubbin_top-left:before,
.slds-nubbin_top-left:after {
left: 2rem;
top: -0.5rem;
}
.slds-dropdown {
max-width: 30rem;
}
.slds-dropdown__item > button {
position: relative;
z-index: 100;
padding: 0.75rem 1rem;
background-color: #ffffff;
border: 0;
display: flex;
width: 100%;
}
.slds-dropdown__item > button:focus,
.slds-dropdown__item > button:hover {
outline: 0;
text-decoration: none;
background-color: #e5e5e5;
}
.slds-dropdown__list > .slds-dropdown__item:not(:first-child) {
border-top: #e2e2e2 1px solid;
}
.slds-dropdown__item .slds-truncate:not(.closeConversation) {
color: #005290;
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>62.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightningSnapin__MessagingHeader</target>
</targets>
</LightningComponentBundle>
Use the Lightning Web Component as the Header in the Custom UI Components of the Embedded Service Deployment.
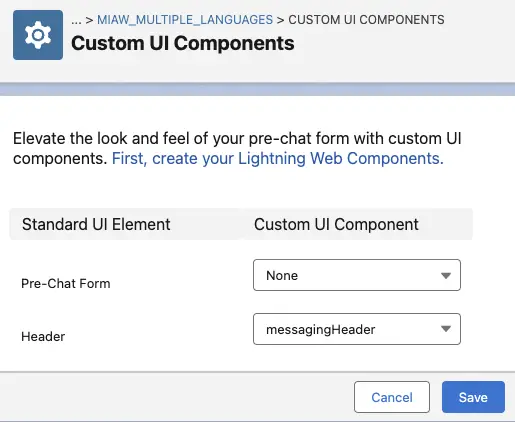
Output:
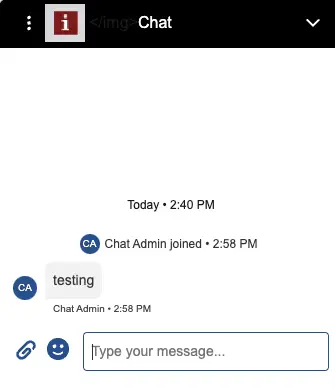