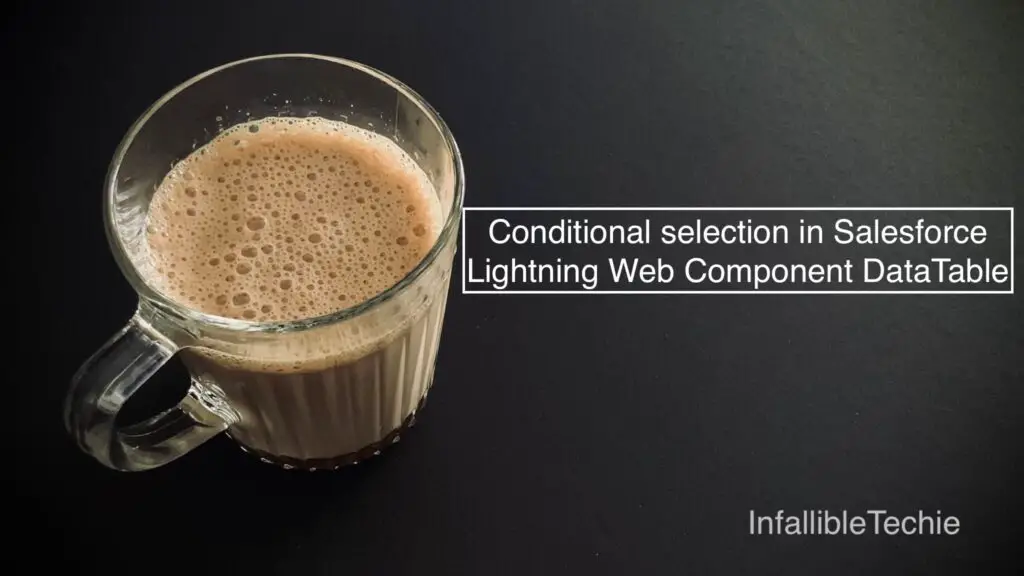
We cannot hide and show the check box in the lightning-datable for the rows. So, for conditional selection, we can validate the selected rows and throw an error if the selected row is not intended to be selected. Using this approach, we can users avoid selecting specific rows in the Salesforce Lightning Web Component lightning-datatable.
Sample Apex Class:
public class SampleLightningWebComponentController {
@AuraEnabled( cacheable = true )
public static List< Account > fetchAccounts() {
return [
SELECT Id, Name, Industry, Type
FROM Account
LIMIT 10
];
}
}
Sample Lightning Web Component:
HMTL:
<template>
<lightning-card
title="Accounts"
icon-name="standard:account">
<template if:true={accounts}>
<lightning-datatable
key-field="Id"
data={accounts}
columns={columns}
onrowselection={handleSelected}
selected-rows={selectedAccountIds}>
</lightning-datatable>
</template>
<template if:true={error}>
{error}>
</template>
</lightning-card>
</template>
JavaScript:
import { LightningElement, wire } from 'lwc';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import fetchAccounts from '@salesforce/apex/SampleLightningWebComponentController.fetchAccounts';
const COLUMNS = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Industry', fieldName: 'Industry' },
{ label: 'Type', fieldName: 'Type' }
];
export default class SampleLightningWebComponent extends LightningElement {
accounts;
error;
selectedAccounts;
columns = COLUMNS;
selectedAccountIds = [];
@wire( fetchAccounts )
wiredAccount( { error, data } ) {
if ( data ) {
this.accounts = data;
this.error = undefined;
} else if ( error ) {
this.error = error;
this.accounts = undefined;
}
}
handleSelected( event ) {
console.log(
'Selected Rows are',
JSON.stringify(
event.detail.selectedRows
)
);
this.selectedAccountIds = event.detail.selectedRows.map( row => {
let tempAccountId;
if(row.Type !== 'Prospect') {
tempAccountId = row.Id;
} else {
this.dispatchEvent(
new ShowToastEvent( {
variant: 'error',
title: 'Account not Available',
message: 'Please select Non-Prospect Accounts only'
} )
);
}
return tempAccountId;
} );
this.selectedAccounts = event.detail.selectedRows.filter(
row => row.Type !== 'Prospect'
);
console.log(
'Selected Accounts are',
JSON.stringify(
this.selectedAccounts
)
);
}
}
js-meta.xml:
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>62.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
Output:
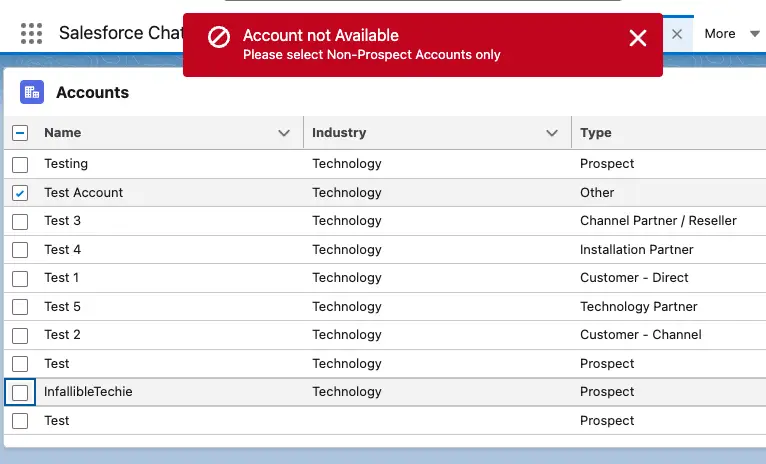